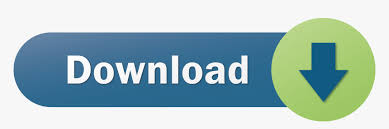
Periodic Table 1.1 serial key or number

Periodic Table 1.1 serial key or number
Caution: The documentation you are viewing is for an older version of Zend Framework.
You can find the documentation of the current version at:
https://docs.zendframework.com/
The Zend_Db_Table class is an object-oriented interface to database tables. It provides methods for many common operations on tables. The base class is extensible, so you can add custom logic.
The Zend_Db_Table solution is an implementation of the » Table Data Gateway pattern. The solution also includes a class that implements the » Row Data Gateway pattern.
Using Zend_Db_Table as a concrete class
As of Zend Framework 1.9, you can instantiate Zend_Db_Table. This added benefit is that you do not have to extend a base class and configure it to do simple operations such as selecting, inserting, updating and deleteing on a single table. below is an example of the simplest of use cases.
Example #1 Declaring a table class with just the string name
- Zend_Db_Table::setDefaultAdapter($dbAdapter);
- $bugTable = new Zend_Db_Table('bug');
The above example represents the simplest of use cases. Make not of all the options describe below for configuring Zend_Db_Table tables. If you want to be able to use the concrete usage case, in addition to the more complex relationhip features, see the Zend_Db_Table_Definition documentation.
For each table in your database that you want to access, define a class that extends Zend_Db_Table_Abstract.
Defining the Table Name and Schema
Declare the database table for which this class is defined, using the protected variable . This is a string, and must contain the name of the table spelled as it appears in the database.
Example #2 Declaring a table class with explicit table name
- class Bugs extends Zend_Db_Table_Abstract
- protected $_name = 'bugs';
If you don't specify the table name, it defaults to the name of the class. If you rely on this default, the class name must match the spelling of the table name as it appears in the database.
Example #3 Declaring a table class with implicit table name
- class bugs extends Zend_Db_Table_Abstract
- // table name matches class name
You can also declare the schema for the table, either with the protected variable , or with the schema prepended to the table name in the property. Any schema specified with the property takes precedence over a schema specified with the property. In some brands, the term for schema is "database" or "tablespace," but it is used similarly.
Example #4 Declaring a table class with schema
- class Bugs extends Zend_Db_Table_Abstract
- protected $_schema = 'bug_db';
- protected $_name = 'bugs';
- class Bugs extends Zend_Db_Table_Abstract
- protected $_name = 'bug_db.bugs';
- // If schemas are specified in both $_name and $_schema, the one
- // specified in $_name takes precedence:
- class Bugs extends Zend_Db_Table_Abstract
- protected $_name = 'bug_db.bugs';
- protected $_schema = 'ignored';
The schema and table names may also be specified via constructor configuration directives, which override any default values specified with the and properties. A schema specification given with the name directive overrides any value provided with the schema option.
Example #5 Declaring table and schema names upon instantiation
- class Bugs extends Zend_Db_Table_Abstract
- $tableBugs = new Bugs(array('name' => 'bugs', 'schema' => 'bug_db'));
- $tableBugs = new Bugs(array('name' => 'bug_db.bugs'));
- // If schemas are specified in both 'name' and 'schema', the one
- // specified in 'name' takes precedence:
- $tableBugs = new Bugs(array('name' => 'bug_db.bugs',
- 'schema' => 'ignored'));
If you don't specify the schema name, it defaults to the schema to which your database adapter instance is connected.
Defining the Table Primary Key
Every table must have a primary key. You can declare the column for the primary key using the protected variable . This is either a string that names the single column for the primary key, or else it is an array of column names if your primary key is a compound key.
Example #6 Example of specifying the primary key
- class Bugs extends Zend_Db_Table_Abstract
- protected $_name = 'bugs';
- protected $_primary = 'bug_id';
If you don't specify the primary key, Zend_Db_Table_Abstract tries to discover the primary key based on the information provided by the describeTable()´ method.
Note: Every table class must know which columns can be used to address rows uniquely. If no primary key columns are specified in the table class definition or the table constructor arguments, or discovered in the table metadata provided by describeTable(), then the table cannot be used with Zend_Db_Table.
Overriding Table Setup Methods
When you create an instance of a Table class, the constructor calls a set of protected methods that initialize metadata for the table. You can extend any of these methods to define metadata explicitly. Remember to call the method of the same name in the parent class at the end of your method.
Example #7 Example of overriding the _setupTableName() method
- class Bugs extends Zend_Db_Table_Abstract
- protected function _setupTableName()
- ->_name = 'bugs';
- parent::_setupTableName();
The setup methods you can override are the following:
_setupDatabaseAdapter() checks that an adapter has been provided; gets a default adapter from the registry if needed. By overriding this method, you can set a database adapter from some other source.
_setupTableName() defaults the table name to the name of the class. By overriding this method, you can set the table name before this default behavior runs.
_setupMetadata() sets the schema if the table name contains the pattern "schema.table"; calls describeTable() to get metadata information; defaults the array to the columns reported by describeTable(). By overriding this method, you can specify the columns.
_setupPrimaryKey() defaults the primary key columns to those reported by describeTable(); checks that the primary key columns are included in the array. By overriding this method, you can specify the primary key columns.
If application-specific logic needs to be initialized when a Table class is constructed, you can select to move your tasks to the init() method, which is called after all Table metadata has been processed. This is recommended over the __construct() method if you do not need to alter the metadata in any programmatic way.
Example #8 Example usage of init() method
- class Bugs extends Zend_Db_Table_Abstract
- ->_observer = new MyObserverClass();
Creating an Instance of a Table
Before you use a Table class, create an instance using its constructor. The constructor's argument is an array of options. The most important option to a Table constructor is the database adapter instance, representing a live connection to an . There are three ways of specifying the database adapter to a Table class, and these three ways are described below:
Specifying a Database Adapter
The first way to provide a database adapter to a Table class is by passing it as an object of type Zend_Db_Adapter_Abstract in the options array, identified by the key 'db'.
Example #9 Example of constructing a Table using an Adapter object
- $db = Zend_Db::factory('PDO_MYSQL', $options);
- $table = new Bugs(array('db' => $db));
Setting a Default Database Adapter
The second way to provide a database adapter to a Table class is by declaring an object of type Zend_Db_Adapter_Abstract to be a default database adapter for all subsequent instances of Tables in your application. You can do this with the static method Zend_Db_Table_Abstract::setDefaultAdapter(). The argument is an object of type Zend_Db_Adapter_Abstract.
Example #10 Example of constructing a Table using a the Default Adapter
- $db = Zend_Db::factory('PDO_MYSQL', $options);
- Zend_Db_Table_Abstract::setDefaultAdapter($db);
It can be convenient to create the database adapter object in a central place of your application, such as the bootstrap, and then store it as the default adapter. This gives you a means to ensure that the adapter instance is the same throughout your application. However, setting a default adapter is limited to a single adapter instance.
Storing a Database Adapter in the Registry
The third way to provide a database adapter to a Table class is by passing a string in the options array, also identified by the 'db' key. The string is used as a key to the static Zend_Registry instance, where the entry at that key is an object of type Zend_Db_Adapter_Abstract.
Example #11 Example of constructing a Table using a Registry key
- $db = Zend_Db::factory('PDO_MYSQL', $options);
- Zend_Registry::set('my_db', $db);
- $table = new Bugs(array('db' => 'my_db'));
Like setting the default adapter, this gives you the means to ensure that the same adapter instance is used throughout your application. Using the registry is more flexible, because you can store more than one adapter instance. A given adapter instance is specific to a certain brand and database instance. If your application needs access to multiple databases or even multiple database brands, then you need to use multiple adapters.
Inserting Rows to a Table
You can use the Table object to insert rows into the database table on which the Table object is based. Use the insert() method of your Table object. The argument is an associative array, mapping column names to values.
Example #12 Example of inserting to a Table
- 'created_on' => '2007-03-22',
- 'bug_description' => 'Something wrong',
- 'bug_status' => 'NEW'
- $table->insert($data);
By default, the values in your data array are inserted as literal values, using parameters. If you need them to be treated as expressions, you must make sure they are distinct from plain strings. Use an object of type Zend_Db_Expr to do this.
Example #13 Example of inserting expressions to a Table
- 'created_on' => new Zend_Db_Expr('CURDATE()'),
- 'bug_description' => 'Something wrong',
- 'bug_status' => 'NEW'
In the examples of inserting rows above, it is assumed that the table has an auto-incrementing primary key. This is the default behavior of Zend_Db_Table_Abstract, but there are other types of primary keys as well. The following sections describe how to support different types of primary keys.
Using a Table with an Auto-incrementing Key
An auto-incrementing primary key generates a unique integer value for you if you omit the primary key column from your statement.
In Zend_Db_Table_Abstract, if you define the protected variable to be the Boolean value , then the class assumes that the table has an auto-incrementing primary key.
Example #14 Example of declaring a Table with auto-incrementing primary key
- class Bugs extends Zend_Db_Table_Abstract
- protected $_name = 'bugs';
- // This is the default in the Zend_Db_Table_Abstract class;
- // you do not need to define this.
- protected $_sequence = true;
MySQL, Microsoft Server, and SQLite are examples of brands that support auto-incrementing primary keys.
PostgreSQL has a notation that implicitly defines a sequence based on the table and column name, and uses the sequence to generate key values for new rows. has an notation that works similarly. If you use either of these notations, treat your Zend_Db_Table class as having an auto-incrementing column with respect to declaring the member as .
Using a Table with a Sequence
A sequence is a database object that generates a unique value, which can be used as a primary key value in one or more tables of the database.
If you define to be a string, then Zend_Db_Table_Abstract assumes the string to name a sequence object in the database. The sequence is invoked to generate a new value, and this value is used in the operation.
Example #15 Example of declaring a Table with a sequence
- class Bugs extends Zend_Db_Table_Abstract
- protected $_name = 'bugs';
- protected $_sequence = 'bug_sequence';
Oracle, PostgreSQL, and are examples of brands that support sequence objects in the database.
PostgreSQL and also have syntax that defines sequences implicitly and associated with columns. If you use this notation, treat the table as having an auto-incrementing key column. Define the sequence name as a string only in cases where you would invoke the sequence explicitly to get the next key value.
Using a Table with a Natural Key
Some tables have a natural key. This means that the key is not automatically generated by the table or by a sequence. You must specify the value for the primary key in this case.
If you define the to be the Boolean value , then Zend_Db_Table_Abstract assumes that the table has a natural primary key. You must provide values for the primary key columns in the array of data to the insert() method, or else this method throws a Zend_Db_Table_Exception.
Example #16 Example of declaring a Table with a natural key
- class BugStatus extends Zend_Db_Table_Abstract
- protected $_name = 'bug_status';
- protected $_sequence = false;
Note: All brands support tables with natural keys. Examples of tables that are often declared as having natural keys are lookup tables, intersection tables in many-to-many relationships, or most tables with compound primary keys.
You can update rows in a database table using the update() method of a Table class. This method takes two arguments: an associative array of columns to change and new values to assign to these columns; and an expression that is used in a clause, as criteria for the rows to change in the operation.
Example #17 Example of updating rows in a Table
- 'updated_on' => '2007-03-23',
- 'bug_status' => 'FIXED'
- $where = $table->getAdapter()->quoteInto('bug_id = ?', 1234);
- $table->update($data, $where);
Since the table update() method proxies to the database adapter update() method, the second argument can be an array of expressions. The expressions are combined as Boolean terms using an operator.
Note: The values and identifiers in the expression are not quoted for you. If you have values or identifiers that require quoting, you are responsible for doing this. Use the quote(), quoteInto(), and quoteIdentifier() methods of the database adapter.
Deleting Rows from a Table
You can delete rows from a database table using the delete() method. This method takes one argument, which is an expression that is used in a clause, as criteria for the rows to delete.
Example #18 Example of deleting rows from a Table
- $where = $table->getAdapter()->quoteInto('bug_id = ?', 1235);
- $table->delete($where);
Since the table delete() method proxies to the database adapter delete() method, the argument can also be an array of expressions. The expressions are combined as Boolean terms using an operator.
Note: The values and identifiers in the expression are not quoted for you. If you have values or identifiers that require quoting, you are responsible for doing this. Use the quote(), quoteInto(), and quoteIdentifier() methods of the database adapter.
Finding Rows by Primary Key
You can query the database table for rows matching specific values in the primary key, using the find() method. The first argument of this method is either a single value or an array of values to match against the primary key of the table.
Example #19 Example of finding rows by primary key values
- $rows = $table->find(1234);
- $rows = $table->find(array(1234, 5678));
If you specify a single value, the method returns at most one row, because a primary key cannot have duplicate values and there is at most one row in the database table matching the value you specify. If you specify multiple values in an array, the method returns at most as many rows as the number of distinct values you specify.
The find() method might return fewer rows than the number of values you specify for the primary key, if some of the values don't match any rows in the database table. The method even may return zero rows. Because the number of rows returned is variable, the find() method returns an object of type Zend_Db_Table_Rowset_Abstract.
If the primary key is a compound key, that is, it consists of multiple columns, you can specify the additional columns as additional arguments to the find() method. You must provide as many arguments as the number of columns in the table's primary key.
To find multiple rows from a table with a compound primary key, provide an array for each of the arguments. All of these arrays must have the same number of elements. The values in each array are formed into tuples in order; for example, the first element in all the array arguments define the first compound primary key value, then the second elements of all the arrays define the second compound primary key value, and so on.
Example #20 Example of finding rows by compound primary key values
The call to find() below to match multiple rows can match two rows in the database. The first row must have primary key value (1234, 'ABC'), and the second row must have primary key value (5678, 'DEF').
- class BugsProducts extends Zend_Db_Table_Abstract
- protected $_name = 'bugs_products';
- protected $_primary = array('bug_id', 'product_id');
- $table = new BugsProducts();
- // Find a single row with a compound primary key
- $rows = $table->find(1234, 'ABC');
- // Find multiple rows with compound primary keys
- $rows = $table->find(array(1234, 5678), array('ABC', 'DEF'));
Querying for a Set of Rows
The for fetch operations has been superseded to allow a Zend_Db_Table_Select object to modify the query. However, the deprecated usage of the fetchRow() and fetchAll() methods will continue to work without modification.
The following statements are all legal and functionally identical, however it is recommended to update your code to take advantage of the new usage where possible.
- $rows = $table->fetchAll(
- $rows = $table->fetchAll(
- ->where('bug_status = ?', 'NEW')
- $rows = $table->fetchAll(
- ->where('bug_status = :status')
- ->bind(array(':status'=>'NEW')
- $row = $table->fetchRow(
- $row = $table->fetchRow(
- ->where('bug_status = ?', 'NEW')
- $row = $table->fetchRow(
- ->where('bug_status = :status')
- ->bind(array(':status'=>'NEW')
The Zend_Db_Table_Select object is an extension of the Zend_Db_Select object that applies specific restrictions to a query. The enhancements and restrictions are:
World's largest periodic table at WA university adds to Australia's Big Things
A Perth university has decorated one wall of a new science building with what it believes is the world's largest periodic table of the elements.
Key points:
- The periodic table on the outside of a WA university building is 662 square metres
- The periodic table was created 150 years ago by Russian chemist Dmitri Mendeleev
- Vice-chancellor Professor Steve Chapman hopes it will join the list of Australia's Big Things
Australia latest Big Thing, at Edith Cowan University's Joondalup campus, was the brainchild of the institution's vice-chancellor Professor Steve Chapman.
"When you build a science building you have to fill it with a lot of high-tech stuff, with fume hoods and benches and gas lines and everything so you kind of build an oblong," Professor Chapman explained.
"So I said to the guys, 'Why don't we have the world's largest periodic table?' and they said, 'You're nuts' but then they looked into it and thought, 'We could do that'."
Professor radiates enthusiasm for periodic table
At 662 square metres, the table at the WA university is more than four times the size of the periodic table at Spain's University of Murcia which was created in 2017 and is 150 square metres.
This year also marks the 150th anniversary of the table's creation by Russian chemist Dmitri Mendeleev.
Professor Chapman, whose academic background is in biological chemistry and crystallography, was brimming with enthusiasm when he spoke about the periodic table of the elements.
"It's arguably, in my opinion, one of mankind's greatest scientific achievements.
"Everything we have in the universe — every known thing — is in the periodic table.
"Looking at the position you can work out whether it's a metal or a gas.
"It has so much information in it, it is like a kind of map of everything that exists on the planet."
As vice-chancellor, Professor Chapman spends more time in management meetings than in the laboratory but he plans to include the giant table in his outdoor lectures.
"The only real connection I get with chemistry is that I do some first-year lectures which I absolutely love.
"I do that just to remind myself why I'm in academia because being a vice-chancellor I'm in a lot of meetings and doing a lot of things to do with finance etcetera.
"But the real reason you do it is to let everybody else do the education, the science and their research.
"But reconnecting by indulging yourself by making the world's largest periodic table — that's kinda cool."
The next Big Thing?
Australia has more than 150 Big Things — oversized sculptures or creations — in each state and territory.
Professor Chapman hoped the periodic table would join the country's list of Big Things.
"This should be on the list of Australia's Big Things and in my humble opinion I think this is the most intellectual of Australia's Big Things.
"It's certainly more intellectual than a peach or a prawn — not that I have anything against prawns and peaches."
1.1 Atomic Structure: The Nucleus
- Last updated
- Save as PDF
- Page ID
- 67039
Objective
After completing this section, you should be able to describe the basic structure of the atom.
Key Terms
Make certain that you can define, and use in context, the key terms below.
- atomic number
- atomic weight
- electron
- mass number
- neutron
- proton
The nuclear atom
The precise physical nature of atoms finally emerged from a series of elegant experiments carried out between 1895 and 1915. The most notable of these achievements was Ernest Rutherford's famous 1911 alpha-ray scattering experiment, which established that
•
- Almost all of the mass of an atom is contained within a tiny (and therefore extremely dense) which carries a positive electric charge whose value identifies each element and is known as the of the element.
- Almost all of the volume of an atom consists of empty space in which electrons, the fundamental carriers of negative electric charge, reside. The extremely small mass of the electron (1/1840 the mass of the hydrogen nucleus) causes it to behave as a quantum particle, which means that its location at any moment cannot be specified; the best we can do is describe its behavior in terms of the probability of its manifesting itself at any point in space. It is common (but somewhat misleading) to describe the volume of space in which the electrons of an atom have a significant probability of being found as the electron cloud. The latter has no definite outer boundary, so neither does the atom. The radius of an atom must be defined arbitrarily, such as the boundary in which the electron can be found with 95% probability. Atomic radii are typically 30-300 pm.
Protons and neutrons
The nucleus is itself composed of two kinds of particles. are the carriers of positive electric charge in the nucleus; the proton charge is exactly the same as the electron charge, but of opposite sign. This means that in any [electrically neutral] atom, the number of protons in the nucleus (often referred to as the nuclear charge) is balanced by the same number of electrons outside the nucleus.
Because the electrons of an atom are in contact with the outside world, it is possible for one or more electrons to be lost, or some new ones to be added. The resulting electrically-charged atom is called an .
The other nuclear particle is the . As its name implies, this particle carries no electrical charge. Its mass is almost the same as that of the proton. Most nuclei contain roughly equal numbers of neutrons and protons, so we can say that these two particles together account for almost all the mass of the atom.
Atomic Number (Z)
What single parameter uniquely characterizes the atom of a given element? It is not the atom's relative mass, as we will see in the section on isotopes below. It is, rather, the number of protons in the nucleus, which we call the and denote by the symbol Z. Each proton carries an electric charge of +1, so the atomic number also specifies the electric charge of the nucleus. In the neutral atom, the Z protons within the nucleus are balanced by Zelectrons outside it.
Atomic numbers were first worked out in 1913 by Henry Moseley, a young member of Rutherford's research group in Manchester.
Moseley searched for a measurable property of each element that increases linearly with atomic number. He found this in a class of X-rays emitted by an element when it is bombarded with electrons. The frequencies of these X-rays are unique to each element, and they increase uniformly in successive elements. Moseley found that the square roots of these frequencies give a straight line when plotted against Z; this enabled him to sort the elements in order of increasing atomic number.
You can think of the atomic number as a kind of serial number of an element, commencing at 1 for hydrogen and increasing by one for each successive element. The chemical name of the element and its symbol are uniquely tied to the atomic number; thus the symbol "Sr" stands for strontium, whose atoms all have Z = 38.
Mass number (A)
This is just the sum of the numbers of protons and neutrons in the nucleus. It is sometimes represented by the symbol A, so
in which Z is the atomic number and N is the neutron number.
Nuclides and their Symbols
The term simply refers to any particular kind of nucleus. For example, a nucleus of atomic number 7 is a nuclide of nitrogen. Any nuclide is characterized by the pair of numbers (Z ,A). The element symbol depends on Z alone, so the symbol 26Mg is used to specify the mass-26 nuclide of manganese, whose name implies Z=12. A more explicit way of denoting a particular kind of nucleus is to add the atomic number as a subscript. Of course, this is somewhat redundant, since the symbol Mg always implies Z=12, but it is sometimes a convenience when discussing several nuclides.
Two nuclides having the same atomic number but different mass numbers are known as . Most elements occur in nature as mixtures of isotopes, but twenty-three of them (including beryllium and fluorine, shown in the table) are monoisotopic. For example, there are three natural isotopes of magnesium: 24Mg (79% of all Mg atoms), 25Mg (10%), and 26Mg (11%); all three are present in all compounds of magnesium in about these same proportions.
Approximately 290 isotopes occur in nature. The two heavy isotopes of hydrogen are especially important— so much so that they have names and symbols of their own:
Deuterium accounts for only about 15 out of every one million atoms of hydrogen. Tritium, which is radioactive, is even less abundant. All the tritium on the earth is a by-product of the decay of other radioactive elements.
Atomic weights
Atoms are of course far too small to be weighed directly; weight measurements can only be made on the massive (but unknown) numbers of atoms that are observed in chemical reactions. The early combining-weight experiments of Dalton and others established that hydrogen is the lightest of the atoms, but the crude nature of the measurements and uncertainties about the formulas of many compounds made it difficult to develop a reliable scale of the relative weights of atoms. Even the most exacting weight measurements we can make today are subject to experimental uncertainties that limit the precision to four significant figures at best.
The periodic table
The elements are arranged in a periodic table, which is probably the single most important learning aid in chemistry. It summarizes huge amounts of information about the elements in a way that facilitates the prediction of many of their properties and chemical reactions. The elements are arranged in seven horizontal rows, in order of increasing atomic number from left to right and top to bottom. The rows are called periods, and they are numbered from 1 to 7. The elements are stacked in such a way that elements with similar chemical properties form vertical columns, called groups, numbered from 1 to 18 (older periodic tables use a system based on roman numerals). Groups 1, 2, and 13–18 are the main group elements, listed as A in older tables. Groups 3–12 are in the middle of the periodic table and are the transition elements, listed as B in older tables. The two rows of 14 elements at the bottom of the periodic table are the lanthanides and the actinides, whose positions in the periodic table are indicated in group 3.
Contributors and Attributions
- Back to top
- There are no recommended articles.
- Article type
- Section or Page
- Show TOC
- no on page
- Tags
- This page has no tags.
What’s New in the Periodic Table 1.1 serial key or number?
Screen Shot
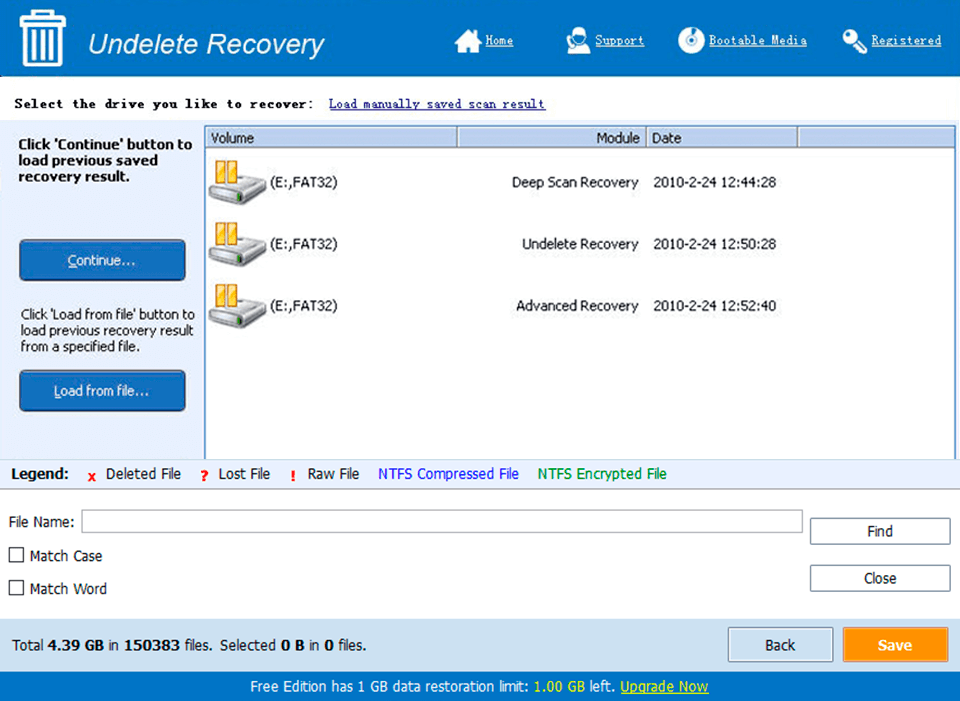
System Requirements for Periodic Table 1.1 serial key or number
- First, download the Periodic Table 1.1 serial key or number
-
You can download its setup from given links: