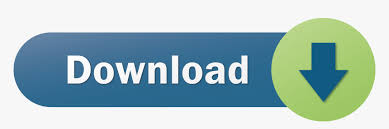
Auto Run Design 3.0.0.16 serial key or number

Auto Run Design 3.0.0.16 serial key or number
3.0 - JavaScript Functions and Methods
Overview
ProcessMaker 3 provides a number of custom JavaScript functions and methods for working with controls in Dynaforms. For more information about how to use custom code in forms, see JavaScript in Dynaforms. Note that these functions and methods are only available in the new responsive Dynaforms used in BPMN processes. If importing an old process from ProcessMaker 2, then use the old JavaScript functions from ProcessMaker 2 to manipulate the Dynaform.
Ways to Access Dynaform Controls
Responsive Dynaforms in ProcessMaker 3 and the libraries they are based upon (jQuery, Backbone and Bootstrap) provide custom methods to interact with Dynaform controls. To use a ProcessMaker method to manipulate a control, first obtain the object for the control, using either a jQuery selector or one of ProcessMaker's custom functions, such as getFieldById(), which are listed below. It is recommended to use ProcessMaker's custom methods to manipulate the controls when possible, because they have built-in error checking when setting the value of fields and are generally easier to use.
Programmers who are more comfortable using standard JavaScript rather than jQuery may wish to access controls using their Document Object Model (DOM) objects, which provide the standard methods. Take into account that these methods are not officially supported, and their use may cause issues when working on ProcessMaker Mobile.
Parts of a Control
ProcessMaker's custom methods refer to 3 parts of a control:
- The label is the text displayed to the left of or above the control to inform the user about the purpose of the control.
- The text is what is displayed to the user in an input field. For textboxes and textareas, the text is the same as the value, but other types of input fields, such as dropdown boxes, checkboxes and radio buttons, have a separate displayed text and a stored value (key) for each option.
- The value is what is entered in an input field and saved when the Dynaform is submitted. The value is the same as the text in fields such as textboxes and textareas, but it is different in fields that have selectable options, such as dropdown boxes, checkboxes and radio buttons, where the visible text is different from the internal value (key) that represents an option.
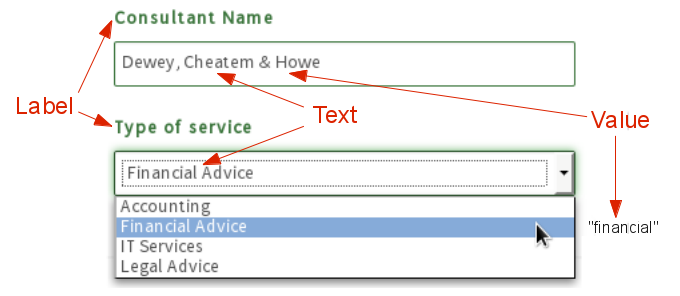
Obtaining jQuery Objects
A jQuery object can be obtained for each control in a Dynaform. This object contains the standard jQuery methods, such as hide() and show(), but it also contains custom methods added by ProcessMaker. It is recommended to use these custom methods, which are listed below, when manipulating Dynaform controls.
jQuery("#id") or $("#id")
All the controls in a Dynaform, including textboxes, titles, labels, links, images, etc., have an ID that can be used to obtain the jQuery object that ProcessMaker uses to manipulate a control. To obtain this object, use jQuery's selector method to access the control using its ID. is an alias for , so the selector method can be called either way:
or:This selector will return an object that has methods to manipulate Dynaform controls, such as getValue(), setValue(), getLabel(), etc. These methods can be called once the control object is obtained.
Example:
This code example obtains the object for a field with the ID "companyName" and then calls the object's setLabel() method to change the label to "NGO Name":
Note:Panel controls before version 3.0.1.5 did not have an ID, so it is not possible to use to obtain a panel object before version 3.0.1.5.
The is used to find an element in the current frame with the specified ID. A control's ID identifies the outer which holds the control, so calling will return an jQuery object for that div, which contains some custom methods to manipulate the control. See the sections below if needing to access the input field or label for a control.
To use a jQuery selector to search for a style class, use a (dot) followed by the class name. For example to search for all elements which use the "textLabel" class:
Likewise, it is possible to search for a tag. For example, to search for all the <span>s tags in the Dynaform:
To search for the elements containing a particular attribute, use . For example, to search for a of "clientId":
All of these searches can be combined. For example, to search for a <label> using the "control-label" class whose attribute is set to "clientId":
The DOM objects for all the elements which were found by a selector are placed in an array in the jQuery object. To find out how many elements were found by the selector, check the property of the jQuery object that is returned. For example, to make the text the color green in the first and last <span>s found by a selector:
var lastIndex = oSpans.length-1;//subtract 1 because array starts counting from 0.
oSpans[0].style.fontColor="green";
oSpans[lastIndex].style.fontColor="green";
Many jQuery methods, such as show(), hide() and css(), will operate on all the elements returned by the selector. These methods return an array of all the elements they operated on. A few jQuery methods such as attr() will only operate on the first element returned by the selector.
The jQuery selector can also be used to convert a DOM object into its jQuery object.
Example:
This code first obtains the DOM object for the "AccountNo" control, which is in the outer DIV, and set its border to the color red. Then, it uses to convert from a DOM object to a jQuery object, so that it can call its setValue() method to change its value to "ARB-501".
oAccount.style.borderColor="red";
$(oAccount).setValue("ARB-501");
NEVER do this:
Accessing Forms
The jQuery object for a Dynaform's <form> can be accessed by specifying the 32 hexadecimal character unique ID which ProcessMaker automatically generates for it.
Example:
Set the background color of the Dynaform with the ID "315000439561bfbb2880516004635450" to a light grey color:
Another way to access the Dynaform that doesn't require knowing its unique ID is to search for a <form>:
Accessing Controls in Subforms
The controls in subforms can be accessed just like normal controls, as long as their IDs are unique. If a control in a subform has the same ID as a control in the master form, then first obtain a subform's jQuery object using its 32 hexadecimal character unique ID which ProcessMaker automatically generates for it. Then, use jQuery's find() method to search for the control's ID inside the subform.
Example:
Set the value of a "companyName" textbox, which is found inside a subform with the ID "92799481456210d2fc9f249076819716":
Standard jQuery Methods
In addition to the custom ProcessMaker methods added to the jQuery objects for controls, jQuery also provides a library of standard methods which can be used to manipulate the controls. Listed below are some of the more useful ones frequently used in the examples on this page.
.attr()
.attr() is a standard jQuery method that gets and sets the value of attributes in elements. If the jQuery selector returns more than one element, it gets or sets the attribute of the first element.
To get the value of an attribute:
To set the value of an attribute:
Attributes are the values of an element that are usually defined in its HTML definition and are usually set before it is rendered on the screen. In contrast, properties are the values of an element that are usually set when it is added to the the DOM and rendered on the screen. Unlike attributes, which usually do not change and are fixed in the initial definition, properties often can be changed by the user. Attributes should be accessed with .attr(), whereas properties should be accessed with .prop().
In the above example, , and are attributes defined in the HTML code, but , , and are properties set when the element is rendered. For most values, it doesn't matter whether they are accessed with or , but it can make a difference in some situations.
For example, if the user changes the value of this textbox from "Acme, Inc." to "Wiley Enterprises", calling:
This code will return "Acme, Inc.", which was the initial value and is now fixed. However, calling:
This will return "Wiley Enterprises" which is the current property and can be changed.
Examples:
Set the attribute to "_self" in a link control with the ID "companyLogo", so it will open the link in the same frame where the Dynaform is located. Remember that the <a> element for a link control has an "a" element that needs to be found with the JQuery method .
Disable a dropdown box with the ID "selectContractor" when the user marks the "noContractor" checkbox.
if(newVal =="1"|| newVal =='["1"]'|| newVal =="1"){
$("#selectContractor").getControl().attr('disabled',true);
}
});
Note:control.setOnchange() reports the value of checkboxes differently based on the version of ProcessMaker:
In versions 3.0.0.1 - 3.0.0.16, use:
In version 3.0.0.18, use:
In version 3.1.0 and later, use:
.show()
show() is a standard jQuery method that can be used to show a control and its label in a Dynaform. Other controls will be moved down or to the right. It does this by setting in the outer DIV, which holds the control and its label.
Return Value:
Returns an object that holds information about the control. For example, a textbox with the ID "lastName":
"0":{
"jQuery111101537927321029987":101
},
"length": 1,
"context":{
"location":{
"constructor": {}
},
"image0000000001": {},
"jQuery111101537927321029987":1,
"_html5shiv": 1
},
"selector": "#lastName"
}
.hide()
hide() is a standard jQuery method that can be used to make a control and its label disappear in a Dynaform. Other controls will be moved up or to the left to take its place. It does this by setting in the outer DIV, which holds the control and its label.
Return Value:
Returns an object that holds information about the control. For example, a textbox with the ID "lastName":
"0":{
"jQuery111101537927321029987":101
},
"length": 1,
"context":{
"location":{
"constructor": {}
},
"image0000000001": {},
"jQuery111101537927321029987":1,
"_html5shiv": 1
},
"selector": "#lastName"
}
Example:
This example hides the field with the ID "productDescription" when the checkbox "noProduct" is marked. Otherwise, the field is shown.
if( newVal ==='["1"]'){
$("#productDescription").hide();
}else{
$("#productDescription").show();
}
}
$('#addProduct').setOnchange(showOrHideDescription);//execute when checkbox is changed
.css()
css() is a standard jQuery method that can be used to either read or change one of the style properties of a control.
To read a style property:
To change a style property:
Note: Style properties in JavaScript and DOM use camel case, whereas CSS uses lowercase with words separated by hyphens (), but can understand both styles, so and are the same.
Example:
Get the width of a dropdown box with the ID "billingMethod" and then add 200 pixels to it, if less than the width of the screen:
wide = parseInt(wide)+200;
if(wide < screen.width-100){
$("#billingMethod").getControl().css("width", wide);
}
Searching for Elements
jQuery provides a number of methods to search for elements, which are similar to the jQuery selector function , but they start their search based on where the element is in the Document Object Model (DOM) tree. Some of the more useful methods include:
- .find() searches within all the descendant nodes in the DOM.
Ex: Get the label of the "companyName" field: - .children() searches just within the child nodes.
Ex: Get all the rows in a Dynaform with the ID "3088873965642528e4073a7021293108": - .siblings() searches within the siblings nodes in the DOM.
Ex: Get all the controls in the same row as the "companyName" field: - .closest() searches for the closest matching element in the DOM.
Ex: Get the form for the "companyName" field: - .first() gets the first among the elements returned by the initial selector.
Ex: Get the first textbox in the form: - .eq(index) gets a specified element from the array of elements returned by a selector. The index starts counting elements from the number .
Ex: Get the fourth textbox in the form:
Backbone Objects for Controls and Forms
Backbone.js is a library that provides the model for Dynaforms and their controls. ProcessMaker offers three custom functions getFieldById(), getFieldByName() and getFieldByAttribute(), which are used to obtain the Backbone objects for Dynaform controls. In addition, the getFormById() function is used to obtain the Backbone object for the Dynaform.
The Backbone object supports most of the custom methods for manipulating controls, such as control.getValue(), link.setHref(), grid.getNumberRows(), plus it offers the setFocus() method which is not provided by the jQuery object.Functions to Obtain Backbone Objects
getFieldById('id')
The method returns an object that is generated by Backbone to manipulate the controls in a Dynaform. Its properties can be used to access the outer DIV for a control, which is used to resize controls when a form's width changes.
Parameter:
: The control's ID.
Return Value:
Returns a Backbone object whose properties may vary according to the type of control and whether the control has a variable assigned to it. All input controls should have the , , , , and properties. A textbox which has a variable assigned to it has the following content:
cid: "xxxxx",//client ID automatically assigned to the form by BackboneJS
$el: {...}, //Access to the jQuery object for the control's outer DIV
el: {...}, //Access to the DOM object for the control's outer DIV
dependenciesField: [...],
dependentFieldsData: [...],
formulaFieldsAssociated:[...],
model: {...},
parent: {...}
previousValue: "xxxxx",
project: {...},
__proto__: {...}
}
Inside the returned Backbone object, the property is the jQuery object for the that encompasses the outer of a Dynaform control. Likewise, the property is the DOM object for the that encompasses the outer of a Dynaform control.
For example, here is the HTML structure of the "company" textbox control that uses the variable:
<divclass="pmdynaform-field-text pmdynaform-edit-text form-group col-sm-12 col-md-12 col-lg-12 pmdynaform-field"
name="field-companyName"id="company">
<labelclass="col-md-2 col-lg-2 control-label pmdynaform-label"for="companyName">
<spanclass="textlabel"title="" data-placement="bottom" data-toggle="tooltip">Company Name</span>
</label>
<divclass="col-md-10 col-lg-10 pmdynaform-field-control">
<inputtype="text" autocomplete="off"value="" placeholder=""class="pmdynaform-control-text form-control" mask=""name=""id="form[company]">
<inputtype="hidden"value=""name=""id="form[company_label]">
</div>
</div>
</div>
Examples:
Set the value of the "totalAmount" field to "499.99":
This example sets the width of the "totalAmount" control's outermost DIV to 500 pixels. It accesses its jQuery object, which is located in its property, and then uses jQuery's css() function to set the width:
The following code does the same thing through the DOM object for the outermost DIV that is located in its property:
getFieldById("totalAmount").$el.children().css("background-color","yellow");
This is the same as using the jQuery selector function:
$("#totalAmount").css("background-color","yellow");
getFieldByName('name')
searches for control(s) by the name of the variable used by the control and returns an array of matching Backbone object(s).
Parameter:
: The name of the variable used by a Dynaform control. Set to "" (an empty string) to search for all the controls that do not have an assigned variable.
Returns an array of the Backbone object(s) for the control(s):
{
cid: "xxxxx",//client ID automatically assigned to the form by Backbone
$el: {...}, //Access to the jQuery object for the control's outermost DIV
el: {...}, //Access to the DOM object for the control's outermost DIV
dependenciesField: [...],
dependentFieldsData: [...],
formulaFieldsAssociated:[...],
model: {...},
parent: {...}
previousValue: "xxxxx",
project: {...},
__proto__: {...}
}
]
returns the same object as , so its , , and other properties can be accessed in the same way.
Example:
Get the ID of the control which uses the "clientType" variable:
getFieldByAttribute(attr, value)
The method searches for controls whose attribute matches a specified value.
Parameters:
: The name of a attribute. See the object below for all the available attributes.
: The value of the attribute to search for.
The attributes for a control can be found in the object, which can vary according to the type of control. See this list of the available attributes for controls.
Return Value:
Returns an array of all the Backbone control objects that match the search attribute. The properties of each object vary according to the type of control.
{ //properties for a textbox control:
cid: "xxx", //client ID automatically assigned to the control by Backbone
$el: {...}, //The jQuery object for the control's outer DIV
el: {...}, //The DOM object for the control's outer DIV
dependenciesField: [...],
dependentFieldsData: [...],
formulaFieldsAssociated:[...],
previousValue: "xxx",
model: {...},
parent: {...},
project: {...}
},
{ //properties for a radio control:
cid: "xxx", //client ID automatically assigned to the control by Backbone
$el: {...}, //The jQuery object for the control's outer DIV
el: {...}, //The DOM object for the control's outer DIV
clicked: false,
previousValue: "xxx",
model: {...},
parent: {...},
project: {...}
}
]
Example:
Return all the controls which occupy 6 columns and set the background color of their inner DIVs to blue.
for(i=0; i < aFields.length; i++){
aFields[i].el.childNodes[1].style.backgroundColor="blue";
}
getFormById('id')
The method returns the Backbone object for a Dynaform.
Parameter:
: The Dynaform's unique ID, which is 32 hexadecimal characters. It can be obtained by clicking on the border of the Dynaform to see its id property.
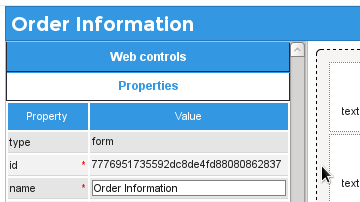
Return Value:
Returns a Dynaform object, with content such as:
cid: "view4",//client ID automatically assigned to the form by Backbone
$el: {...}, //The jQuery object for form
el: {...}, //The DOM object for form
factory: {...},
items: {...},
model: {...},
Project: {...},
viewsBuild:{...}
}
The object that is returned contains the object, which allows access to the form's jQuery methods and properties. Use the object to access it as a normal form object. For example, can be used to submit the form. can be used to reset the form back to its original values. is the number of inputs in the form and is an array of those inputs.
Example 1:
var total = parseFloat($("#amount").getValue())+ parseFloat($("#tax").getValue());
if(total >=500){
alert("Error: The amount plus tax must be less than 500. Please enter a new amount.");
returnfalse;//stop submit
}
returntrue; //allow submit
}
Methods and Properties in Backbone Objects
Apart from the custom methods to manipulate a control, the Backbone object holds the control's model information at:
The control.model.attributes is an object with all the attribute properties of the control and the control.model.keys() method can be used to obtain an array of the attribute names used by a control.
The method in the Backbone object is a jQuery selector which searches for elements inside the control. For example, to obtain the "companyName" control's label:
Methods for Controls
The following are custom methods provided by ProcessMaker to manipulate controls. All of these methods except for setHintHtml() are available in both the jQuery and Backbone objects for the control, so they can either be called with or (or one of the other 3 functions to obtain Backbone objects).
control.getLabel()
The method returns the label of a control in a Dynaform.
Note:This function does not work with panel controls since they do not have labels. Before version 3.0.1.3, this function was not available for radio controls.
Example:
When a user passes the mouse over a field with the ID "AccountNo", the following code checks whether its current label says "Account No." or "Client No.". Depending upon which label is used, it sets the border color of the "AccountName" or the "ClientName" field to blue in order to draw the user's attention.
var lol=$('#AccountNo').getLabel();
debugger;
if($('#AccountNo').getLabel()==="Account No"){
$('#AccountName').getControl().css("borderColor","blue");
}
elseif($('#AccountNo').getLabel()==="Client No"){
$('#ClientName').getControl().css("borderColor","blue");
}
});
$('#AccountNo').getControl().mouseout(function(){
//set back to original color:
$('#AccountName').getControl().css("borderColor","");
$('#ClientName').getControl
3.1.0.15 - 2020-01-09
- when deluxe Whozz Calling units don't provide an end call message, estimate the number of rings based on the duration — this is only useful when calls are received in rapid succession
3.1.0.14 - 2020-01-08
- added three fields to device setting info appearing in TSRs
- handle two Whozz Calling start messages without intervening end message nor time to timeout (this fixes recorded start time and duration when calls come in very quickly)
3.1.0.13 - 2020-01-07
- added command to Calls list context menu (access with right-click on Calls list) to only show new items in the calls list (you can change the "new" status on the same menu)
3.1.0.12 - 2019-04-14
- Contacts window: deleting numbers or patterns: enable Del key for deleting + don't confirm if global confirm deletes is off + keep position
- Contacts tab: when deleting contact, keep position
- Actions tab: when deleting action, keep position
3.1.0.11 - 2018-11-08
- remove expired and missing items from web cache automatically (expired items were not used previously, but they weren't deleted until ACID was restarted)
- if web cache folder is deleted while we're running, recreate it automatically
- gracefully handle missing cached web server files
3.1.0.10 - 2018-06-18
- Options window, Speech page: disable controls when sound is disabled and show explanation
- Options window, Speech page: added setting for speech audio output device
- include 32-bit or 64-bit in Windows version in Technical Support Report
- report Windows 8.1, Windows Server 2012 R2, Windows 10, and Windows Server 2016 in Technical Support Report
3.1.0.9 - 2018-01-02
- fixed problem where display panel might be partially or mostly obscured on systems with multiple monitors when the monitors aren't exactly side-by-side
3.1.0.8 - 2017-12-08
- fixed problems when deleting a call in progress
3.1.0.7 - 2017-07-20
- in the Calls list, include up to 128 characters and multiple lines (combined) in the Address column, if shown
3.1.0.6 - 2017-05-01
- corrected PerformAction examples in help
- fixed potential problem searching for Whozz Calling? Ethernet devices in Options window when none are currently being monitored — the problem resulted in an empty list for specifying the Whozz Calling? connection (serial or Ethernet) and/or neverending scanning and/or preventing Ascendis Caller ID from terminating cleanly
- debug versions: when no debug log path is specified, try using the temp folder if the log file can't be written to the application folder
- debug versions: when debugging is enabled, warn and disable logging to file if the log file can't be written to
3.1.0.5 - 2017-02-23
- fixed problem where an outgoing phone call would erase the name for a non-contact phone number (probably only happened with Whozz Calling? devices that track outgoing calls)
- Rebuild Phones command: added functionality to fix previously blanked names (to use this command, press and hold the Control and Shift keys while selecting "Rebuild Phones" on the "File" menu. You can release the keys once the "Rebuilding Phones" progress window appears.)
- fixed exceptions when restoring or repairing a database that refers to devices that are unknown or are no longer known
3.1.0.4 - 2017-02-10
- fixed possible incorrect data in CallInfo when action runs when call ends
3.1.0.3 - 2017-01-12
- when call ends, update call and contact information in CallInfo accessible from actions so latest information is available for actions when call ends (among other things, this means call end actions can access notes made during the call if changes are saved before the call ends)
3.1.0.2 - 2016-11-17
- added Call Frequency report (on View menu)
3.1.0.1 - 2016-06-23
- added Test Action button to item toolbar
3.0.0.44 - (never released)
- fixed occasional problem remembering HTML notification window size under Windows 8.1
3.0.0.43 - 2016-01-25
- show hourglass cursor when marking calls as new or read
- removed 'mark as new' and 'mark as read' commands from calls report — make these changes in the calls tab
- fixed possible exception when checking for automatic backup time when Windows has been running longer than 49 days
3.0.0.42 - 2015-06-25
- support dialing when using modem directly (i.e., not in TAPI mode)
3.0.0.41 - 2015-05-20
- replaced FindComet.exe with non-.NET version to avoid error message on some Windows XP machines
- updated Google search URLs (https is now required, apparently)
- make sure script editor is at least partly on screen when opened
3.0.0.40 - 2015-03-02
- fixed error messages on startup on systems with non-colon time separator
- fixed problem saving changes in Options window when devices were enabled before activating as a client
- fixed looking up area code from Contact list
3.0.0.39 - 2015-02-05
- attempted fix for composite devices sometimes being handled as separate devices
- added debug messages around instance data handling to help find cause of "already open in another user session" messages
3.0.0.38 - 2015-01-16
- increased default number of automatic backups to keep from 3 to 9
- Call window: can now delete labels by pressing "Delete" key while label combo box is dropped down and an item is highlighted (previously this would only delete the label if it was currently selected)
- Contact window: can now delete labels (on Extra tab) by pressing "Delete" key while label combo box is dropped down and an item is highlighted
- Contact window: can now sort labels (on Extra tab)
- Calls For window: open call window when a call is double-clicked
3.0.0.37 - 2014-12-09
- added duplicate calls report (accessible by View->Duplicate Calls, or by right-clicking on Calls grid and selecting "Delete Duplicate Calls...")
- added fake outgoing call command
- made fake Out-of-Area calls incoming
- fixed licensing problem with composite devices where a component device might be disabled
- attempted fix for problem downloading Whozz Calling? calls one customer saw
3.0.0.36 - 2014-10-15
- added CallBeganDate, CallBeganTime, CallEndedDate, and CallEndedTime columns to Calls Report
3.0.0.35 - 2014-10-07
- enabled CTRL+A shortcut for selecting all text in Call window Notes when Notes has focus
- trap exceptions when retrieving local IP address to fix exception one user reported when reopening Whozz Calling? Ethernet Link device
3.0.0.34 - 2014-08-06
- show phone number in outgoing call dialed from Ascendis Caller ID using modem
- prevent saving changes in Options window when Whozz Calling? device is enabled but Ascendis Caller ID is scanning for devices or serial ports
- fixed potential exception when shutting down Ascendis Caller ID while Options window is scanning for Whozz Calling? devices or serial ports
3.0.0.33 - 2014-06-19
- fixed problem accessing drive D: at startup reported by one user
3.0.0.32 - 2014-06-09
- CTI Comet: cleaned up log messages and device configuration
3.0.0.31 - 2014-06-04
- added support for CTI Comet CID capture device
3.0.0.30 - 2014-05-23
- log start and end of scanning for serial ports and Whozz Calling? Ethernet devices in Options window
3.0.0.29 - 2014-05-19
- Find in Contacts list now searches Notes field
3.0.0.28 - 2014-05-07
- fixed rare problem with auto import contacts date setting on some systems
3.0.0.27 - 2014-04-18
- improved handling of call waiting calls on composite devices (Please note that call waiting ID support, as implemented in Ascendis Caller ID and the Zoom 3095, is called "Snooping CID" in the Zoom documentation. It only works when another phone device that supports call waiting ID is connected in parallel with the modem. In our tests, it works if the phone in use ("off-hook") supports call waiting ID, but not if a non-call waiting ID phone is being used. It is apparently not sufficient for a call waiting ID phone to be plugged into the phone line — it must also be in use ("off-hook").)
- remember last contact selected when assigning number to existing contact
- fixed cosmetic issues in Call Notes window, especially with custom fonts
- fixed cosmetic issue in Auto Import Contacts window
3.0.0.26 - 2014-03-03
- close network connections before suspending and open on resume to fix potential hang problem when network connection is not available on resume
- fixed problem when changing composite device description
- improved composite device call processing when call information is not received from devices in close proximity in time
- fixed problem where call end was sometimes reported as before call start
3.0.0.25 - 2014-02-07
- added contact notes, contact extra, and contact custom fields to calls list and calls report (In order to see these fields in the field customization drop down list, you may have to reset the grid by right-clicking on it and choosing "Reset Grid". This will remove all grid column customizations!)
3.0.0.24 - 2013-12-13
- added "Tell EZLynx" action
- improved error handling when database can't be opened at startup
- added "Tell Time Matters" action
- added DDE_Send.exe
- when dialing from the contact list, now dials the phone number for the selected contact instead of the first phone number in the database for the contact
3.0.0.23 - 2013-08-13
- added help for secure web server and web access editor
- per-folder secure web access: check access file in parent folder(s) if username is not found in local access file
- web access editor: minor cosmetic changes
- web access editor: fixed bug where changes to a user were not detected for purposes of confirming cancel
- web access editor: fixed bug where backup was deleted after cancel confirmation prompt if cancel was canceled
- Options window: minor cosmetic changes to Web/TiVo Server page
3.0.0.22 - 2013-08-08
- support more date formats when importing CSV calls
- sped up CSV call importing ~36%
- Options window, Mail tab: don't display password
- added per-folder web server authentication
- added secure web server
- install: backup files in webroot folder before overwriting
3.0.0.21 - 2013-07-16
- fixed last 1 or 2 digits of long phone numbers (> 12 digits) on Whozz Calling? devices appearing in name (note that Whozz Calling? devices only support up to 14 digits for phone number and additional digits will still appear in name)
3.0.0.20 - 2013-07-10
- fixed exception when deleting all contacts and importing contacts
- support more date formats when importing new contacts
3.0.0.19 - 2013-07-09
3.0.0.18 - 2013.04.02
- added more speech error descriptions
3.0.0.17 - 2013.04.01
- attempted fix for reported speech exception ($8004503A) for one Windows 8 64-bit user
- encode control characters in XML export files
3.0.0.16 - 2013.02.24
- fixed potential problem preventing startup on Windows Server 2012 64-bit (and other versions of Windows?)
- made ###NUMBER### a synonym for ###DISPLAYNUMBER### in contact blocks in web server pages
3.0.0.15 - 2013.01.10
- fixed problem accessing modems directly in Windows 8
3.0.0.14 - 2012.12.13
- added call waiting ID support for Zoom 3095 in direct mode (aka 'not TAPI') To use: in the Options window, Devices page, Modems and TAPI sub page, get properties on the modem and enable "Enable call waiting id". Notes:
- When a call waiting call is detected, the action for the caller runs but Hangup and PhoneSound commands are blocked since Ascendis Caller ID doesn't know which party is on the line. Since the modem doesn't distinguish between normal and call waiting ID messages, Ascendis Caller ID assumes a caller ID message without a preceding ring is a call waiting ID message. In some countries, this could prevent Hangup and PhoneSound from ever working.
- Call waiting id detection may vary with the phone being used when the call is detected — this is a hardware limitation.
- fixed deleting call from Calls Report using navigator toolbar
3.0.0.13 - 2012.12.07
- fixed word wrap in Contact window's notes memo
3.0.0.12 - 2012.10.29
- if no devices are open, periodically reopen configured devices — fixes problem when a modem is not available when we start but becomes available later
- fixed problem with automatic backups if system goes to sleep during backup
- default network status window to status page since log is disabled by default
- reconnect to network servers when waking from sleep — if configured to poll first, this will download calls since going to sleep
- fixed deleting calls from navigator toolbar
3.0.0.11 - 2012.10.02
- fixed potential duplicate calls when using Whozz Calling? Ethernet Link models on some networks
- turned off "Process selection" for calls report since it doesn't work with this type of grid
- changed "Buy Now" links to go to the web pricing page, so user must choose the number of phone lines desired
3.0.0.10 - 2012.06.21
- don't show date-based filter items in calls report duration filter drop down
- fixed problem filtering calls report on duration
- added "Fake Out of Area Call" command (available with other fake call commands on status bar context menu)
- when refreshing the contact list, retain the focused row and, if the number of records hasn't changed, the selections
3.0.0.9 - 2012.06.01
- support "RING L...,N..." messages (from NetMod ISDN modem)
3.0.0.8 - 2012.05.30
- don't assert when an empty string is passed to Speak command
3.0.0.7 - 2012.05.26
- sped up CSV contact importing 150 times for some CSV files
- handle more date formats when importing CSV contacts
- added port and authentication mode to send mail log message
- use ascendis.com internally instead of www.ascendis.com
- activation fix for some machines
3.0.0.6 - 2012.03.05
- log phone number assignments that occur because of pattern matching to Activity Log
- when name AND number patterns are specified for a single contact pattern, both patterns must match for the phone number to be assigned (previously either match caused the phone number to be assigned)
3.0.0.5 - 2012.03.02
- fixed problem where some calls received on non-Lite Whozz Calling? devices could be processed as two calls (wait longer for device's response to "V" command; when "V" response and detail messages are received from device, we know it's not Lite) — problem was seen on a Whozz Calling? 4 Ethernet Link
3.0.0.4 - 2012.02.09
- don't perform automatic backup if calls are in progress
- don't configure Whozz Calling? Ethernet devices if multiple devices are found and we're not configured to talk to one on the local subnet (this fixes a problem we've seen where multiple Whozz Calling? Ethernet devices cause a device configuration error which causes one device to hang up on all calls)
- Options window: allow re-scanning for network Whozz Calling? devices (choose appropriate option in Whozz Calling? port combo box)
- Options window: scan serial ports and network Whozz Calling? devices in background to speed opening window
- support choosing a single Whozz Calling? Ethernet Link device (only works for devices on same subnet)
- when importing calls via CSV when RawNumber field is not specified, use any provided name field
- fixed problem introduced in 3.0.0.3 which caused automatic backups to be performed too frequently
3.0.0.3 - 2012.01.19
- Repair Modem: fixed problem where wrong modem settings might be used if a modem with the same modem id was previously recognized by Ascendis Caller ID
- Repair Modem: fixed problem repairing TAPI responses on some 64-bit machines
- trap and report additional types of automatic backup failure
- Repair Modem: don't check Windows' TAPI settings and responses if the modem is configured for direct mode
- Repair Modem: don't change modem access mode to TAPI if the modem is configured for direct mode
3.0.0.2 - 2011.12.29
- reopen devices after repairing modem if we're not restarting
- immediately disable all devices once activated as client
- fixed problem with Skype version 5 (which no longer installs Skype4COM)
3.0.0.1 - 2011.12.05
- fixed activation problem with computers with varying max CPU clock speed
2.3.0.49 - 2011.10.13
- Repair Database command now optimizes too
- installs empty and initial database CIDDB files in data folder too for ease of access
- increased default main window width
- enable new TAPI devices if activation allows and "Tell me about new devices" is enabled
- fixed problem importing version 1 database
- added commands to import version 1 settings and version 2 settings and database
- when importing version 1 database, default to installed database folder; check folder before importing; backup existing database first and restore on failure
2.3.0.48 - 2011.09.22
- tweaked Network Status window, including increasing status bar height
- fixed potential exception on some machines when printing call notes
- fixed rare exception on some machines when using the Run command in actions
2.3.0.47 - 2011.09.16
- fixed bug where downloading calls from Whozz Calling? memory used computer time if "prefer computer time" was enabled (memory calls should always use device time)
- fixed problem where minimizing display panel all the way and then exiting Ascendis Caller ID prevented the display panel from being restored
- fixed potential problem when rotating Line Monitor logs in debug builds
- Find Actions window: fixed searching (broken with font changes in 2.3.0.31) and accelerator for Text field (broken with font changes in 2.3.0.35)
- fixed possible date format issue in Find Calls window
- "Add Locations from Contacts" command: include country code
- updated help
2.3.0.46 - 2011.09.09
- updated help
- tweaked Edit Call window to allow slightly larger fonts
- fixed some font size issues in Edit Location window
- corrected help on contacts CSV files; added simple sample
2.3.0.45 - 2011.09.02
- don't let user select a Ring action that hangs up
- warn user when choosing a Ring action other than "Ring" or "Do Nothing"
- reduced default font size for HTML notification window
- increased default size of Line Monitor window
- clarified logged message when raw CID data includes other info
- updated help
2.3.0.44 - 2011.08.25
- added automatic backups (on by default; see File -> "Auto Backup Database..." for configuration)
2.3.0.43 - 2011.08.24
- improved handling of unexpected TAPI error codes
- trap TAPI error when getting system's country code and default to "1"
2.3.0.42 - 2011.08.21
2.3.0.41 - 2011.08.19
2.3.0.40 - 2011.08.19
- more error codes for activation failure
2.3.0.39 - 2011.08.18
- new actions now use {SpeakName} instead of {Name} in default speak text
- "Empty Database.ciddb" is now literally empty — no records exist in any of the lists
- now installs "Initial Database.ciddb" into Ascendis Caller ID Program Files folder — this contains the database as it appears on first install
- added "Speak Name" and "Speak Name and Number" actions (these will only appear for new installations; if you want to add these simple actions to an existing installation, import "Standard Actions.xml" from the Program Files folder using File -> Import -> Actions)
- the "Default" action now speaks the phone number only if the caller is not a contact and the number is not the same as the name (this change will only appear for new installations)
- when a contact is deleted, recompute the name fields for the phone numbers assigned to the contact from the raw caller id name (as would happen when the call is first received) — fixes problem with names "sticking" to a phone number after a contact is deleted
- fixed problem with TAPI modem on some systems where script (and speaking) could be triggered twice
- fixed race condition with scripts that could cause logged errors
- updated distributed Locations table to version 2.4 (no content changes; this just saves Ascendis Caller ID from having to update it on first run)
2.3.0.38 - 2011.08.10
- added default CountryCode to Locations table so old locations aren't lost during update and old XML Location files can be imported (system's country code is used for default; this should be correct for most users but if not you will have to change each Location record manually or export to CSV, alter in a text editor or spreadsheet program, and import after deleting existing Locations)
- trap errors during database updates and restore database
- default to XML file type in export dialogs
2.3.0.37 - 2011.08.05
- added option to disable Whozz Calling? device memory call download (in Options window, Devices page, Whozz Calling? subpage, Options)
- fixed problem where calls in progress when Ascendis Caller ID was terminated would show incrementing call duration when Ascendis Caller ID was restarted and a call was active
2.3.0.36 - 2011.07.22
- include US area codes in Locations list (existing customers can import "US Area Code Locations.xml" from the Ascendis Caller ID Program Files folder)
- support importing Locations from XML files
- support overwriting Locations when importing from XML files (hold down CONTROL key when selecting Import -> Locations command)
- added CountryCode column to Locations table (this allows locations for multiple countries in the same Locations list)
- added DWS script function GetDataFolder
- Location window: widened latitude and longitude fields; use spin edit for timezone
2.3.0.35 - 2011.07.13
- network log options window: fixed cosmetic issues with some fonts
- Auto Import Callers window: fixed cosmetic issues with some fonts
- Options window: fixed cosmetic issues with some fonts
- fixed cosmetic issue in some message windows
- Find windows: fixed button truncation with some fonts
- contacts window: increased drop down height of Label combo box on Extras page
- minor improvements to pasting activation code
2.3.0.34 - 2011.07.01
- added "All users share same settings" option in Options window, Advanced page (when checked, settings are stored in common ini file)
2.3.0.33 - 2011.06.30
- gracefully handle problems writing to line monitor log
2.3.0.32 - 2011.06.28
- fixed problem where last line of displayed messages might be hidden depending on font used
2.3.0.31 - 2011.06.27
- added mute toolbar button (duplicates functionality of "Disable sound (including speech) in all actions" checkbox on Sound page of Options window)
- "Calls For" report: autosize column widths initially; use Windows' short date format
- fixed problem with speech "Play" button in Edit Action / Easy Action window
- when tray icon is clicked while Options window or another modal window is open, bring app to foreground and appropriate window to front
- added program font setting (Options window, General page) (see size note, below)
- use current system window title font (note that in some windows a smaller size of the selected font will be used if the selected size is too big for the window)
2.3.0.30 - 2011.05.26
- fixed new calls count
- in Options -> Devices -> Whozz Calling? -> "Enable Whozz Calling? device on:", added option to scan for more serial ports (initially only 16 serial ports are scanned)
- in beta versions, only keep up to last 1MB of Line Monitor log in file (the Line Monitor log is not kept in a file in normal release versions) — this can be configured in the context (right-click) menu of the Line Monitor button bar
- added "Notes" column to available columns in the contacts list
- use activation codes instead of old registration method — THIS MEANS CUSTOMERS OF VERSIONS BEFORE VERSION 3 (which is not yet for sale at the time of this writing) WILL BE USING TRIAL MODE UNTIL THEY ACQUIRE AND ENTER AN ACTIVATION CODE. Customers who purchased Ascendis Caller ID on or after May 1, 2010 can get a free activation code by using the following form: http://ascendis.com/callerid/upgrade_v2_to_v3_free_form.php If your email address has changed since your purchase, the free upgrade will fail. In that case, please submit a support request. Please include your registered email address in the request. http://ascendis.com/callerid/support_form.php Long-term customers who have provided useful feedback in the past can get a free activation code by submitting a support request. Please include your registered email address in the request. http://ascendis.com/callerid/support_form.php
2.3.0.29 - 2011.03.22
- don't use or free db accessors created from main thread while executing scripts
2.3.0.28 - 2011.03.09
- fixed problem with some modems under TAPI when they only report the phone number which, within certain timing constraints, could result in a call being reported as two calls, one with correct phone number and one unknown
2.3.0.27 - 2011.01.03
- fixed EOverflow exception when speaking through USB sound device for one user
- fixed potential problem with configured volume not respected around speech
- removed SAPI4 speech support
2.3.0.26 - 2011.01.03
- reduced period of time after user interacts with call list during which program will not automatically select new call from 10 seconds to 3
- improved Whozz Calling? initialization when device is busy when we start
- Whozz Calling 2: erase memory while downloaded calls are being processed
- workaround divide-by-zero exception that can occur under Windows Vista when speaking synchronously (SpeakWait command)
2.3.0.25 - 2010.12.24
- added SpeakWait command to DWS scripts (it waits for speech to finish before returning) (SpeakWait is only compatible with SAPI5 voices)
2.3.0.24 - 2010.12.23
- fixed problem downloading Whozz Calling? 2 memory calls when number of calls in memory was more than system could process fast enough
- trap and log exceptions when repositioning contacts list after changing a contact (rare, but one user encountered it)
- automatically disable detail mode for Whozz Calling? devices reporting too many on-hook messages (as can happen when too many phone devices are connected while device is using voltage detection)
2.3.0.23 - 2010.11.24
- fixed potential exception when deleting all contacts
- fixed exception when deleting last (or all) calls
2.3.0.22 - 2010.11.21
- Repair Database command: fixed handling of two phone records that resolve to the same "match" phone number
- Delete Duplicate Calls command: ignore milliseconds when comparing calls — this fixes problem where calls imported from exported files (which don't include milliseconds) were not recognized as duplicates
- trap and report exceptions when exporting
2.3.0.21 - 2010.11.04
- fixed exception when reporting exception when getting window handle to bring app to top on new call
2.3.0.20 - 2010.10.14
- fixed potential startup problem (reported by one user)
- show hourglass cursor when rebuilding contacts list
2.3.0.19 - 2010.10.01
- fixed bug where an action that deleted a call could try to delete the call more than once, although subsequent attempts would fail
- added message type descriptor to Activity Log entries
- fixed problems with commands not properly visible or enabled (introduced in 2.3.0.17)
- added "Find in Contacts" command to calls and calls report lists right-click menu
- fixed problem where dates in grids were occasionally displayed in wrong format
- fixed exception when editing, changing, and saving a contact while viewing the contact list
2.3.0.18 - 2010.09.24
- fixed polling case with multiple calls from same phone number where phone number already existed on client
2.3.0.17 - 2010.09.22
- minor changes to "Item Toolbar" items' visibility / state / position for consistency
- fixed excess CPU usage at idle (caused by action updaters that hit database)
- trap exceptions when getting window handle to bring app to top on new call
2.3.0.16 - 2010.09.16
- "Show or Add in Outlook" action now specifies CompanyName field in Outlook contact and files phone number according to the phone number description in the Ascendis Caller ID contact if one exists (to add the new Outlook actions to an existing database, hold down the CONTROL key while selecting File -> Import -> Actions, then choose the "Outlook Actions.xml" file in the Ascendis Caller ID program folder)
- hide many context menu items when multiple calls or contacts are selected
- added "Dial Phone" toolbar button for Calls, Calls Report, and Contacts lists
- added "Perform Action" to call context menu
2.3.0.15 - 2010.09.14
- gracefully handle modems that return unexpected voice compressor syntax (like MultiTech Systems MT5634ZPX-PCI)
- more work on outgoing call information detection for TAPI devices
2.3.0.14 - 2010.09.10
- use display name in popup notification window instead of first name + last name
- improved outgoing call information detection for TAPI devices
- doubled amount of Line Monitor data kept while running
- fix for problems displaying images in display panel and HTML call notification window on some systems
- trap exceptions when displaying images in HTML call notification window
- attempted fix for rare exception on shutdown when using YAC
- made Status page the default page when showing the Network form
- increased default modem call timeout to 30 seconds in direct mode (this can avoid phantom "No Caller Information" call after valid caller id capture on slow systems; we recommend direct-mode modem users increase the value manually since the new default won't override existing settings)
- use configured modem call timeout in direct mode
- reduced possibility of modem call timing out in direct mode because modem message had been received but not yet processed (resulting in a single call being interpreted as two calls)
2.3.0.13 - 2010.08.30
- don't change the database when tracking active call duration (refreshing the calls table seems to be enough to update call duration, which is calculated) — this should avoid database engine error exception one customer saw
2.3.0.12 - 2010.08.23
- fixed problem preventing "Calls for this Number" and "Calls for this Contact" reports from working when "Keep child windows on top of main window" was enabled
- Options window, Network page, Web/TiVo server sub-page: disable web root controls unless web server is enabled; added help clarifying web root setting
2.3.0.11 - 2010.08.20
- fixed problem where system dialogs (font, color, etc) could be hidden behind other windows when switching back to Ascendis Caller ID or when call is received
- changed default background color for HTML notification window so "Make background transparent" (in Transparency settings window) works
- added Print button to Find windows
- Outlook actions now look for caller in contact folder and subfolders
- Edit Action window: select all text in script memo (Advanced actions) when CTRL+A is pressed in it
- fixed bug where COM methods in advanced scripts could fail because COM library wasn't initialized
- added INCLUDE_ACTION directive to DWS scripts
- when deleting a contact, use display name, first name + last name, or company in confirmation as needed
- added DWS script functions ContactCount, PhoneCount
2.3.0.10 - 2010.08.16
- after successfully launching an editor for editing a notification layout, change the selected layout setting to the custom layout
2.3.0.9 - 2010.08.04
- fixed problem deleting data files in uninstaller when requested to do so
- added HTML call notification window (select in Options window, Notification page; right-click on notification window (use Preview if desired) for configuration options) (NOTE: this uses a built-in HTML control, and not the Internet Explorer, Mozilla, or other browser engine. JavaScript is not supported, and CSS support is limited.)
- added CallInfo fields PictureHeight, PictureWidth
- added script commands GetSettingBool, GetSettingStr
- made script function "CallCount" thread safe
- don't used cached web page if html template was subsequently modified
2.3.0.8 - 2010.07.28
- reopen devices when resuming after sleep
- default to '0.0.0.0' in network settings server bind addresses
- fixed problem printing single call
- update call duration in calls list during call (for Whozz Calling? and other devices that track call duration)
- log messages when a second instance is started
2.3.0.7 - 2010.06.28
2.3.0.6 - 2010.06.21
- added date format info to Technical Support Report
- track and note changes in date format in Activity Log
- reduce font size of progress bar in status bar (used for report progress) to better fit some displays
2.3.0.5 - 2010.06.15
- don't change the display panel to show an outgoing call if an incoming call is in progress
- don't change the display panel to a different existing call when the status of the call changes
2.3.0.4 - 2010.06.10
- added option to only display contact window for known contacts when "Open contact window for each call" is enabled in Options/Notification
2.3.0.3 - 2010.06.03
- trap rare exceptions when displaying a message and attempt to display using alternate methods
2.3.0.2 - 2010.05.24
- Rebuild Phones command: filter non-numeric characters from phone numbers not containing letters as is done when calls are first received (the Rebuild Phones command is normally hidden; to see it hold down the control and shift keys while clicking the File menu)
- network log: added line separator commands to button bar context menu
2.3.0.1 - 2010.04.27
- dynamically widen "active calls" pane of status bar if needed
- added "Calls for
2.2.0.32 - 2010.04.21
- workaround HiRO H50113 modem bug in direct mode with respect to CID reporting
- added CTRL+M shortcut to view the Line Monitor
- added CTRL+T shortcut to test action in Calls and Calls Report lists
- Repair Database now fixes invalid contact pictures by deleting them (this is not normal, but could occur if a pre-2.2.0.3 version of ACID is used with a 2.2.0.3 or later database)
- trap and warn if an invalid contact picture is detected
- allow overwriting contacts during import (hold down CONTROL key while selecting "File -> Import -> Actions")
- don't show Edit Action button for Locations list
- always use CTRL+A for Edit Action, CTRL+E for Edit Contact, and CTRL+K for Edit Call Notes regardless of which list is showing, if the command is allowed
- show note about database compatibility in installer if relevant
- backup and repair database at install time if previous version < 2.2.0.32
- automatically backup database before repair
2.2.0.31 - 2010.04.15
- allow overwriting actions during import for XML files (hold down CONTROL key while selecting "File -> Import -> Actions")
2.2.0.30 - 2010.04.14
- don't log "Could not find phone number format rule" message when phone number is empty (such as for "No Caller Information" calls)
- fixed potential problem resulting in delayed end of sound detection in direct mode
- fixed potential problem using PhoneSound in TAPI mode
- added "Post-answer delay" to Advanced Device Properties window
- added Help button to Advanced Device Properties window
- added "Use DTR for hangup" setting to Device Properties window
- hangup changes: changes introduced in previous version are not compatible with all modems, so now they're only done by default for Zoom 3049C and Zoom 3095, and only in direct mode
- Find commands: restore find window if minimized
- trap potential exceptions when generating settings portion of Technical Support Report
2.2.0.29 - 2010.04.02
- improved TAPI-mode data modem hangup success rate for some modems (no failures after change in our tests)
- fixed bug in Contact window where changing only the action and closing the window by pressing the Enter key didn't save the change
- hang up after action that plays sound file(s) on Skype if action didn't hang up (now the same as other devices)
- convert sound files to Skype format before playing (improves sound file compatibility with Skype, which only supports PCM/16kHz/16-bit)
- fixed problem hanging up and playing sounds with Skype connections (problem was introduced in 2.2.0.20, when separate script threads were added)
- fixed bug where sound files for easy actions and old-style scripts were not found if the path was not specified even if they existed in the program folder (this fixes the problem with the built-in "Ring" action)
- added "Duplicate Action" command to Actions context menu
- improved direct-mode modem hangup success rate for some modems (from about 90% to 100% in our tests)
2.2.0.28 - 2010.03.20
- automatically resize pictures when pasted or loaded into Contact window (pictures are resampled to a height of 200 pixels if larger unless "Autosize Images" is disabled on display panel context menu)
- improved real-time networking speed, especially with large action scripts or contact pictures
- serial mode message normalization
- don't display message on startup when modem in serial mode does not support voice mode (it will still be logged)
2.2.0.27 - 2010.03.17
- show meaningful error message instead of generic exception when display panel can't be drawn (extremely rare)
2.2.0.26 - 2010.03.12
- don't try to use serial port if open fails
- stop trying to open serial port if SetCommTimeouts fails
2.2.0.25 - 2010.03.11
- don't stop trying to configure serial port if SetCommTimeouts fails
2.2.0.24 - 2010.03.10
- fixed problem when changing modem settings from TAPI to direct mode while scripts are running
- show progress window when waiting for scripts to complete while shutting down or changing modem settings
2.2.0.23 - 2010.03.02
- updated help to include changes since version 2.2.0.0
- Activity Log: added F3/Find Next command
- reduced delay before playing sound over phone in direct mode
2.2.0.22 - 2010.02.26
- fixed problems remembering main and find windows size and position
2.2.0.21 - 2010.02.25
- increased network client polling speed and responsiveness
- polling: don't send the same auxiliary records more than once during a single poll
2.2.0.20 - 2010.02.24
- sped up network logging
- scripts run in separate threads (fixes freeze while playing sounds over modems in direct mode)
- improved direct mode sound playback for HiRO H50113 (for best results, use sounds in 4bit IMA ADPCM format)
2.2.0.19 - 2010.02.19
- fixed problem converting wave files for PhoneSound in direct mode that may have added short garbage to end of sound
2.2.0.18 - 2010.02.17
- don't pass signed PCM sound data to modem in unsigned PCM mode and vice versa — fixes noisy sound issues with Zoom 3095 on some wave files
- throttle sound data to modem in direct mode — fixes sound playback speed problem with long wave files
2.2.0.17 - 2010.02.15
- trap and report exceptions when rebuilding database
- implemented delete in Find Calls window (press the "DEL" key to delete a call)
- fixed problem with 'New Calls' count when deleting multiple calls from calls report with mixed 'New' status
- mark calls report dirty when 'New' is changed in a call
- added fixed width font checkbox to Line Monitor
- trap registry exceptions when "AttachedTo" registry entry for modem is the wrong data type (one user with a non-standard device reported this error)
- added limited PhoneSound support in direct mode. This has been tested mostly successfully in the following OS/modem configurations: o Vista 64-bit with Zoom 3049C (best sound), Zoom 3095, and HiRO H50113 (driver v.2.1.88.0; sound usually not so good) o Vista 32-bit with Zoom 3049C (best sound), Zoom 3095, and HiRO H50113 (driver v.2.1.88.0; sound usually not so good) o Windows XP with Zoom 3049C (best sound), Zoom 3095, and HiRO H50113 (driver 2.1.70; sound poor) o Windows 7 64-bit with Zoom 3049C (good sound), Zoom 3095, HiRO H50113 (driver v.2.2.98.0; good sound) Notes: - The HiRO H50113 is an external USB winmodem and seems pickier than the hardware Zoom modems. You may or may not have luck with it. - If your modem can't play a particular sound file or ACID can't convert it to a compatible format, check the Line Monitor for a list of formats your modem supports (search for "ModemFormatID"; the list may be appear cryptic, but it contains the information you need to make an optimally compatible sound file). Use your favorite sound editor or Audacity, a free, open-source sound recorder and editor: http://audacity.sourceforge.net/
2.2.0.16 - 2010.01.30
- support filtering phone numbers from device to remove unwanted digits (such as outgoing line selection): To use, add a phone format rule (Options window, Format page) and include an 'F' in the position of the digit to remove. Be sure to make the conditions explicit so numbers that shouldn't be altered aren't (to remove a leading digit, you will probably need a "Length = n" condition AND a "Number starts with m" rule).
- phone number formats: detect changes to format conditions and rebuild phone records
- Line Monitor: give focus to memo when find panel is closed; added F3/Find Next command
- reopen modems configured for serial mode if "Prefer raw caller id" was changed in Options window (device properties)
2.2.0.15 - 2010.01.29
- don't allow viewing network window when networking is disabled
- added help for DWS date and time functions
- fixed enabling/disabling/registrations problem with Whozz Calling? devices in Options window introduced in 2.2.0.14
- enabled scroll bars in Script Editor
- added DWS functions AnsiSameStr, AnsiSameText
- when receiving actions from an ACID server, gracefully handle case where an action with the same name but a different unique identifier exists
2.2.0.14 - 2010.01.26
- Options window: when user enables Whozz Calling? device, adjust the number of lines monitored (and tell user) if insufficient registrations are installed
2.2.0.13 - 2010.01.20
- fixed problem where device might not be opened even though it was enabled in the Options window, Devices page: the problem happened when another device with the same TAPI ID and name had been used by an ACID server to which the computer was a client
- modem repair: enable Try Again button when modem could not be opened
- fixed hangup command for some modems (like HiRO H50113 (Agere chipset)) in TAPI mode
2.2.0.12 - 2010.01.18
- only include unused TAPI devices in Line Monitor during initialization if the total number of TAPI devices is less than or equal to 10 (used TAPI devices are always listed) — this will shorten the Line Monitor in extreme cases so it retains more diagnostic data
2.2.0.11 - 2010.01.12
- modem repair: other tweaks
- modem repair: fixed endless loop and stack overflow when insufficient rings were received
- Line Monitor: include device name if multiple devices are used
2.2.0.10 - 2010.01.06
- Whozz Calling? calls in memory (for units with memory): corrected call start and end times (they were off by call duration); use previous year if call day and month is more than 2 weeks in the future (units do not store year of call)
- sped up Line Monitor processing when displayed and enabled
- added Find function to Script Editor (press CTRL+F to access)
- modem repair: support repairing modems already open directly
- modem repair: open modem directly if TAPI open fails
- when performing an action for a call, log the action name to Line Monitor
2.2.0.9 - 2009.12.31
- modem repair: added raw cid support
- modem repair: fixed MESG response generation when NMBR response <> "NMBR"
- recognize "DDN_NMBR" as caller's phone number in TAPI bypass mode
2.2.0.8 - 2009.12.21
- trap and report exception if a problem prevents a TAPI line from being accessed during communications spinup
- fixed bug where an outgoing call placed through a modem but not through Ascendis Caller ID would trigger the action for "No Caller Information" unless configured to ignore outgoing calls
- added support for raw caller id for modems when not using TAPI (check "Prefer raw caller id" in Device Properties window from Devices page of Options window)
2.2.0.7 - 2009.12.17
- remember "Fixed width font" setting in Activity Log window between executions
- fixed problem with some modems under TAPI that change call handles during the call that could cause a single call to be interpreted as two calls
- fixed potential problem with modems under TAPI on slow or busy machines that could cause a single call to be interpreted as two calls
- added Find function to Line Monitor and Activity Log windows (press CTRL+F to access)
- fixed problem accessing modem responses in modem analysis part of support report on normal user accounts in Vista and Windows 7
2.2.0.6 - 2009.11.23
- fixed race condition with realtime network clients which could eventually prevent communications with clients and proper application shutdown
- added DWS script functions IPos, Contains, ContainsText
- added CallInfo.DeviceName (synonym for CallInfo.RawLine)
- when importing or deleting locations, update the calls list immediately
2.2.0.5 - 2009.10.20
- when importing contacts, don't split first name or last name fields
- fixed CallInfo when accessed from nested action
2.2.0.4 - 2009.10.16
- fixed problem importing contacts from CSV file when LastName was the only name field provided
- added documentation for StringReplace to DWS String Library topic in Help
- added following advanced DWS action commands: GetTempPath, GetAppFolder, FileExists, StartsWith, EndsWith
- disable Ctrl+D shortcut (for dialing) when the command is hidden (fixes exception in that case)
- added "PerformAction" command to advanced DWS actions
- Edit Action window: when an action type is changed to advanced, automatically add "//LANGUAGE=DWS" if the script is empty
2.2.0.3 - 2009.10.13
- fixed problem when polled by version 1 network clients
- added "RunWait" command to advanced DWS actions
- added "Edit Action" and "Add Action" commands to item toolbar when Action list is visible
- after changing an action's name, position the Action list on the action
- after creating a new action, position the Action list on the new action
- Options window, Devices page, Skype sub-page: disallow enabling Skype if all registrations are already in use
- support overwriting local contacts when contact is received from server via polling
- Options window, advanced Network page, Client sub-page: added "Overwrite local actions" setting: when enabled, actions sent from server will replace local actions when they exist; when disabled actions sent from server will only be accepted if the action doesn't already exist
- Options window, advanced Network page, Server sub-page: added settings for sending contacts and actions to network clients (previously contacts were always sent; sending actions only applies to version 2 network clients)
2.2.0.2 - 2009.09.30
- fixed rare "RichEdit line insertion error" when editing a script
2.2.0.1 - 2009.09.29
- fixed problem where a small notification window would show the wrong call data if the call was deleted while the window was showing
- when testing an action from the Action list, load the test caller's contact info if a contact is defined
- added ContactCallsMade, ContactCallsReceived, ContactFirstCalled, ContactLastCalled, PhoneCallsMade, PhoneCallsReceived, PhoneFirstCalled, PhoneLastCalled to CallInfo object
2.2.0.0 - 2009.09.25
- all changes since 2.1.2.0
2.1.2.21 - 2009.09.15
- added Ini and Classes libraries to DWS scripts; see DWS manual for usage (manual available on DWS web site: http://sourceforge.net/projects/dws/ )
- help: added PhoneSound
- help: added link to DWS on web and suggestion for checking DWS manual for additional DWS functions
2.1.2.20 - 2009.09.10
- when importing CSV contacts, fill missing FirstName, LastName, DisplayName fields from provided field(s) (RawName, DisplayName, FirstName, LastName)
- when importing CSV calls, fill missing FirstName, LastName, DisplayName fields from provided field(s) (RawName, DisplayName, FirstName, LastName)
- properly handle case where no CR or LF follows last directive in HTML templates
2.1.2.19 - 2009.09.02
- fixed Windows 7 repair problems: changing modem init string to enable caller id and restarting after repair
- trap and log exceptions when saving contact image to temp file; this happened for one user and could conceivably happen if an anti-virus program was scanning an existing file at the time
- updated help for all changes since 2.1.2.0
- fixed call note printing (including when no printer is defined)
2.1.2.18 - 2009.08.27
- autosize images in display panel (to disable, right-click on display panel and remove the checkmark next to "Autosize Images")
2.1.2.17 - 2009.07.29
- added auto import contacts option to delete contacts without phone numbers
- added notification window setting to show the edit buttons
2.1.2.16 - 2009.07.22
- added Copy All command (on right-click menu) to small notification window
- added buttons to small notification window to edit call notes or contact
2.1.2.15 - 2009.07.15
- fixed problems repairing modems which use 64-bit registry entries in Vista 64
2.1.2.14 - 2009.06.28
- fixed a memory leak when the contact view was updated (became apparent when Contact list was visible and enough calls were detected)
- if an unsupported device type is found in the device list (this should only happen if the list becomes corrupt), show a helpful message and continue
- contact window: confirm cancel if changes have been made (and would be lost)
2.1.2.13 - 2009.06.03
- fixed exception when editing action from contacts grid context menu
- added Description, DisplayName (synonym for Name), Title, ContactLabel, ContactNotes, Extra, Email, and CallLabel fields to CallInfo object
- renamed Notes field on CallInfo object to CallNotes
2.1.2.12 - 2009.05.29
- support importing Description field for contacts
- small notification window: added Description field; hide empty fields; shrink width to fit text
2.1.2.11 - 2009.05.21
- fixed "Edit Action" command on Calls list context menu for outgoing calls
- hide "Edit Action" command from Calls list context menu when no call is selected
- hide "Export as Displayed" command from list context menus when list is empty
2.1.2.10 - 2009.05.08
- cache web pages (currently caches last 10 unique pages) — this greatly improves web server performance when database hasn't changed
2.1.2.9 - 2009.04.29
- hide border in large notification window until window is right-clicked for editing
- fixed problem showing missed calls window after it had been closed
- fixed potential problem in large notification window where window size was effectively not saved when maximized
- changed the devices file name extension to avoid anti-virus scans when we access it (should speed up program startup on systems where files with "ini" extension are scanned)
- fixed problem where editing a contact from a call could cause an exception if the window was already open
- added Preview button to Notification options page
- added transparency options to large/custom notification window (transparency is on at about 50% by default; to change this right-click on the notification window when it appears (to bring up notification window on demand, use the Preview button in the Options window, Notifications page))
- in call notes window, don't duplicate company name if company name matches contact name
- when call comes in, deselect existing call before selecting new one
2.1.2.8 - 2009.04.10
- View->Activity Log, View->Network Status, and Help->Diagnostics->Line Monitor now always show the respective windows, instead of toggling their visibility
- backup device settings file before rebuilding
- fixed problem with auto-topmost-fixing in previous version which could cause main window to stay on top of all other windows, including non-ACID windows
- fixed possible exception introduced in 2.1.2.7 when Ascendis Caller ID closes after Options window was opened
2.1.2.7 - 2009.03.31
- use multiple threads when sending real-time network messages to clients, so slow clients don't make other clients wait
- fixed problem where color and font customization windows could be hidden behind large notification window, including a general solution that should find and fix these problems on the fly
2.1.2.6 - 2009.03.26
- more Whozz Calling? tweaks
- fixed extraneous leading spaces for first line of notes on call printout
- size small notification window to fit data
2.1.2.5 - 2009.03.25
- don't create debug file when generating missed calls report
2.1.2.4 - 2009.03.24
- initialize Whozz Calling? device if version info is received when call is expected and device has not already been initialized
- allow more time for Whozz Calling? device to respond
- fixed problem displaying '<' and '>' in display panel
- added HtmlEncode function for scripts
- improved call printout appearance
- added Notes, ServerName, CallLabel fields to CallInfo object
- added header and footer to call and missed calls printouts
- remember page setup (saved when print preview's page setup window is closed using "OK" button; saved separately for different report types)
2.1.2.3 - 2009.03.18
- Call Notes window: fixed print preview problem when ACID is configured to keep child windows on top where print preview sub-window would appear behind call notes window
- missed call report changes: - fixed problem where call appeared as missed if incoming call exceeding max call duration was received after short call from same caller - added Call Duration column - added print
2.1.2.2 - 2009.03.17
- missed call report changes: - remember window size and position - remember report settings (settings are saved when report is built) - fixed line selection - added time period - added auto build option
2.1.2.1 - 2009.03.16
- added missed calls report (View -> Missed Calls)
- track HTTP/TiVo bytes sent and received in Network status window
- added YAC server and client (see < http://www.sunflowerhead.com/software/yac/ > for YAC information)
2.1.1.1 - 2009.02.17
- fixed calls filtering in web pages
- support ACID templates in ".htm" files too (was just ".html")
- fixed content disposition in web pages
2.1.0.38 - 2009.02.09
- updated help for Options/Mail
- connected Options/Advanced page to help
- connected Options/Device/Modems and TAPI page to help
- added help for Skype page of Options window
2.1.0.37 - 2009.01.21
- fixed Address column in Calls list
- assume all Whozz Calling? detail "R" messages are for incoming calls
2.1.0.36 - 2009.01.17
- added "Edit Action" context menu command to call, call report, and contact lists
- added DWS script function "GetComputerName"
2.1.0.35 - 2009.01.03
- for TAPI calls, only use CALLERID data for incoming calls, and only use CALLEDID and CONNECTEDID for outgoing calls
2.1.0.34 - 2008.12.17
- added "Call Began Date", "Call Began Time", "Call Ended Date", "Call Ended Time" as available columns in Calls grid
- added "Export as Displayed" to grid context menus
2.1.0.33 - 2008.12.10
- another printing patch
- ensure preview window is on screen when opened (could have been saved in a position on a monitor that is no longer present)
2.1.0.32 - 2008.12.10
- patch for printing problem reported by one user
- enabled incremental searching in all main window grids and Select window (type to search for row where first column matches typed text; to search using a different column, move that column to the first position)
- when editing display script (using ctrl+right-click on display panel), open in Notepad if "dwshtml" is not mapped to a program
2.1.0.31 - 2008.12.04
- trap and report exceptions when parsing network messages
- added "Phone Notes" field to Calls grid
- added copy-clicked-cell command to grid context menus
- added advanced network client option "Overwrite local contacts"
- added hidden command to edit display script (hold control key while right- clicking on display panel)
- added contact custom fields to CallInfo object
- added Skype support (see Skype subpage in Options window on Devices page)
2.1.0.30 - 2008.10.30
- added option for Whozz Calling? devices to use computer date & time for call timestamps (valuable for Whozz Calling? Lite devices, which only report call time to the minute)
2.1.0.29 - 2008.10.23
- added option to specify starting tab when showing contact window for incoming call notification
- gracefully handle read-only ini settings file
- restore ability to use ini file for settings (broke around 2.1.0.26?)
- fixed rare Delphi exception when hint window might appear when Windows tick counter (GetTickCount) rolls over (which means Windows was running for more than 49.7 days)
2.1.0.28 - 2008.10.13
- added ability to delete labels from call notes window (select label to delete and press delete while list of labels is displayed — labels will not be permanently deleted until you click OK to close the window)
- made label combo box display more labels at one time, and made drop-down resizable
- added ability to sort labels alphabetically in call notes window (check "Sort labels alphabetically" next to labels control)
2.1.0.27 - 2008.10.01
- fixed first ring suppression for some Whozz Calling? devices
- support multiple simultaneous Whozz Calling? Ethernet Link devices (limitations: Whozz Calling? Lite devices are not supported; all devices are treated the same with respect to capabilities (first ring suppression and call blocking will be inconsistent if not all devices support it; no calls will be downloaded from devices with memory); devices must specify unique line numbers (supported on Whozz Calling? 4 and 8)
- fixed potential range check error when waiting for Whozz Calling? 2 devices to erase memory
- fixed Whozz Calling? ring type detection for calls with only one ring
- allow more time for Whozz Calling? devices to respond (improves behavior with Whozz Calling? Ethernet Link devices)
- more informative error message when ELSetup or ELPopup could be preventing communication with Whozz Calling? Ethernet Link devices
- fixed occasional bizarre phone number formatting for ###NUMBER### tag in calls table in HTML pages
- support ###ACTIONNAME### tag in calls table in HTML pages
2.1.0.26 - 2008.09.24
- fixed potential exception when activity log messages are added from secondary threads (introduced in 2.1.0.16)
- trap and ignore EIdConnClosedGracefully exception when disconnecting from mail server
2.1.0.25 - 2008.09.19
- reflect location changes immediately in calls list, display, and actions (note that contact location information still overrides location list data, so calls from known contacts use the contact location info instead, even if empty)
2.1.0.24 - 2008.09.07
- fixed rare problem where null in activity log caused improper extraction of newest entries for technical support report
2.1.0.23 - 2008.09.05
- added ability to open data path from about box (right-click on "Ascendis Software" logo for command)
- added data path to technical support report
2.1.0.22 - 2008.09.03
- polling server: fixed potential hang on program shutdown if client disconnects before receiving all calls
- polling server: send calls in date order so client can pick up where it left off in case of premature disconnection
- use disk tables for polling server queries (may improve memory usage)
- improved accuracy of bytes sent & received reporting in Network Status window
- improved Whozz Calling? handshake reliability when device is slow to respond (could be caused by slow or overloaded computer?)
2.1.0.21 - 2008.08.27
- improved network polling performance when network logging is disabled
- don't log warnings about non-numeric phone numbers with unmatched format rule
2.1.0.20 - 2008.08.12
- added option to keep child windows on top of main window (currently applies to Edit Contact, Edit Call, Find, Activity Log, Line Monitor, Modem Log, Modem Responses, Network Status windows) — see General page of Options window, "Keep child windows on top of main window". The default for this is on, so if you're fond of the previous behavior, uncheck this.
- Options window: if a modem configuration was changed, reopen TAPI AND serial devices (fixes bug where changing modem from TAPI to serial or vice-versa didn't open device until restart)
2.1.0.19 - 2008.07.18
- added call timeout setting to device properties page (access from Devices page in Options window) — increase this if your rings are more than 10 seconds apart (6 is common in the US) or your modem or system is very slow (The disadvantage of a long call timeout is that a new call received within the timeout window will be perceived as the same call, since modems are pretty dumb, call-wise)
- don't cache contact pictures in display panel (so image shown always matches the image in the Edit Contact window)
2.1.0.18 - 2008.07.12
- when repairing database, fix contacts without UID by creating new UID (this should not happen, but has for at least one user)
- fixed problem where wrong phone number was probably used when looking up contact's phone number on the internet
2.1.0.17 - 2008.07.07
- modem repair & direct serial mode: support "DDN" and "DN" as synonyms for "NMBR" (may improve behavior with some Canadian phone companies)
2.1.0.16 - 2008.06.28
- support three TLS options for SMTP authentication (NOTE: users previously using "Secure (TLS) Login" will probably need to change the authentication setting to "Secure Login (Require TLS)" to get mail working again)
2.1.0.15 - 2008.06.27
- ignore Whozz Calling? Ethernet network echoes
2.1.0.14 - 2008.06.20
- log error code when modem responses registry key cannot be opened
2.1.0.13 - 2008.06.17
- install "Empty Database.ciddb" into program folder (this can be restored (File -> Restore Database) to restore database to initial contents, deleting all added calls, contacts, actions, locations, etc.)
- fixed small (normally not detectable) memory leak in Whozz Calling? device support introduced in 2.1.0.11
- replace missing phone records when encountered
2.1.0.12 - 2008.06.10
- trap and report exception when updating phone record for call
2.1.0.11 - 2008.06.06
- modem repair: trap and log problems accessing modem responses
- support Ethernet Link Whozz Calling? devices (select "Ethernet" for port in Whozz Calling? device options)
2.1.0.10 - 2008.05.22
- gracefully handle more undesirable and unusual phone numbers (like '-') (users with such problematic phone numbers will need to repair their database using File -> Repair Database)
2.1.0.9 - 2008.05.14
- don't stop at embedded forms when moving to next or previous window using keystrokes
- changed keystrokes for next and previous window to F6 and SHIFT+F6 or CONTROL+F6 (so now SHIFT+PAGEUP and SHIFT+PAGEDOWN can be used for multi- selections in grids)
- support CTRL+C in main window even when calls or calls report is not showing, or is showing but no call is selected
- support CTRL+C to copy caller phone number in notification windows
- added "Copy Caller Name" and "Copy Caller Number" commands to notification window popup menus
2.1.0.8 - 2008.05.13
- when rebuilding phones table, combine phone records that resolve to same phone number (with respect to MatchNumber)
2.1.0.7 - 2008.05.12
- removed SIP support from general distribution version
2.1.0.6 - 2008.05.09
- Options window: other minor tweaks
- Options window, Edit Contact window: make Action combo boxes taller when screen size allows
- fixed bug where display panel showed last location info for calls with no known location info
- fixed bug in Edit Contact window which prevented manually adding phone numbers (probably introduced in 2.1.0.1)
2.1.0.5 - 2008.05.03
- minor cosmetic adjustments
- added Help for Find Locations, Edit Location
- fixed Find in Locations
2.1.0.4 - 2008.04.28
- better handling of no sound device systems at startup
- support automatically setting options at install time
- support automatically importing xml file at install time
2.1.0.3 - 2008.04.22
- fixed memory leak for polling servers with pre-2.0 call data
- fixed potential exception when receiving pre-2.0 device data via network
- attempted fix for reported exception when drawing call list
2.1.0.2 - 2008.04.17
- added File -> Export -> Auto Export Calls command
- fixed bug in Vista when where wrong file type might be used for exported files
2.1.0.1 - 2008.04.14
- added hidden command "Remap Calls to Phones"
- fixed bug where partial phone numbers from phone device were mapped to existing phone numbers
- don't try to speak on systems with no sound output devices
- fixed crash on startup on systems with no sound devices
- fixed glitch in tray menu that sometimes showed extraneous separators
- workaround some modems under TAPI where a phone number was reported twice for one call, the second time being the caller's name
- option to extract phone numbers from SIP addresses
- store full SIP addresses
2.1.0.0 - 2008.03.26
- fixed Network Status window getting moved from secondary monitor (left of primary monitor) to primary monitor when closed and opened within a session
- removed separate "Log on to incoming mail server before sending mail" option (Options window, Mail page) — set Authentication to "POP3 Login" instead
- added TLS login support for mail server (Options window, Mail page)
- added "Log mail communications to Activity Log" to Options window, Mail page
- fixed EIntOverflow exception on systems w/o sound when running script
- added POP3 port setting to Mail page of Options window
- fixed Repair Modem problem under Vista in certain cases
- moved device settings from registry to ini file
- support loading GIF files for contact images
- keep windows accessible on startup on multiple monitor systems when monitor arrangement has changed
- added preliminary SIP support (not completed yet!)
2.0.1.18 - 2008.01.08
- fixed distinctive ring detection with Whozz Calling? devices
2.0.1.17 - 2008.01.07
- fixed outgoing call number reporting when dialing from within Ascendis Caller ID
- when double-clicking on deleted call in Calls Report list, show message instead of throwing exception
- fixed email column in calls list
2.0.1.16 - 2008.01.03
- added context menu commands to copy email address and send email from calls list, call report, and contacts list
- recompute location from Locations list any time a phone call comes in that has not been assigned to a contact
2.0.1.15 - 2007.12.05
- added "Recompute Phone Statistics From Calls" command to File menu when control key is pressed while bringing down menu
2.0.1.14 - 2007.12.03
- Calls report: fixed "Last Month" and "This Month" filters
- added call count and total duration to calls report (existing users may have to right-click on any column heading and choose "Footer" to make the footer visible)
- when repairing the database, set FirstCalled and LastCalled fields, for phone records where either is empty or zero, to the first or last call, respectively, from the phone number
2.0.1.13 - 2007.11.30
- when repairing database, set FirstCalled field, for phone records where it is empty, to the first call from the phone number
- attempted fix for rare EIniFileExceptions during startup or shutdown
- fixed problem importing calls from XML for phone numbers not assigned to contacts
- display panel: don't show 'from' before company when name is blank
- log message in Activity Log when database file is missing on startup
- fixed record count when exporting entire database
- don't report non-caller id call info events in Activity Log
2.0.1.12 - 2007.09.24
- turned off beta expiration
- when matching TAPI devices to known devices, check TAPI ID and device name first, then device name, then TAPI ID (should improve behavior when an internal device is moved from one card slot to another)
- support ActionTypeName when importing Actions from CSV files
- support importing ActionName, CallsMade, CallsReceived, FirstCalled, LastCalled, RawName in Contacts CSV files
- support exporting Locations files
- prevent duplicate locations
2.0.1.11 - 2007.09.14
- added "Ignore outgoing calls" setting on the Advanced page of the Options window
- when dialing from within Ascendis Caller ID, reflect the dialed number in the outgoing call record
- initialize phone location fields from Location list when relevant
- added Locations tab to main window
2.0.1.10 - 2007.08.20
- added Off Hook Time setting to Advanced Device Properties window
- always enable "Advanced" button on Devices page of Options window
- fixed problem introduced in 2.0.1.9 when running on system with no sound devices
2.0.1.9 - 2007.08.15
- fixed exception when toggling "New" status via grid for call in progress
- fixed potential action-not-found error when adding action and using in contact without restarting Ascendis Caller ID
2.0.1.8 - 2007.08.13
- added advanced script command Volume to change volume for sounds and speech (overrides current volume settings in Options window, except "Disable sound")
2.0.1.7 - 2007.08.09
- workaround improper TAPI configuration when formatting phone numbers
2.0.1.6 - 2007.08.08
- sped up hanging up phone using modem without TAPI
- fixed potential problem when hanging up phone using modem without TAPI
2.0.1.5 - 2007.07.30
- fixed problem preventing proper startup when a faulty or unusually incompatible TAPI device is registered in Windows
2.0.1.4 - 2007.07.27
- fixed problem importing CSV contacts when first name, last name, or company has changed
2.0.1.3 - 2007.07.25
- fixed problem when importing XML contacts from machine with actions with same name but with different unique identifiers (happens if actions are created on two machines, or actions existed from version one and were converted independently)
- fixed help link in Start Menu group
- fixed problem where installing over version 1 with same Start Menu group name would result in no Start Menu group
2.0.1.2 - 2007.07.23
- fixed problem importing XML contacts when same phone numbers were already in the database (symptom: contacts were imported w/o phone numbers)
- when installing over version 1, remove version 1 uninstall entry, desktop icon, and Start Menu items
- fixed potential exception when Windows runs without shutdown for 49.7 days
- fixed potential 'Could not find caller in the contacts list!' message when contact exists
2.0.1.1 - 2007.07.11
- added auto import contacts option to overwrite existing contacts
- added setting to disable new device notification
- fixed New Calls display
2.0.1.0 - 2007.07.09
- first official version 2 release
2.0.0.8 - 2007.07.08
- don't act on outgoing calls received through polling unless configured to do so
- don't include extension in default export names to avoid double extensions
- fixed call list repositioning after polling
- fixed problem automatically restarting for Rebuild Devices List
- support more fields in web server for calls and contacts (see help for list)
- updated help for version 2
- disable Modem Logs submenu item when modem log is not accessible (as is common in Vista)
- Options window: fixed help link for Advanced page
- implemented Help in Find windows
- Find Contacts window: improved behavior
- corrected modem analysis with respect to '"EnableCallerID\1" setting value'
- added Help button to Select Contact window
- implemented Help button in Edit Call window
- replaced "Raw Line" column in Find Calls window with "Device Name"
- fixed Delete Duplicate Calls command
- fixed problem where "Test Action" command for recent calls might be disabled
- change default mail server authentication to "Simple Login"
- fixed control drawing bug under Vista when ALT is pressed
2.0.0.7 - 2007.06.29
- fixed beta expiration problem with systems using non-US date formats
- Select Contact window: added name column; sort by name column initially
- restart Ascendis Caller ID automatically when stopped for repairing database
2.0.0.6 - 2007.06.27
- fixed possible exception when Windows shuts down while Ascendis Caller ID is running
- fixed some update issues when adding or editing patterns or deleting contacts with patterns
- allow image files to dropped onto the Picture area in the Contacts window
- added {CountryCode}, {Prefix}, {Suffix}, and {More} to macros for URLs and easy actions, and to CallInfo in advanced actions
- fixed autolaunch for Vista
- detect and prevent multiple instances including separate user sessions
- removed grouping options in grids
- allow cancel in Auto Import window after specifying invalid import file or folder
- fixed exception when auto import folder could not be found
- put program icon on common user desktop so it's visible by all users
- fixed modem repair under Vista for standard users
- multiple TiVo server fixes
2.0.0.5 - 2007.06.13
- when importing contacts from CSV files, properly handle address info for display in Calls list
- added option to automatically delete calls based on number of calls or call age (see Advanced page in Options window)
- reorganized some options in Options window from General and Search pages to General and Advanced pages
- when looking for phone number from network server, check MatchNumber column if RawNumber column isn't found (fixes possible EDBISAMEngineError / DBISAM Engine Error # 9729)
- fixed bug where adding new phone number to an existing contact in "Edit Contact" window would not propagate address information ("Repair Database" corrects this for existing contacts)
- fixed problem where using shortcut from Calls context menu prevented calls list from being scrolled to new call when call came in
- installer can now add exclusion to Windows firewall (default = open firewall)
- corrected location of some program settings in registry
2.0.0.4 - 2007.05.30
- fixed potential exception when deleting call, call report, contact, or action items with delete key
- make auto startup work for current user only to avoid fast user switching problems (will not affect users who only use one user account; for others, turn on auto startup for account that is logged in first)
- fixed some problems repairing and restoring modem settings on Windows Vista
- Edit Contact window: trap and report errors when loading jpeg images
- (code) sign application and installer
- don't automatically enable new devices when no longer in trial mode (fixes bug where adding a new TAPI device could disable an enabled Whozz Calling? line)
- position calls list on newest call after getting new calls via polling
- fixed problem polling version 1.x network servers
2.0.0.3 - 2007.05.18
- when importing contacts from CSV, only warn once about blank phone numbers
- when looking for phone number, check RawNumber column if MatchNumber isn't found (may fix EDBISAMEngineError / DBISAM Engine Error # 9729 reported by a user)
2.0.0.2 - 2007.05.17
- improved Repair Modem compatibility with Windows Vista
- refresh Phones table before looking up incoming phone number (may fix EDBISAMEngineError / DBISAM Engine Error # 9729 reported by a user)
- refresh Calls table before updating with call progress (may fix EDBISAMEngineError / DBISAM Engine Error # 8708 reported by a user)
- don't try to repair modems accessed directly, since it isn't necessary and is only relevant for TAPI access
- fixed exception during startup when modem was added while trial screen was displayed
- update new call count after deleting calls
- don't show date and time in display panel when calls list is empty
- renamed "NewCalls" DWS script function to "NewCallCount"
- added "CallCount" function to DWS scripts
- added full text search and favorites to Help
- properly start up when launched while another instance is shutting down
2.0.0.1 - 2007.05.10
- made display panel / toolbar splitter slightly more visible
- reposition contact list after editing contact
- added Speak Name macro to easy action macro popup
- don't show error when importing very old version 1 databases
- some help updates
- raised price in trial mode screens
2.0.0.0 - 2007.05.07
- fixed help links in Help menu
- support bold and italic font styles in display
- don't automatically repair databases on new installs
- create calls report initially without logging exception
- ignore v1 trial period (start new trial period for v2)
- when importing version 1 settings and data, import registration files too
1.9.123.0 - 2007.05.02
- fixed possible unregistration of licenses when multiple instances of Ascendis Caller ID were started at the same time
- fixed possible device list overwrite when multiple instances of Ascendis Caller ID were started at the same time
1.9.122.0 - 2007.05.01
- fixed non-fatal error after installing version 2 over version 1 and restarting machine
- new application icon
- auto import callers window: don't accept empty filename when auto import is enabled (fixes exception in this case under Windows XP)
- refresh database after changing contact and before displaying selected call so latest changes are reflected
1.9.121.0 - 2007.04.23
- log message when network call is ignored because it originated on the same machine
- use dwshtml file for display panel
- if version 1 database is found in database folder, offer to upgrade it
1.9.120.0 - 2007.04.18
- fixed problem with customized call list fonts and highlighted text
- automatically reposition calls grid on new call unless user is using it (such as clicking or pressing a key in last 10 seconds)
1.9.119.0 - 2007.04.17
- fixed potential exception when changing small call display window position on machines with limited user rights
- added ability to select font for incoming and outgoing calls (right-click on Calls or Calls Report list and select "Appearance")
- removed "Hide server name" option from General page of Options window (obsolete)
- show outgoing calls in gray in Calls list
- added (currently hidden) command to import version 1 database (hold down SHIFT when opening Import menu) (normally conversion is performed when version 2 is first run)
- fixed bug when importing CSV calls where calls from existing numbers could be mistaken as duplicates
- support importing CSV calls files exported from version 1
- hid some currently unsupported columns in Find windows
1.9.118.0 - 2007.04.10
- fixed problem with Auto Import Callers when running under limited user
- fixed problem with dbisam.lck files appearing on desktop
- hid some currently unsupported columns
- corrected spacing on column names
- increased default main window width to 800
- when importing from version 1, make sure raw phone numbers are truly raw
- check for valid TAPI configuration on startup and prompt if not valid
- improved shutdown behavior when database files are missing
- added "Contact Label" column to Calls and Calls Report lists
- show progress when deleting multiple calls from Calls list
1.9.117.0 - 2007.03.21
- don't log encrypted network messages (for polling when network logging is on)
- Send Call To Network Clients: don't require "Forward network calls to network clients" setting
- fixed problems where wrong device could be referenced if it had the same name as a device on different machine (this could cause incorrect device descriptions)
- added AddressID to CallInfo object
- fixed potential problem where Whozz Calling? device from network server could be mistaken as locally connected Whozz Calling? device
- don't send outgoing calls to version 1 network clients
- fix problem with app freezing when restoring from tray icon
- show correct line description in notification popup
- include script name in script error messages
1.9.116.0 - 2007.03.18
- disable Rebuild Calls Report button while rebuild is in progress
- when automatically reconnecting to real-time network server after connection was lost, perform polling first if so configured
- pass device information for calls to network clients (so device descriptions are correct)
- added ability to restrict network clients by IP address for Whozz Calling? devices
1.9.115.0 - 2007.03.03
- fixed several context menu commands on calls report after calls have been deleted
- update contacts list after adding number to existing contact
- added Rebuild Calls Report button to Items toolbar when appropriate
- right-clicking on list backgrounds now brings up context menus (previously had to click on data cell, which was impossible when list was empty)
- added ability to restore database when dropped on Ascendis Caller ID icon when Ascendis Caller ID is not running
- improved real-time network support (now sends complete phone record to compatible clients (was already sending phone number))
- added client setting for Whozz Calling? devices to restrict clients
1.9.114.0 - 2007.02.27
- when exporting calls, use hh:mm:ss format for CallDuration
- fixed progress when exporting calls
- added efCSV, efHTML, efText to DWS scripts to replace CSV, HTML, TEXT
- added elCalls to DWS scripts to replace CALLS
- added elActions, elContacts to DWS scripts to replace CATEGORIES and CALLERS
- fixed HTML and text export
1.9.113.0 - 2007.02.22
- Find Calls, Find Contacts: added relative date filters; ignore time when filtering; don't open item when double clicking on header
1.9.112.0 - 2007.02.20
- added sums of Calls Made and Calls Received to Find Contacts window
- added sums for Call Duration and Rings to Find Calls window
- fixed problem where zero Call Duration was usually displayed as a time in Find Calls window
- improved percent escaping in Find
- added Beginning and Ending options to Find
1.9.111.0 - 2007.02.19
- added Complete and Partial options to Find
- added Find Actions
- fixed incorrect # of total contacts in status of Find Contacts window
- improved progress responsiveness in Find Calls window
- let Escape close Find windows
- fixed tab order in Find windows
1.9.110.0 - 2007.02.16
- fixed Find button in Find Calls (wasn't working)
- changed shortcut for Find to Ctrl+F
1.9.109.0 - 2007.02.15
- added Find Contacts command
- added Find Calls command
- added insert status to Script window status panel
- added actions Show in Outlook, Show or Add in Outlook, Show in Outlook and add appointment, Show in Outlook or add appointment
- display action name in Script window caption
- made DWS function VarIsEmpty act like it did in Ascendis Caller ID version 1 (in that 'nil' values return True)
- added database info to Technical Support Report
- fixed polling
- fixed import calls (for v2)
1.9.108.0 - 2007.01.26
- don't create debug SQL files
1.9.107.0 - 2007.01.25
- added "Standard Actions.xml" to install instead of "Standard Categories.csv"
- added "Do Nothing", "Block", "Block and Announce" to default actions
- fixed problem retrieving (display) name and speak name from contacts
- pass contact record to real-time network clients (pictures > ~20k will not be included)
- improved real-time network support
- don't log messages that repeated within 1 day (previously was 2 minutes)
1.9.106.0 - 2007.01.23
- added Company to main display
- added "Move Number to Different Contact" command to Contact list right-click menu
- added network support for outgoing calls
- added Company to notification tray pop-up
- added Company and address to small notification window
1.9.105.0 - 2007.01.20
- added Company and location info to tray notification and small notification window
- added Company, CallBegan, CallEnded, Address, City, County, State, PostalCode, Country to macros and CallInfo
1.9.104.0 - 2007.01.17
- fixed network call sending
- added "Print Calls Report" to File menu
- disable "Print Calls" when calls list is empty
- disable "Print Call" when calls list is empty or > 1 call is selected
1.9.103.0 - 2007.01.12
- when importing contacts, merge new and existing contacts when first name, last name, and company match
- when importing contacts, adopt existing phone numbers
- don't warn about empty ring or outgoing call action names
- support editing call notes while calls is in progress
- give meaningful error message when user tries to create Action with same name as existing action
- fixed Company column in Calls list
1.9.102.0 - 2007.01.05
- don't create RebuildPhonesQuery.sql file
- support multiple phones per contact when importing from CSV files
- export phone numbers and actions with contacts when exporting to XML
- don't warn when editing contact with no phone numbers (was logged previously)
- support relative web root setting (relative to data folder)
- fixed export hints
- default to exporting and importing in XML
1.9.101.0 - 2006.12.28
- added '-settings
- fixed ini file settings use
- fixed potential exception when creating new action
- support outgoing call actions
- fixed "Send call to network clients" command
- when importing v1 settings, correct network log file path for v2
- fixed filtering wildcards
- allow LIKE filter operator
- fixed pattern matching problems
- Options window, Mail page: always show advanced mail settings
- fixed problem with proper Whozz Calling? device detection and configuration when Whozz Calling? 2 is clearing memory while program is starting
- fixed call start time reporting for Whozz Calling? devices when devices that pick up and hang up phone (to check for messages) are present
- added "Item Toolbar" to View menu
- added images for Item toolbar
- remember Item toolbar visibility
- use most recent raw name for callers not in contact list
- performance improvements
- fixed optional comma in display panel
1.9.100.0 - 2006.12.04
- added Duration column to Calls and Calls Report
- mark call report dirty when a call is deleted from calls list
- track number of rings for (non-Lite) Whozz Calling? devices
- set time of (non-Lite) Whozz Calling? devices
- made small and large notification windows come to top without stealing focus
- fixed small and large notification windows
1.9.99.0 - 2006.10.13
- remember Select Contacts window placement
- removed unused columns from Select Contacts window
- fixed many right-click actions in grids
- disable Test Action for outgoing calls
- fixed TiVo call images
- fixed Mark as Read/Mark as New
- added lookup-style Calls grid (for speed)
- renamed old Calls grid to Calls Report
1.9.98.0 - 2006.09.22
- fixed "Look Up Phone Number" on Contacts grid right-click menu
- fixed "Copy Contact Name" on Contacts grid right-click menu
- fixed "Copy Contact Number" on Contacts grid right-click menu
Friday, 15 April 2011
KUMPULAN CD KEY
WinMe QC63F-M7VVT-CQXVP-89CFX-K8WHD
WinMe 4.90.3000 español: 53336-335-0355186-14795.
WinMe 4.90.3000 español: 52782-oem-0000563-26947.
WinMe 4.90.3000 inglés: 52783-012-3415591-14184.
WinMe RBDC9-VTRC8-D7972-J97JY-PRVMG
WinMe h6twq-tqqm8-hxjyg-d69f7-r84vm
WinMe vxkc4-2b3yf-w9mfk-qb3db-9y7mb
WinMe C9TCH-G72Y6-G4DQK-QCQRM-K7XFQ
Windows ME Full Retail: Gy9fq-2j9mr-pm78b-j9jct-x8rdg
Windows ME Product key code upgrade 98 s/n:
FYG4R-3RK8M-DJGPJ-9GTRY-Q7Q49
Windows 95 OEM Version : 11195-OEM-0000043-11111
Microsoft Windows 98 SE : J4BBX-CBRW9-7J4RG-TPY7V-32WPW
win2000 : yc93-y8ck8ly-eppnby8-yvnx
Windows XP Home OEM PL : FMMRG-VT4HV-96GVK-6JFQT-VTH7T
Windows XP Professional PL : FCKGW-RHQQ2-YXRKT-8TG6W-2B7Q
3D Studio Max 4.0 : s/n 226-19791979 key XLSQBQ
Adobe After Effects 5.5 : EWW470R1001999-030-259
Adobe livemotion 1.0 : LVW100R7100843-292
Adobe photoshop 6.0 : PWW600R7105467-948
Adobe golive 5.0 : GJW500R2898273-460
Adobe illustrator 9.0 : ABW900R71111141-999-830
Adobe premiere 6.0 : MBW600R7100765-881
Adobe photoshop 6.0 : PWW600R7105467-948
Adobe Photoshop 8 - serial: EWW470R1001999-030-259
Ad-aware Professional - password: 1291453
Advanced Office XP Password Rec. Pro 2.0 AOXPPR-PRO-TADFH-43369-HTHAF-42745
Advanced Paradox Password Recovery 1.01 MSPP-83852-DWMRA-762
Advanced PDF Password Recovery 1.34 PDFP-99322-EYSVY-886
AlamDV Name: KHS Inc. | s/n: dqonbaobazyxwvut
AlamDV 2.01 name: Claudio Duffini | s/n: bspdopxmaziansxm
Alcohol 120% v1.3.1.823 s/n:
AL12PPPPAFAE4rneMMz
Analityk 1.00 System Wspomagania Dec.* PL* wAo6xQ-ontcA8-QpGEii-v24iSE
Animation Masters 1234-123
Anim-FX 5643er-2158719ptr87
Applet Button Factory 5.1 Name: 12aew | s/n: 9j8f5
Aqua 3D screensever 1.51 Name: DARRYL WILSON | s/n: FLDP-MXMS5MBFBV3T-JBTT
Aquatica Waterworlds 3.xx Name: Wanlop L. | s/n: TMOPZ-KWVZA-SEIQO-JYGXA
Ashampoo AudioCD MP3 Studio 2000 2.50 Code:CSN77B5AA0B54N0326
AtomixMP3 v2.2 9KP-P45-MFC
Audio Record Wizard 3.2 Name:overdays.net | Code:17CD-452C-9FD3-2441
AutoCad 2000 *PL * Serial: 112-11111111 | CD-key: 5x8NUG
AutoCad 2000i s/n 999-12345678 | CDkey 94ED61
AutoCad LT 2002 *PL * s/n: 400-12345678 | CD-key: T4ED6P
AutoCad architekt. desktop 2i : 112-11111111 cdkey: 5x8nug
AutoCadarchitekt. desktop r3 : 400-19791979 cdkey: tlsqbs
AutoCadmechanical desktop r3 : 111-91236432 cdkey: 263a
AutoDesk inventor 4 : 456-7890-1234 cdkey: 8qpm2m : 202-12345678 cdkey: g4ed6p
Backup DVD 8.4 s/n: ASRO2-IFHT1-AWNA9-TRE89 | s/n: FIA89-TERA3-SQF26-ANW71
Banner Maker Pro 3.0.3.2 Name : MackOi DBC | Serial : 3122545210621
Banner Maker Pro 3.0.3.3 Serial: 3122545210621
Befaster 2.7x name: Mister Stop | s/n: 2838949334
Big Scale Racing ED66-6866-1766-2A66
BiznesPlan 2003 s/n: BPD6203828218
BlindWrite Suite 4.2.5 s/n: 5LFG - ENPV - EI5W - 5LFJ | Code: YRB1F1J1YDYU7MEJ
BlindWrite Suite 4.2.7 Code:5LFG-ENPV-EI5W-5LFJ
BlindWrite Suite 4.2.9 Code:5LFG-ENPV-EI5W-5LFJ
BlindWrite Suite 4.3.0.31 YRB1F1J1YDYU7MEJ
Borland C++ Developers Kit 5.0 BDS1350WW10185
Borland C++ Builder 4 s/n: 100-000-0427 | key: xcx8-19x0
Borland C++ Builder 5.0 s/n: 111-111-1111 | key: 4eb1-2301 go
back and enter 4eb 230
Borland Delphi 4.0 C/S s/n: 123-123-1234 8ex2-5fx0
Borland Delphi 6.0 Enterprise serial: z9j8-pum4n-c6gzq | key: rw2-7jw
Borland JBuilder Pro 2.01 s/n: 1000000171 key: c3-7-0-0
Borland JBuilder 3.5 Pro s/n: xa33-?2xtt-rpvb9 | key: 5p7-?rz
Borland JBuilder 4 serial: xa22-?hrs5-2ubgs | key f2j-46g
Borland JBuilder 5.0 Enterprise serial: xa52-?npkv-gcqzw | key: m69-tet
Bryce 4 BF00WBB-0000000-CUY
Borland c++ builder 5.0 : 200-000-0360-3fx8-23x0 bryce 4 : bf00wbb-0000000-cuy
Cakewalk Music Creator 2002 CWMC102-03754
Cakewalk Pro Audio 9.0 s/n: cwpa900000000 | key: 7009001015988
Caligari Truespace 6.0 s/n: 8040701010404
Camedia Master 4.0 s/n: zcm1-400e-2009-6083-08mk
Catz, Your Computer Pet s/n: 1287-6097-3288
CD Autorun creator 1.0.9 91736941951541
CD-Bremse 1.08-1.12 user:hacekw | serial:26EE51FCBDB6
CD Mate Deluxe 2.2.2.5 name: TEAM CAT 2002 | s/n: CMAA0EA775TAA
CD Mate Deluxe 2.2.8.1 Name: Oxide Owns |
Company: Oxide | s/n: CMNC8DOC810F7
CD Mate Deluxe 2.2.11.19 Name: TEAM CAT 2002 | s/n: CMAA0EA775TAA
CDRLabel 6 Line1: 35786784 | Line2: 186844707
CDRoller 4.20 Name: NeRo | s/n: 0071-3812-5781-3881-1791
Cdrwin 3.9c Your Name: Mark Brown |
Company/Email: Power Shells | Unlock Key: 960693AE-138F1499-7E990C80-08505467 |
Check Key: 9E56C7C9-85898737-6D161819-76C958E7
Cdrwin 5.05.001 50R0R-CDR22-48362-76423-71087
CDSpace 4.1 cd-key: SF5782-4577-216131
C-GEO LT s/n: 77996
Chameleon Clock 2.51 Code: PW-LOCK-6YJA-LU3T-PN2ZK
CheckIt Suite 6.5 TC-025045
Chem Office 2000 Ultra serial: 199920000 | keycode: FRKNLGMPT
Cinema 4D s/n: 16060101169-54A8C4
Cinema 4D Demo 16060101169-54A8C4
Clasp 2000 3.0.3 name: Chafe/TEX99 | s/n: 418333-128331EX99-3623322
CloneCD 4.0 name: Randal Dudley Dudley |s/n: 1619103-1-0045531653-3485491080
CloneCD 4.0.0.0 beta 30 1619103-1-0045531653-3485491080
CloneCD 4.0.1.10 1503807831-2-2247829181- 1489782676
CloneCD 4.0.1.3 1528916631-2-2225435462-1603894703
CloneCD 4.1.0.1 name: JUSTiC | s/n: 2276405-1-2819017008-1043966198
CloneCD 4.2.0.2 1124216600-2-1325733709-1652003845
Collins (slownik PL-ANG) Z00-A400455 | Y61-A300661
Collins 2.0 (slownik PL-ANG) Y11-A217482
Contractus 15-7e19-1-8f-8d5c
Cool Edit 2000 v1.0 Name: MFD Corp. | s/n: Q17EXF5U
Cool Edit Pro 2.0 Name: Peter Quistgard | s/n: 200-00-37YQOQ7L
CoolIE 2.0 Name: TEAM LAXiTY | s/n: vqvOfKTyvmnuTiDibSXG
CopyDVD 6.0 Username: dbrain | e-mail: tsrh@tsrh.lol | regcode: 6M6RBCTSKKHYH6EDBUAO
Copy DVD to CD-R 6.01 s/n: 6MRBCTSKKHYH6EDBUAO
Corel Bryce 5.0 BF50CRD-1257022-WDB
Corel Custom Photo SE 1.0 ccp-psfownz
Corel Draw 9.0 456-1134-1987 | DR9NR-168470W025
Corel Draw 10 English D10NR-3284253T76Corel Draw Graphics Suite 11 DR11CRD-0012082-DGW
Corel Graphics Suite 11 DR11CRD-0012082-DGW
Corel KnockOut 1.5 1004304309
Corel Painter 7 PF70CRD-2564458-SZH
Corel Print Office 2000 P02NR-F721127808
Corel WordPerfect Office 2000 Wp911-8675309PHX
Corel WordPerfect Office 2000 Professional PW9-8Q45777988
Corel Xara 3.04 1992-7866-5926
Customizer XP 1.8.5 Serial: 28031979-ph-17081945
CuteFTP Pro 3.0 AGDWS2M7P5L88E | ACHWS3M795LG8E
CuteFTP XP 5 build 50.6.10.2 s/n: ACBBY2TH79J9C3
CuteFTP 5.0.1 XP A9675644L45JJB
Cyberlink PowerDirector Pro 2.5 s/n: RR99852984H45264
CyberLink PowerDVD XP Deluxe Edition 4.0.1329 s/n: DX32812592653746
CyberLink PowerVCR II v3.0 Deluxe PD7000EPS0000453
Czarodziej Faktur 2.0 CF20-11111111-21092184-11111
Cakewalk pro audio 9 : cwpa900000000 cdkey: 700-900101-5988
Canvas 7 : W181+AICSZ1035033
Corel Draw 10 : D10NR-3284253T76
Collins 2 : X15-A520580 / nocdcode: URSSQI / lis: PSRSUXYU
Delphi 5.5 : 111-111-1111 cdkey: fex1-91x0
Design pro 2000 : dpeur-3102397-d293717-v
Deutsher translator : tCiu-xr3m-fiFJ-QNQ7
English translator 2000 : 945-bd5-a0-caad-e65f
Euro plus + reward millunnium : RP3-12870-kzK!-PL
Finale 2001 : wfnr112460
Hot metal 6 : SQMMO 00D7A 04773
Indesign v1.0 : IPE100R99999999-364
Internet translator : 42eb-504c-4c91-08
Internet translator : 42EB-50C4-4C91-08
Macromedia fireworks 3.0 : FWW300-54034-00155-66943
Macromedia fireworks 4.0 : FWW400-02666-67248-02548
Macromedia flash 4.0 : FLW400-16664-27865-12345
Macromedia flash 5.0 : FLW500-06844-47238-93689
Macromedia dreamweaver 4 : DWW400-03771-57289-73501
Macromedia director 8.0 : WDW800-00276-47200-20442
Macromedia freehand 9.0 :FHW900-00010-47278-88947
Mixman dj : 321-4751-09291
Microsoft Office 2000 PL - T6T4H-H894B-HC382-J8RVY-8CPQ6 lub GC6J3-GTQ62-FP876-94FBR-D3DX8
Mks_Vir.2003 Polish Retail v2.0 - stare seriale: IYEZ-2GPN-AE36-KA65-DIHS, X2VA-86R1-JGUA-HFH7-27N7, E72H-H5N6-79U7-NNAE-PXTW, EE9G-7WSH-GPFU-TKUE-Y6B8, V5IH-7FSC-CN1P-F5J5-E128, HFYN-WA22-JH75-1KLE-AL3U Nowe seriale: 6918-KIRI-K2PY-AIX2-S6NW
Office XP Professional PL - DTVWV-JWTRD-VVJD8-K3WYH-V7B4D
Partition magic 6 : PW600-0028414588
Partition Magic 8.0 - serial: PM800ENSP1-1111113
Portfolio v5.0 : FFE-500-123-778-123123
PowerDVD 2.55 (retail release) - serial: DV3X417M28947802
PowerDVD 5 Deluxe - CD-Key : DX88183R73239812 SN : 10000360
Solid works 2001 : 9940-0102-0101-0465 cdkey : wmc0
Sonic foundry ACID PRO v2.0 ACID PRO 2.0 Serial Number: 76-RZLQ1Z-YEBPJQ-4ECDN3
MP3 Plug-In Serial Number: BM-PB4QYM-D9K51Z-Z4PVWN
Sonic video : 9h-rbxsqt-ye7gex-nqw56x
Statistica 5.5 pl : ngn51q55npn555
Steinberg mastering : 59-27041
Slead video studio 5.0 : 11102-85000-00015330
Wavelab 3.0 win9x : yc93-y8hy6uy-eppnby8-yku9
Windows 95 OEM Version : 11195-OEM-0000043-11111
Microsoft Windows 98 SE : J4BBX-CBRW9-7J4RG-TPY7V-32WPW
Win2000 : yc93-y8ck8ly-eppnby8-yvnx
Windows XP Home OEM PL : FMMRG-VT4HV-96GVK-6JFQT-VTH7T
Windows XP Professional PL : FCKGW-RHQQ2-YXRKT-8TG6W-2B7Q
Airport 2000 : a2s0037865899
American mcgee's alice : 2000-0112900-0010978-3379
Amsterdam schipchol 2000 : 6836-0462c1
Aqua Nox : 5005-4DB8-65F3-FDCF
Battlefield : 1942 5000-0000000-0000000-1318
Black & White : 0901-3324366-4702210-2081
clive barker's undying : 2500-0911911-0911911-2705
Command and Conquer - serial: 4963-7882913-5984076-0674
Command and Conquer : Red Alert 2 020736-428526-011875-6507
C&C Tiberian Sun : 999999-999999-999999-4190 LUB 001979-000000-001999-1106
C&C Gernerals - serial: 575-1454-8742-1243
Dark reign 2 : gad6-teb4-cup9-pap6-5529
Delta Force Task force Dagger : 72YL-R4SB-GKG6-3WKS-HQ27
Delta force 3 land warrior : n5e3-yxh2-g983-9wyb-b3zs
Delta Force - Black Hawk Down - serial: QZP3-EPZW-SP5N-2XEX-H7JG
Devastation : 5B36F-976AA-6A471-58B8D-92B7B
Diablo 2 : Lord of Destruction : 6PNP-D7PG-HZ8M-PZNP
Emperor : Battle for Dune : 036642-256734-246754-5204
Fifa 2003 : DEVI-ANCE-RULE-ZF79
Gunman chronicles : 2609-57245-0368
Half-Life : 4288-32888-8846 lub 1358-88426-7319 lub 5597-15616-9878 lub 7488-31479-5805 lub 1374653317652fuul
Half-Life Counter-Strike : 2462-92319-7642 lub 6194-46803-2697 lub 0412-34698-2864 lub 6629-82570-9849
Harry Potter And The Chamber Of Secrets ! shit:-\ !: 1740-9488245-5171152-5225
Homeworld 2 - serial: NAS3-DEC2-BYJ5-CUJ6-8385
Insane : ntne-eed8-wg2a-76mw-a4
Kingdom under fire : e1kj-rl62-cOO7-ft96
Kohan: immortal sovereigns : 6BD9-9A56-BC85-455A
Medal of Honor Allied Assault : 5000-0000000-0000000-5068
Maden 2000 : 1500-6610360-1643530-0243
Madden NFL 2001 : 1500 6610360 1643530 0243
Madden NFL 2002 : 0901-5445152-2745753-4827
Moto Racer 3 : 0d08-d667-7323-303d-b4ab-8b9d-2597-8350-2051
Nascar 4 : gac7-reb8-tux6-dac2-7833
Nascar Thunder : NASC-ARTH-UNDE-RJAM
NBA Live 2001 : 2001-0020601-0010978-6694
NBA Live 2003 : GMQK-SMMV-Y3QV-GR66
Need For Speed: Hot Pursuit 2 - 8249-7EE3-84EW-TXET
Need For Speed Underground - serial: ZAW2-TYS2-REW2-FAS2-2852, RYN5-FEX7-NUN9-RET3-9879, NEL5-FAJ5-GEC3-FUB2-4899, DEZ4-SEF3-RUR9-ZYB5-4247, XAN9-FAW7-RYG9-RUB5-8473, RES2-TUG7-RYD5-RUR8-8429, TYF5-BAF6-DYX4-MUM5-3273
Neverhood : 54767-442-2533967-82003
NeverWinter Nights : QFETM-MPU3X-DN6FF-MHFDA-YWARA-4HMPE-RDJTG
NHL 2002 : 1000-2003004-0000000-4904
Operation Flashpoint : 1111-11111-11111-11111-11111 lub 7D6P-M4XJG-JATKE-EZLKD-J8M07
Advent.pinball forgotten island: 0901-3066676-3327010-9227
Quake 3 team arena : tsbh-7ccg-dpwp-b2lt-84
Sacrifice : 5pwn-6dup-42yb-16f2
Settlers 4 :0917-1079-8663-8038-7447
Sid Meier's SimGolf : 5000-0000000-0000000-5071
Sim Coaster : 2001-0013101-0010978-2823
Sims : 100486-585530-905808-0928
Sims Balanga : 0901-4177371-2064872-3510
Sims Randka : 4501-8861690-6901571-5555
Soldier of fortune pl : BEN2-BAC7-BUZ6-JAD3-9742
Soldier of Fortune linux version : BEN6-MUC8-BEZ6-BUJ6-3764
Soldier of Fortune 2 Double Helix : 3Z4J-J3PP-K848-EWPK-1F
Startrek starfleeet command 2 : rys8-lab4-jef8-bym3-4652
Submarine Titans : gaymadboy-7teh
Sub Command : 0901-1315206-2102812-3248
Superbike 2001 : 1500-4288423-2982915-3163
Swat 3 : bac2-bab2-bab2-bab2-2352
Swat 3 elite edition : LYF7-FUB6-JEM5-NUD6-5854
Tiger Woods Pga Tpour 2001 Golf : 1500-3202255-2068109-2932
Triple Play 2001 : 14103 BUSY ETCH JOWL TELL
Trophy bass 4 : nub9-wub8-zuc7-raj3-3976
Unreal Tournament 2003 : 9ZNDP-8TDWG-BA8HF-X2D4D
Warcraft 2 Battle Net Edition : 6696-2TGV-TT64-CNMV
Warcraft 3 : DP5ESN-XGQO-VK4UJT-JM71DWHPCJ
Wolfenstein Return to Castle : CLAL-A7WJ-DTSJ-WARP-88
Vampire Redemption - RAC2-SAL2-GAT3-RAD3-654
#1 DVD Audio Ripper 1.0.33 Name: TEAM ViRiLiTY s/n: 90C84A01-5A123607-2FA15BF0-A89D9482-B3AD13F5-E5F57562-A7C869E3-A6EF5C57 http://www.dvdtox.com
#1 DVD Ripper (SE) 1.3.40 Name: tDS Crew s/n: 30872895-339863D1-F9C7A927-C8363D4E-DB435D1F-84331102-C5445F70-8F9C5436 http://www.dvdtox.com
007 MP3 Sound Recorder 1.2 S/N: 56090-546225C16A83 SN: 60C48-E4E225D17A83 SN: 4C3CC-F4C265F12A83 By Alkavour (05/01/2006) http://www.007-dvd.com/download/mp3007.exe
1-More PhotoCalendar 1.80 Name: CRUDE S/N: 2509-8079-4200 mail:crude@scene.org By Alkavour (14/01/2006) http://www.1-more-photocalendar.com/
1stclass Professional 4000 S/N: 1st4000982940nmw (null)
24x7 Automation Suite 3.4.24 s/n: 07691-5914730-07691 http://www.softtreetech.com
2Flyer Screensaver Builder Pro 7.4 S/N: cByeigeGobOEPN9m reg no.:006300086440 By Alkavour (24/01/2006) http://www.2flyer.com/
3D Canyon Flight Screensaver 2.0 S/N: Name: Karen Buttram S/N: ZNDR-Q5MKVWC2CQA5-MNZX name: teabag master code: CMRX-5EBUYUTQYT4J-SNAA Type in registration. Do NOT copy and paste. http://www.digimindsoft.com
3D Flash Animator 4 Release 5 4 Release 5 S/N: 11718363936 http://www.3dfa.com/
3D Mark 1.0.2 S/N: 3DM06-EQF5A-HFTZC-EMX03-WVYR7-HSP65 or 3DM06-LUZ4X-6KSDC-Y0XF9-Q27K0-CPVNR (null)
3D MP3 Sound Recorder 3.8.12 Name: Beatrix Kiddo S/N: register with this name:Beatrix Kiddo_1M2 serial:MFJ5RK-S3I97I-AF7KDD-REHKKM-VCIFJW-8QEOF2 By Alkavour (10/01/2006) http://www.tongsoft.com
3D MP3 Sound Recorder 3.9 Name: THOR_MESTER_673 S/N: 9KB6PT-OCUUW4-EF4FG9-LLTVC5-4BCIHH-YLJ9PS by____THOR_MESTER http://www.tongsoft.com
3DMaek 2006 v 1.0.2 S/N: 3DM06-0FCET-YKWY4-6Y9DE-80HVQ-HLF89 i've got this one from the FFF keygen. http://www.futuremark.com
3DMark06 v. 102 S/N: 3DM06-EQF5A-HFTZC-EMX03-WVYR7-HSP65 http://www.futuremark.com
3DMark06 v1.0.2 S/N: 3DM06-B9T6R-R46UX-8E0FV-DWQQ5-0Y4MJ 3DM06-L5T3U-Q2JQ2-N2A2F-4JNUJ-C2CC7 3DM06-KXYE8-WMJYP-RNX5V-23MZD-8SL8M 3DM06-EQF5A-HFTZC-EMX03-WVYR7-HSP65 3DM06-LUZ4X-6KSDC-Y0XF9-Q27K0-CPVNR http://www.futuremark.com
3gp converter 2.1.46.609b S/N: IUSGZYO64JCH5YM2BLIABD70-3832-59A7-98FD (null)
3GP to GIF JPEG Converter 1.0 s/n: 3gp20-0927 http://www.avimpeg.net
602Print Pack 5.0.05.1114 Name: the unlokcer S/N: CP5CME-STL4YM-999-0X8DZGVFX http://www.software602.com/
7 Download Services 3.2.5 s/n: 6262-6457-6652-6854-7049-7244 http://www.smrksoft.com
8signs Firewall Remote Administration Tool 2.3 S/N: 4400-3DXM-29XM-L3PR sn:4467-187M-DR45-R3HE sn:4426-4TB6-3YHR-AFGB By Alkavour (15/01/2006) http://www.8signs.com/
8Signs Firewall 2.3 S/N: 4452-23CY-LFPY-7X52 sn:4403-1VH2-FXUE-2FD8 sn:4442-56G7-B56L-C8DL By Alkavour (15/01/2006) http://www.8signs.com/
A
A1 DVD Audio Ripper 1.1.31 s/n: 0A6C63BD17EF881B4C7D87328E8059EE8557 http://www.dvdapp.com
A1 DVD Audio Ripper 1.1.45 s/n: 2A984B44R5D16C0F79I6C630361058421A0E http://www.dvdapp.com
A1 DVD Ripper Professional 1.1.11 Name: BRD Cult s/n: 1EE3A63A966BBA39251CE2111A6BE51A http://www.dvdapp.com
A1Click Ultra PC Cleaner 1.01.27 s/n: notHinGbUtthEbiBlE http://www.superwin.com
A1Click Ultra PC Cleaner 1.01.28 s/n: notHinGbUtthEbiBlE http://www.superwin.com
abbyy finereader 8.0 8.0 professional edition S/N: FPEF-8000-0000-0000-8564 (null)
Absoft Fortran Pro 9.0.x86 s/n: 777344-GMXS-SSG6-FGFG-GF3G-6 http://www.absoft.com
Absolute MP3 Splitter and Converter 2.2.18 Name: TEAM ViRiLiTY s/n: eh=F3GgZ3EcZZz4KzTz1jz0USbIjXzi3Oa http://www.iaudiosoft.com
Absolute Video Converter 2.5.15 Name: TEAM ViRiLiTY s/n: Drb35Ek1MpbvJZaynnBaE9191pdS55eJEq http://www.iaudiosoft.com
Absolute Video to Audio Converter 2.6.2 Name: Brainrain s/n: aNnfpufLNggQI1czda http://www.iaudiosoft.com
Absolute Video to Audio Converter 2.6.6 Name: alkavour S/N: AjyXkh5A9fxIRl7iUy By Alkavour (24/01/2006) http://www.iaudiosoft.com
AC Browser Plus 2.3.6.0 Name: Team EXPLOSiON s/n: ACBR-0C162E27-038F537F-0488-0620 http://www.konradp.com
AC Browser Plus 2.4.1 Name: Team EXPLOSiON s/n: ACBR-10E9218B-056914A2-0488-0608 http://www.konradp.com
Accessory File Viewer 5.0 s/n: 21C53W62 http://www.accessoryware.com
Accessory Picture Viewer Max 4.0 s/n: 81B32K41 http://www.accessoryware.com
Accountant Pro 8.7xx Name: any name S/N: F8700-3104436 (null)
ACDSee PRO Photo Manager 8.0.67 S/N: DNNDVH-3348T-35HJV7-3226FGZ By Alkavour (14/01/2006) http://www.acdsystems.com/
Ace Video Workshop 1.4.39 Name: alkavour S/N: 672A5F30-106C4F1E-C69ADAB8-B2D23B6C-52CEAC7B-26384CE2-0A544DA4-D90673F0 By Alkavour (14/01/2006) http://www.avi2vcd.net/index.htm
AceHTML Pro 6.05.1 Name: TEAM ViRiLiTY s/n: JNG5-SPPP-MYBT-ECDV http://www.visicommedia.com
AceHTML Pro 6.05.2 Name: TEAM ViRiLiTY s/n: JNG5-SPPP-MYBT-ECDV http://www.visicommedia.com
Acme CAD Converter 5.75 S/N: 8RDVLILP4LDVLPF388XIA697N9L92DNELUJ4G0USD5GETFQVXF 1E26BAY1YDRR8 By Alkavour (05/01/2006) http://www.freefirestudio.com/cadconvert.htm
Active Desktop Calendar 5.0.050224 s/n: 871194-10510020-890 http://www.xemico.com
Actual Worktime 1.0 Name: NiTROUS s/n: AWT-3WU7W-2P65M-9HL4D http://www.actualworktime.com
Actual Worktime 1.1 Name: BLZ-TEAM s/n: AWT-3CJ7P-2V65E-9JR4U http://www.actualworktime.com
AdLabPlus 2.71 Name: Administrator Adress: Team TMG s/n: 44013 http://www.weberconnect.de
Adobe Audition 1.5 S/N: 1137-1412-9673-2576-8300-9286 (null)
Advanced ACT Password Recovery 2.03 S/N: ACTP-36B6-TNNE-TUZX-XASBF-TXRK-QCDEG By Alkavour (10/01/2006) http://www.elcomsoft.com
Advanced Archive Password Recovery 3.01.7 S/N: ARCHPR-TBHW5SSMYGQS-3KQ2E6E9CU4S4BMN By Alkavour (10/01/2006) http://www.elcomsoft.com
Advanced Instant Messengers Password Recovery 2.90 S/N: MGPR-8HPY-WP3D-MX6F-AWURW-NGNX-BNTSF By Alkavour (10/01/2006) http://www.elcomsoft.com
Advanced MP3 WMA Recorder 5.9 Name: BRD s/n: 0B37-187D-6362-1B15 http://www.xaudiotools.com
Advanced Net Monitor for Classroom Pro 2.3.5 Name: TEAM ViRiLiTY S/N: single:73EFG04GE0 site:53R3T55T08 By Alkavour (24/01/2006) http://www.eduiq.com/netmon.htm
Advanced Net Monitor for Classroom 4.3.6 Name: http://www.bg-warez.org S/N: SN Single: 36IAKABK04 SN Site: 7BN7P12P7B By Alkavour (14/01/2006) http://www.eduiq.com/dwn/advnetmon.exe
Advanced Outlook Express Password Recovery 1.33 S/N: AOLPR-MCARW-56259-HTDNH-25245 By Alkavour (10/01/2006) http://www.elcomsoft.com
advanced system optimizer 2 S/N: 0Z15YH-C41M2U-T7GVVG-YCA0PB-6NP01H (null)
Advanced Tetric 5.0 Name: Beatrix Kiddo s/n: ABHNB-VLUWL-N83JE-875RX velowaver.com
Advanced VBA Password Recovery Pro 1.60 S/N: AVPRP-COMM-NCYVD-58735-RYAZX-97856 By Alkavour (10/01/2006) http://www.elcomsoft.com
Advanced ZIP Password Recovery 4.00.24 S/N: 00003112193920061941FFS2QECMAQQUJWMH By Alkavour (10/01/2006) http://www.elcomsoft.com/azpr.html
Adware Agent 4.81 s/n: 10408312 http://www.killersoftware.com
Agenda Pr900 French 1.87.129.7 Name: BS s/n: VB8100736 mxgs.viirex.com
Ahead Nero 7 Ultra Edition S/N: 1C82-0010-8011-0000-6136-3562-3612 (null)
Ahead Nero Ultra Edition V. 7.0.1.4b S/N: 5C82-0010-8011-0000-9016-5244-5274 http://www.nero.com/deu/index.html
Akram Audio Converter 2.13 Name: TEAM ViRiLiTY s/n: e91de1b2156316b486fc4d654156313156318f4d41bf http://www.akramsoft.com
Alap Imposer Pro 1.1.4 S/N: IPSR114-07979-75653-64681-W (null)
AlgoLab Photo Vector 1.98.53 Name: TEAM HERETiC S/N: 011MU2MASPR7GM4TC0B email:heretic@inter.net By Alkavour (24/01/2006) http://www.algolab.com/Photovector.htm
Algolab Raster to Vector Conversion Toolkit 2.95 Name: TEAM HERETiC S/N: 011MU2MASPR7GM4HTC0B Licenses:1 Email:heretic@inter.net By Alkavour (24/01/2006) http://www.algolab.com/
Alien Skin Eye Candy for Adobe Photoshop 5.1 s/n: EFJNAMIEEJBJ http://www.alienskin.com
All Video to VCD SVCD DVD Converter 2.1.0 Name: TEAM ViRiLiTY s/n: bxHxWme+58KAGIPhagomUYLfkSsrnMO4Lk3wlzSbyNv+c5f8gq http://www.anytodvd.com
Allok AVI MPEG Converter 1.42 Name: TEAM ViRiLiTY S/N: 7EEDDFF8-BF10F82F-B9BB798C-21FF0F65-15FD80C1-75D9FCE9-85D72CD3-9C3CC844 By Alkavour (24/01/2006) http://www.alloksoft.com/
Allok AVI MPEG WMV RM to MP3 Converter 1.46 Name: TEAM ViRiLiTY S/N: 9496042E-2D624DD6-3F891F4D-2A85531E-33E5D451-D01B6089-B9888CAF-5E31C588 By Alkavour (24/01/2006) http://www.alloksoft.com/
Allok AVI to DVD SVCD VCD Converter 1.58 Name: TEAM ViRiLiTY S/N: 8B81429C-2961AB2C-6593C915-32B4F65C-C706C960-72750AD2-23AAABB4-BC1105A7 By Alkavour (24/01/2006) http://www.alloksoft.com/
Allok WMV to AVI MPEG DVD WMV Converter 1.58 Name: TEAM ViRiLiTY S/N: 2FD08162-C407AA50-08BDC4A8-40A43F1E-45304108-B22AA20B-3D95A4A9-D99729FE By Alkavour (24/01/2006) http://www.alloksoft.com/
Alo RM to MP3 Converter 1.13 Name: Brainrain s/n: c5e27526d5d612bb3c2b14615959b91b http://www.alosoft.com
Alpeak CD Anywhere 1.8 S/N: PCD10C91C1ADCEDC5D06 SN: GC1C591F01A3D2B538E8 SN: SCDF3DF848A403238D18 By Alkavour (23/01/2006) http://www.alpeak.com/english/
Always On Time 1.0.1.14 Name: REVENGE CREW S/N: 138943469 By Alkavour (24/01/2006) http://www.bpssoft.com
aMac Address Change 5.0 S/N: 68149572251260 sn:25360726296294 By Alkavour (07/01/2006) http://amac.paqtool.com
Amazing Slow Downer 2.73 Name: UnderPl s/n: DF5-65P-ZS-8K http://www.ronimusic.com
Amazon DVD Shrinker 2.4.2 Name: alkavour S/N: bn6=rPzug8RQplF7Mq By Alkavour (14/01/2006) http://www.videoexe.com/amazondvdshrink.exe
Amor AVI DivX to VCD SVCD DVD Converter 2.4 Name: alkavour S/N: bFd1vZvoctOelqYK+duIYLsKHZOD2lHVApExYvArmxCz7kfUYq By Alkavour (23/01/2006) http://www.zealot-soft.com/
Amor Photo Downloader 1.5 Name: BRD S/N: 048E823B878B447FE03BE311904DCA61E3BE64 By Alkavour (23/01/2006) http://www.zealot-soft.com/
AMUST Registry Cleaner 2.1 Name: REVENGE CREW S/N: 59856b9790f8936393f9cf23300aa8a1 Email: none@none.no By Alkavour (29/01/2006) http://amustsoft.com
Anniversary Bios 2.4 s/n: 2056W475872 http://www.symphonicsoftware.com
Anti Tracks 6.0.1 Name: crysalis S/N: A641-44BC-FEEA-1110-1CE2-094A-E28A-D15F-C46C-A3A3-E7E1-8997-C97E-624C By Alkavour (14/01/2006) http://www.rightutilities.com/
Anti-Boss Key 3.91 Name: BLZ-TEAM s/n: 5D95-BF1C http://www.mindgems.com
Antilop TV Sat Remote Programmer 1.1 Name: http://www.bg-warez.org S/N: Email: crackteam@bg-warez.org Key: E60F-43EF-6CD3-4462 By Alkavour (05/01/2006) http://www.antilopsoft.com/tvsat.zip
Antivir Personal Edition 6.X S/N: Einfach eine Textdatei erstellen und diesen Text in sie hinein kopieren: H+BEDV Products License Key File 49-GDN-190-QVS-349 (null)
Anti-virus Personal Pro 5.0.390 S/N: 02d6-00006b-0007ec91 (null)
AnyDVD 5.8.2.1 S/N: REGEDIT4 [HKEY_LOCAL_MACHINE\Software\SlySoft\AnyDVD\Key] "Key"="0FAkPlBRHIiONqWrVIzyho57r3aEN3KvUtKO6OViRwz igEMLeKIqX HKMAmGBp6nrYVpty25/OuSxgbMPXxPYG5CnhtT68+KHpYnZwrpxSaC2/1 fDIBjhXiQ7SlaVm4hoJ/9oAN8WQ0mc8ZszEzGcKIvaGzNjh4khs2+aUMDDs RzJdRZG0F6VDYP8B+ 1. create file Key.AnyDVD with content REGEDIT4 [HKEY_LOCAL_MACHINE\Software\SlySoft\AnyDVD\Key] "Key"="0FAkPlBRHIiONqWrVIzyho57r3aEN3KvUtKO6OViRwz igEMLeKIqXHKMAmGBp6nr YVpty25/OuSxgbMPXxPYG5CnhtT68+KHpYnZwrpxSaC2/1fDIBjhXiQ7SlaVm4hoJ/9oAN8 WQ0mc8Zsz (null)
AP Muenze Pro German 3.09 s/n1: 53021 Name: Lutz Laub s/n2: 151272509 http://www.muenze-online.de
Apollo DivX to DVD Creator 2.0 Name: THOR_MESTER S/N: 095D5DEB-C3829F62-7B5B4148-7C941EB5-FFCC56D2-0F595445-88463E3A-9747B251 HEL??__ http://www.createdvd.net/apodvdcreator.exe
Apollo DivX to DVD Creator 2.0.0 Name: http://www.bg-warez.org S/N: F882865B-737A2844-003D950C-A0F70E5A-0403CA18-796CED30-B9778AB9-6D9BAD71 By Alkavour (05/01/2006) http://www.createdvd.net/apodvdcreator.exe
Apollo PSP Video Converter 2.0.1 Name: http://www.bg-warez.org S/N: 32435324-7B8DABE6-66BBA347-6ECB27EE-642B544E-57681246-CBC8512F-85FCCDCF By Alkavour (15/01/2006) http://www.alltomp4.com/apopspvideo.exe
Apollo Versatile Burner 3.0.0 Name: alkavour S/N: BD9CFD65-35CEDB50-5F39BE6A-B74828C3-4795C2CA-0B89D3F4-B38CEDFA-C2FCFA7A By Alkavour (05/01/2006) http://www.apollo-tech.com
Ardamax Keylogger 2.4 Name: REVENGE CREW S/N: VRWQWPDWRTBXBRT (null)
Arial Audio Converter 2.1.6 Name: TEAM ViRiLiTY s/n: fFrow5Y=f2RwlmtU2B+p5HIEHmneTMl9zq http://www.xrlly.com
Arial CD Ripper 1.3.80 Name: TEAM ViRiLiTY s/n: edda9e251cc3d9b84960a2e077b2e6fd http://www.xrlly.com
Arial CD Ripper 1.4.4 Name: TEAM ViRiLiTY S/N: edda9e251cc3d9b84960a2e077b2e6fd By Alkavour (24/01/2006) http://www.xrlly.com
Arial Sound Recorder 1.2.5 Name: TEAM ViRiLiTY s/n: Hwi7g7Lss55r70WtbMSGUOYCtMh+LJKS http://www.xrlly.com
Armor Tools 4.0 s/n: 1436418372-BLZ-3717025688 http://www.wintools.net
Ashampoo Burning Studio 5 5 S/N: 545136 (null)
Ashampoo Magic Defrag 1.10 S/N: AMDGA5-77HS10-KHT11P sn:AMDGAN-770RFC-4YX3DN sn:AMDGAB-77X4CE-UBF4FX By Alkavour (24/01/2006) http://www.ashampoo.com/
ASHAMPOO MAGIG BURN V.5 S/N: AMB535-77SB4A-9DXO15 AMB5JM-773N57-BT889G AMB5EK-77A9DC-OFU88B (null)
Ashampoo Photo Commander 4 Name: Sheena Sudan S/N: APHC04-77D50E-CDE9BC no more need for windows media player, winamp, etc. to play movies and songs it is best ever all in one apllication. http://www.ashampoo.com
Ashampoo Photo Commander 4.00 S/N: APHCB0-7771AC-116CAC http://www.ashampoo.com
Atani 3.3.0 s/n: 6V0XMNIU757 http://www.atani-software.net
Atomic Mail Sender 2.90 S/N: DV5jMEzhms6CqHyryS sn:ziA5LcNqo7aCMlayrF sn:lH9hkyoyhUpdGl7IWl By Alkavour (14/01/2006) http://www.amailsender.com/
Audio Edit Magic 7.5.9.675 s/n: AEM5942714 http://www.mp3editmagic.com
Audio Notes Recorder 6.1 S/N: 199808-101868C5-184 (null)
AudioGrail 6.4.0.99 Name: REVENGE CREW S/N: 61089114095081129078114032079065076132 By Alkavour (10/01/2006) http://www.kcsoftwares.com
AudioList Plus 3.7.6 Name: AGAiN tEAM s/n: 12448 http://www.wakefieldsoft.com
Auftrag 2.3.7.19 Name: TEAM ACME s/n1: AU151070873 s/n2: 23274256 http://www.zwahlen-informatik.ch
Aurora MPEG To DVD Burner 4.78 Name: alkavour S/N: 3863FDD3-1F4735C6-85F957E6-598FEDB0-900328C5-0B30D931-D8B925F2-A38F9DAC By Alkavour (24/01/2006) http://www.mediatox.com/
Aurora Video VCD SVCD DVD Converter And Creator 4.1.7 Name: BRD Cult s/n: FB7A58CD-002BAFDC-4C56DF3E-E39F27AE-5EE0F935-B4DF19E4-0303B2F6-E9FB39C0 http://www.mediatox.com
Auto Cleaner 3.0 Name: nGen team s/n: dc4ea7acc09552de0f3f7f1b8834e851 http://www.superscan.net
Auto MP3 Player Win2kXP 1.20 Name: CRUDE s/n: 123456X188035 http://www.lifsoft.com
Auto Power on and Shut down 1.44 s/n: JXA?<5026<=90 http://www.lifsoft.com
Auto Power-on and Shut-down 1.45 s/n: 13A?=656=2614:= http://www.lifsoft.com
AutoRun Assistant Pro 3.0.1 Name: TEAM ViRiLiTY s/n: 43N4U632333413-697A3 http://www.typhoonsoftware.com
AutoRun Assistant 2.9.5.020805 Name: nGen 2oo5 s/n. 6W56827N52-948702389 http://www.typhoonsoftware.com
AutoRun Assistant 2.9.6.021605 Name: TEAM ACME s/n: 4N05803H52-948702389 http://www.typhoonsoftware.com
AutoRun Design 3.0.0.16 Name: TEAM ViRiLiTY S/N: 001B9B36BC7CE94E key:EBDC53065B0A752C337795AAA35D7E1C By Alkavour (10/01/2006) http://www.alleysoft.com/
AutoRun Pro Enterprise 4.0.0.34 Name: http://www.bg-warez.org S/N: S/N:0018A901AAB74AEB Key:068A26A851CAA0F9 http://www.longtion.com/autorunenter...RPESetup40.exe
AutoScreenRecorder Pro 2.1.285 S/N: license type:single user number of users:1 license key:AS2P-VZ9Y-D6AE-035V-OZI@ or license type:custom number of users:99 license key:AS2P-999O-B0KG-945F-3XSL By Alkavour (15/01/2006) http://www.wisdom-soft.com/products/...enrecorder.htm
AuVer 1.48.2 s/n: 1113/s2060160/8T37 http://www.saonet.de
AV VCS Diamond 4.0 S/N: 14022483 (null)
avast! 4 Professional Edition Download 4.6 S/N: S3659261R0021S1021-A9PAH1AZ S3405626R0021S1021-2UR9U6SJ S4615515R0021S1021-1UBP0XW1 Excelent!!!! Two years free http://www.avast.com
avast!_Professional_Edition 4.6.744 S/N: C5825354R0021S1021-P3B4MHYH (null)
AVConverter MP3 Converter Pro 4.1.18 Name: Team Linezer0 S/N: C0F44CC0-14E3CBD1-47C9F60B-3C544ACF By Alkavour (14/01/2006) http://www.avconverter.com/
AVG Anti-virus 7.1 build 371 S/N: 70-TF10Z1-P5-C02-SCM5Y-J4S-3HQJ this one works (null)
AVGA Antivirus 7.1 Build 371 S/N: 70-THXMV1-PJ-C21-SACQY-3WN-KKS8 (null)
AVI DivX MPEG to DVD Converter and Burner Pro 1.6.0 Name: TEAM ViRiLiTY s/n: dN5Xg3xk4celaJ6TsCvQnkR4pK81ltwrAHrDSWOPeksATuQQt1 quh6g http://www.anytodvd.com
Awesome 3-Card Poker 1.0a Name: UnderPl Team s/n: 1812642 http://www.simplyawesomesoftware.com
Awesome 50-Play Poker 1.0a Name: UnderPl Team s/n: 2742202 http://www.simplyawesomesoftware.com
Awesome Let-It-Ride 1.0a Name: UnderPl Team s/n: 1254906 http://www.simplyawesomesoftware.com
Awesome Triple-Play Poker 1.0a Name: UnderPl Team s/n: 4136542 http://www.simplyawesomesoftware.com
Awesome Video Poker 1.0a Name: UnderPl Team s/n: 790126 http://www.simplyawesomesoftware.com
AXIALIS ICON WORKSHOP corporate edtion 6.0 S/N: 33513544-02633-02553-62824-35121 (null)
B
Backup Made Simple 5.1.186 S/N: 1649-6DF1-62E7-E3B3 SN: 2CD6-72AE-E334-4CA5 SN: 26E9-01EB-E297-993A By Alkavour (24/01/2006) http://www.willowsoft.com
Backup Made Simple 5.1.91 s/n: 2D3E-8A23-E963-A299 http://www.willowsoft.com
Backup Made Simple 5.1.92 s/n: S6m4-cHc9-8256-337E http://www.willowsoft.com
Backup To CD-RW 5.1.91 s/n: 7C2C-88B9-538B-A42E http://www.willowsoft.com
Backup To CD-RW 5.1.92 s/n: CIlH-1fR9-915C-C33E http://www.willowsoft.com
Bandes Annonces Extractor 4.0.5c s/n: VGHK-SDLC-MPLU baextractor.free.fr
Basic Inventory Control 5.0.115 Name: TEAM ACME s/n: 8600410 http://www.microguru.com
Batch Image Resizer 2.10 Name: Team EXPLOSiON s/n: M6DWYIRG6C4X5K56 http://www.anytodvd.com
Batch Image Resizer 2.16 Name: Team EXPLOSiON S/N: M6DWYIRG6C4X5K56 By Alkavour (12/01/2006) http://www.anytodvd.com
Batch Image Resizer 2.16 Name: http://www.bg-warez.org S/N: 3XUSYRHRIAQ3RIF6 By Alkavour (14/01/2006) http://www.anytodvd.com
Batch Image Resizer 2.18 S/N: Email: bauerlindemann@laxity.ru Key: MNSMMCHI3N7YU7PP By Alkavour (29/01/2006) http://www.anytodvd.com
Batch Image Reziser 2.15 S/N: email : www@serials.ws sn: FMFL5A68IX2VLQGR http://www.jklnsoft.com
Batch Video Converter 1.8.0 Name: TEAM ViRiLiTY s/n: ArU22Ll7sDJJqwiHA0nZ4o7XopCu7DIYTlzqHsIICrO76NhITy http://www.anytodvd.com
Batch Video Converter 2.0 Name: alkavour S/N: AKgfOz58nCy+R=z9FJPxfmZCMJi14aHA3vyFgeoOga0wGYKq1i By Alkavour (24/01/2006) http://www.anytodvd.com
Batch Video Joiner 2.0.0 Name: TEAM ViRiLiTY s/n: BdvcoeTqc=c2uk8UUkH5JoN6poNxwbYy4ger1uQcThvokr=Jtq http://www.anytodvd.com
Batch WMV to AVI MPEG WMV VCD SVCD DVD Converter 2.0.0 Name: TEAM ViRiLiTY s/n: bZsJaX1Lu1ULRhI=aYsalq09q4YkJ2YLBGohMx8HpaeTmZe=ci http://www.anytodvd.com
Battlefield 2 1.12 S/N: 3ETB-6Z8W-GV6Q-XDG3-6E89 Working Key http://www.ea.com
BCAD For PC Versions (Designer) 3.9.723 s/n: DS05GE-15773073 http://www.propro.ru
BCAD For PC Versions (Engineer) 3.9.723 s/n: ET05IT-5380828 http://www.propro.ru
BCAD For PC Versions (Furniture Pro) 3.9.723 s/n: MP05GE-3730913 http://www.propro.ru
BCAD For PC Versions (Standard) 3.9.723 s/n: ST05IT-306723 http://www.propro.ru
BCAD For Tablet PC Versions (Designer) 3.9.723 s/n: DS05GE-15773073 http://www.propro.ru
BCAD For Tablet PC Versions (Engineer) 3.9.723 s/n: ET05IT-5380828 http://www.propro.ru
BCAD For Tablet PC Versions (Furniture Pro) 3.9.723 s/n: MP05GE-3730913 http://www.propro.ru
BCAD For Tablet PC Versions (Standard) 3.9.723 s/n: ST05IT-306723 http://www.propro.ru
Becky Internet Mail 2.12.01 s/n: 7006-3437-M753 http://www.rimarts.co.jp
Becky Internet Mail 2.20.01 Name: TEAM WDYL-WTN s/n: 4001-3437-K867 http://www.rimarts.co.jp
Belltech Buisiness Card Designer Pro 3.0 S/N: Name: Buttercup Serial: LQ4Y8PYAUY48488G (null)
Belltech Greeting Cards Designer 3.0 S/N: Name: Buttercup Serial: 597HOSR3DH5RMR5Z (null)
Belltech ScreenSmart 3.0 S/N: Name: Buttercup Serial: 12400107Mv{1496035 (null)
Belltech Small Business Publisher 3.0 S/N: Name: Buttercup Serial: VZ5951XE595HDH5R (null)
BestAddress HTML Editor 2005 Professional 8.1.0 Name: LUCiD s/n: 4794 - 1806 - 1533 - 5472 http://www.mmaus.com
BestMan 1.5.1 Name: TEAM CAT 2005 E-Mail: TEAM CAT 2005 s/n: CL1K3V46PFLR8H73 http://www.sassenfeld.de
Bird Bricks 2.0 s/n: 2156549921 http://www.sumagames.com
Birthday Bios 4.3.0 s/n: 4136T6834 http://www.symphonicsoftware.com
BitDefender Professional Plus 9.0 Name: Mad^Agent S/N: 651F9-94C67-751FC-919E2 http://WwW.MadAgent.Tk http://www.bitdefender.com/
BitDefender Professional Plus V. 9.0 S/N: 0B300-9FE73-BF068-99BB1 http://www.bitdefender.com/
Bitdefender Standard 9 S/N: EF837-721BE-2008C-7F029 http://www.bitdefender.de
Blaze Media Pro 1.6.0.0. S/N: BMP7-A720-DA21 (null)
BlitzCalc 1.0.0.97 Name: Team EXPLOSiON s/n. 44-825c-9eec-d711-aa http://www.tripence.com
BlitzCalc 1.0.1 Name: Team EXPLOSiON s/n: 44-825c-9eec-d711-aa http://www.tripence.com
Blog Navigator Professional 1.2.1.272 s/n: BN-S5KQ2-M8REA-2OF14-6KHLF-4208966334 http://www.stardock.com
Blumentals iNet Protector 2.21 s/n: INP2SECURE http://www.blumentals.net
Blumentals Screensaver Factory Enterprise 4.0 S/N: SCF4ESUPER By Alkavour (15/01/2006) http://www.blumentals.net/scrfactory/
BMS Fahschultrainer 2006 2006 S/N: Name: NICOLE WEISS Schl??ssel: 048Z595O01839 (null)
Bonaparte 1.4.6 Name: EMBRACE s/n: NPB-2005-TA24* http://www.en.bonaparte-game.com
BookBag Plus 3.7.6 Name: AGAiN tEAM s/n: 12718 http://www.wakefieldsoft.com
Bowl101-IX 1.6.46 s/n: 92505 http://www.bowl101.com
Brockhaus Multimedia 2006 DVD 2006 S/N: XY58C-PM87-HFYS (null)
BrowserBob 4 Basic 4.1.2.0 s/n: AXiS123186270063 http://www.browserbob.com
BrowserBob 4 Developer 4.1.2.0 s/n: AXiS122025369262 http://www.browserbob.com
BrowserBob 4 Professional 4.1.3.0 s/n: AXiS126716170163 http://www.browserbob.com
BT Engine 4.5.0103 Name: vorteX s/n: 840594603855 http://www.soft4kids.com
BulletProof FTP Client 2.52 Name: Knight/TSRh TeaM S/N: no of licenses:1 Line1:ILV]F8QfTVUeReSiZ:C\HTPgEIZ\OOC@YjV>JnUcJLO`MVJGR*GUOX PqQEVOM7V`SlD-Cn Line2:P:X]B+MfSLJ#HiQ"JiLfR,D;ONV0N]UmJ9N;MaO^VKRFB(X-D By Alkavour (07/01/2006) http://www.bpftp.com/
Business Cards Designer Pro 3.0 S/N: SW26H7P9262EAE2N (null)
Business Letter Pro 2006 5.2.0.0 Name: DIGERATI s/n: DKOE-PYGP-MGLN-EKUE-1MGP-UG1N http://www.ematrixsoft.com
C
CachemanXP 1.1.0.0 S/N: CX101-9KD3X-7WNS8-UFRPT-DY00J-01W10-1E020 (null)
Calcsharp 1.0 Name: Team DiGERATi s/n: Q4E4F-A23E3-F12S3-D1D34-D123R http://www.calcsharp.net
Calendar Builder 3.3a Name: NiTROUS s/n: RKS-1126301 http://www.rkssoftware.com
CalMaker 1.5 Name: nGen 2oo5 s/n: 245672 termulator.free.fr
Campaign Suite Extended Developer 05.09.03a Name: Lutz Laub s/n: 1030-7978-2911-3713 http://www.twinrose.net
CamUpload 1.1 Name: anything s/n: 000010010000 http://www.spanto.com
Catalogue Pro 4.12.13 Name: Team Cafe s/n: j5A1-373j-ocHx-h536 peccatte.karefil.com
CD Bank Cataloguer 2.6.5 Name: NiTROUS@TEAM.COM s/n: 42GULWYMGTUTWFW3 http://www.qunom.com
CD Bank Cataloguer 2.6.6 Name: TEAM@TBE.com s/n: YOUR-TBETEAM-2005-E http://www.qunom.com
CD Extraction Wizard 1.7 s/n: CDEW-3DF2-3D99-6932-1B43 http://www.streamware-dev.com
CD To MP3 Ripper 1.0 s/n: END9432-6592-NER4530-1052-YRP5431 http://www.musicalsky.com
CD To OGG_Ripper 1.0 s/n: NGE4523-9628-HEB9456-9234-WAL9546 http://www.musicalsky.com
CD To WMA Ripper 1.0 s/n: MVX8530-2875-YEB5294-4634-EPB3405 http://www.musicalsky.com
CD Wave Editor 1.94.6 Name: Chief Wiggum s/n: EF5EFA62EC74EE93 http://www.milosoftware.com
CDMenuPro 5.00.03 Name: CLUSTER/BLiZZARD! s/n: 18825-40184-98765-90265-321123454 http://www.cdmenupro.com
Cheat Guru 1.0 Name: nGen team s/n: DC4EA7ACC09552DEF3F7F1B8834E851 http://www.customessay.org
Cheetah DVD Burner 1.5 s/n: 0192837465-3000 http://www.cheetahburner.com
Christmas Time 3D Screensaver 1.0 S/N: Name: Code: BOAUGRZWET http://www.digimindsoft.com
Cinema 4D Production.Bundle 9.507 S/N: Cinema 4D: 10901098125-JNMH-PMVW-HKDR-NSLD BodyPaint: 31000098125-SHGB-VRSN-JFWN-VWRL Advanced Render: 32100098125-HDLD-ZKHT-KLHC-MSJH Dynamics: 36100098125-TLXV-LZGB-KJPJ-SXZT MOCCA: 35100098125-MRTB-FDHL-DTVH-GPLM PyroCluster: 341000981 (null)
CK-Software AuktionMail 2.2.0.89 Name: ReD0c/GEAR S/N: 4111-5345-3000-GEAR http://www.ck-software.de/
CK-Software Einladungskarten Designer 1.3.1.28 Name: ReD0c/GEAR S/N: 3602-4827-4239-GEAR http://www.ck-software.de/
CK-Software Personal Mailer 6.3.4.126 Name: ReD0c/GEAR S/N: 4111-5345-2300-GEAR --- Addon GetMail: ReD0c/GEAR 2528-3383-2700-GEAR http://www.ck-software.de/
CK-Software Testauswertung DTVP-2 2.0.1.60 Name: ReD0c/GEAR S/N: 4111-5345-3100-GEAR http://www.ck-software.de/
CK-Software Testauswertung DTVP-A 1.1.0.68 Name: ReD0c/GEAR S/N: 4811-4045-3137-GEAR http://www.ck-software.de/
CK-Software Visitenkarten Designer 1.6.1.16 Name: ReD0c/GEAR S/N: 2602-1827-4219-GEAR http://www.ck-software.de/
Clarysis Executive Powerpak 4.0 DC04252005 s/n: 436850374 http://www.clarisys.ca
Clarysis Executive 4.0.0.6 SP3 s/n: 320163173 http://www.clarisys.ca
CleanMantra 2.0 Name: CRUDE s/n: 1f9d6fec9876584dc716e29efdfbfb02 http://www.pcmantra.com
Click n View 3.0.6 Name: alkavour S/N: LJG9-GE1J-09L4-IBTS By Alkavour (12/01/2006) http://www.enfull.com/download3.asp?id=490&soft=enfull1
ClickAndHide 1.1 Name: 4kusN!ck S/N: MLDBBQLKNEEMRAOA Mail: 4kusNick@revenge-crew.com By Alkavour (29/01/2006) http://www.oleansoft.com/clickandhide.htm
Clipboard Box 2.3 Name: iNFECTED s/n: 82010910878706967 http://www.dreamflysoft.com
Clipboard Box 2.5 Name: alkavour S/N: 82010910910810797118 By Alkavour (24/01/2006) http://www.dreamflysoft.com
Clippper 3.6.1 Name: REVENGE CREW S/N: JL24523LNOL3KdL3IKLKKM4257NcJ54I2546NL5LIJM7KJ446M L6N53LMMI0I Email: none@none.no By Alkavour (29/01/2006) http://clipper.nohik.net/
CloneDVD 3.9.0.0 S/N: 435AL48LSX5M5 (null)
Colored ScrollBars 1.1 s/n: 2h8v4c5uMi16 yaldex.com
Combustion 3.x S/N: serial: 341-12345678 activation code: 978e421f (null)
CompassBox 2.0 S/N: 3680587144695764 http://www.genobz.sail
Computer Alarm Clock 2.2 Name: TEAM ViRiLiTY s/n: e3k999571n3 http://www.tarsoft.com
Copy DVD Gold 2.01 Name: http://www.bg-warez.org S/N: 486EA07561B592A58 By Alkavour (05/01/2006) http://www.copydvdgold.com/copydvdgold.exe
CopyKiller 3.64 Name: 1285 s/n: 47981 http://www.webstylerzone.com
counter strike 1.6 S/N: S/N: AAAAA-AAAAA-AAAAA-AAAAA-AAAAA Note: works also for "CounterStrike: Condition Zero" Retail. (null)
Counter-Strike: Condition Zero 1.0 S/N: 5ZN2D-DHZ4F-3HWVC-DYVQG-MBHJB (null)
CPU Guard 1.2.22 Name: http://www.bg-warez.org S/N: 606618-0137-8291-5984-0FC5EFE1 By Alkavour (15/01/2006) http://www.sysint.no/nedlasting/SetupCpuGuard.zip
Crazy Bubbles 1.1 1.1 S/N: CBUB-987654-0A3ED0 (null)
Creative Painter 3.2 s/n: DKAP5T17DZoEfv8rxQZ7 http://www.mokosystems.com
Cricket Statz 2005 Standard 1.2.4 Name: TEAM ACME Club: ACME s/n: E460B05A13F88408 http://www.cricketstatz.com
Cricket Statz Standard 2005.1.2.3 Name: Team Cafe Club: Team Cafe s/n: 865412B7838B681E http://www.cricketstatz.com
Crossword Forge 3.6 Name: Kent s/n: ZUUCCCVVC4 http://www.scotandrews.com
Cryptime 3.4 Name: 4kusN!ck S/N: PLLBQIHIGKKPDAOC Mail: 4kusNick@revenge-crew.com By Alkavour (29/01/2006) http://www.oleansoft.com/cryptime.htm
Crystal Player Professional 1.96 S/N: 00022831EC81D740DCC40A3BFA479DF4E9F80F2CEB2E405C42 2F5C5134A26E7F2BD 39FDF932E67992037B296A801DCFA44AB6C7F53BF886B4ECE6 D33CD55CB9F511899 2FFDABCA1E83709448AF5D4A46FA0FD5C7F1B9C52BB6160A1D 71602FC0437575A9D FFE3692294CFAFB6025AA820D7DA1460FAD0E5E1A1B45BEE7D 12CC
[nyambung semua ya...?]
http://www.crystalplayer.com
Crystal Reports Activation Number 9, 10, 11 S/N: 8013180283 (null)
CurveMeister for Adobe Photoshop 2.1.0b S/N: WSG-CXT-ERU By Alkavour (10/01/2006) http://www.enfull.com/download3.asp?...7&soft=enfull1
Customer Database Pro Multi-User Version 6.0.108 Name: TEAM ACME s/n: 43344-424D4-83633-393FF-FFFFF http://www.microguru.com
Cyberlink PowerDVD CLJ Deluxe 6.0.0.2023 s/n: TFVE4-EWSRP-57BZJ-JJSPB-6TJM3-BTMF8 http://www.gocyberlink.com
Cyberlink PowerDVD CLJ Express 6.0.0.2023 s/n: H9ESH-U86W3-8P8QP-AXWWS-ZZLEG-4FV7X http://www.gocyberlink.com
Cyberlink PowerDVD CPRM Pack 6.0.0.2023 s/n: E4LTN-Y92ZN-3E2WM-YNE55-YFB79-REHZN http://www.gocyberlink.com
Cyberlink PowerDVD Deluxe 6.0.0.2023 s/n: ST9X4-AZWW9-CHJGD-VPYRH-EYH26-JVUC6 http://www.gocyberlink.com
Cyberlink PowerDVD DivX Pack 6.0.0.2023 s/n: 2FE9Y-9ZQTX-ML5VA-R99Y8-7JJQD-QXYL5 http://www.gocyberlink.com
Cyberlink PowerDVD DTS 96/24 Pack 6.0.0.2023 s/n: 6QL8C-RUR32-D4W2R-5EU3Q-Y77Z4-XQ95G http://www.gocyberlink.com
Cyberlink PowerDVD DTS Pack 6.0.0.2023 s/n: EPDYL-FWU2D-BUG66-FN5QJ-8KNPH-MJADA http://www.gocyberlink.com
Cyberlink PowerDVD DVD-Audio Pack 6.0.0.2023 s/n: UVXGY-3LDHA-4PG9C-335RU-YA28R-UBMHX http://www.gocyberlink.com
Cyberlink PowerDVD Express Pack 6.0.0.2023 s/n: WA8ZZ-D86TY-67E6D-A29CB-N93XB-UD5BP http://www.gocyberlink.com
Cyberlink PowerDVD Express 6.0.0.2023 s/n: RCCSV-8EJHX-EUEPQ-PNEW4-GV6XD-K5ZGZ http://www.gocyberlink.com
Cyberlink PowerDVD Interactual Pack 6.0.0.2023 s/n: H4DEG-Y8Y4U-HB2KV-ELJF9-XAPJ6-UN74V http://www.gocyberlink.com
Cyberlink PowerDVD OEM 2CH 6.0.0.2023 s/n: HD2YW-WE2BB-VB5PE-FWZNH-U8QJS-UN9CV http://www.gocyberlink.com
Cyberlink PowerDVD Standard 6.0.0.2023 s/n: VHCBB-7TDRE-6GQ9U-6BQHY-ENLCC-TRF6N http://www.gocyberlink.com
_________________
Ilmu Akan Bermanfaat Bila Membiasakan Untuk Saling Berbagi
D
Daily Planner Plus 4.8 s/n: DAILYPLANREG2000 http://www.reg-software.com
Dawn of War 1.0 S/N: 1e6a-1d77-3492-844e (null)
DB Blob Editor 2.5 Name: REVENGE CREW S/N: 54912-252150-6507217 By Alkavour (29/01/2006) http://www.withdata.com
DearDiary 2.04 S/N: S018H133I270V3 By Alkavour (10/01/2006) http://www.sgssoft.com
Deluxe Ski Jump 3 1.4.0 Name: Arne Sandsether S/N: 0028-8517-1688-1354-7981 Delete the license.cfg located in the user data and type the serial in the game.Good luck! (null)
Demonstration Screen 1.4 Name: 4kusN!ck S/N: EGAFADDGCGCDBCFF Mail: 4kusNick@revenge-crew.com Quantity: 100 By Alkavour (29/01/2006) http://www.oleansoft.com/dscreen.htm
Der Brockhaus 2006 2006 S/N: DU5WW-EV34-KYLT (null)
DeskCalc FiStWare 3.0.6 Name: Team EXPLOSiON Company: Team EXPLOSiON s/n: TAX-V3-K7AICKGU http://www.deskcalc.com
DeskCalc TaxPro 3.2.1 Name: .:Team ACME 2005:. S/N: Firma:ACME Inc. serial:CAL-V3-SSHZTYGOA By Alkavour (14/01/2006) http://www.deskcalc.com
DeskCalc TaxPro 3.3.1 Name: .:Team ACME 2005:. Company: ACME Inc. s/n: CAL-V3-SSHZTYGOA http://www.deskcalc.com
Developer Express CodeRush for Visual Studio 1.1.44 S/N: 2004-5F75-104DC7BD-029338 sn:2004-5D76-F0B88C26-705511 sn:2004-C9F5-94E8A1FB-243708 By Alkavour (24/01/2006) (null)
Developer Express Refactor Pro for Visual Studio 1.0.31 S/N: 2005-5388-330310E5-010780 sn:2005-CEFB-A6CC4053-186502 sn:2005-5D76-E6AEFE24-695611 By Alkavour (24/01/2006) http://www.devexpress.com/
Diashow pro 9.0 S/N: 0H15J8C6A3C0J6G7I38 (null)
Dictionnaire Robert Collins fran??ais anglais v.5 2002 S/N: XIFR8-77NBS-QJ0QH-C9FVF (null)
Die Sims Deutsch S/N: 114647-933076-400627-7067 (null)
Digital Audio Editor 4.7 Name: Anything S/N: Company: Anything Code...: DAE5946742 By Alkavour (12/01/2006) http://www.audioeditor.us/
Diner dash 1.2 Name: http://www.bestserial.com S/N: PTB6R-GAEUL-VOPVJ (null)
DirWatcher Pro 2.3.176 Name: TEAM ViRiLiTY Company: TEAM ViRiLiTY s/n: 935-9487566-7128 http://www.polarsoft.de
Disk Director Server 10.0 S/N: English sn: XE8WX-GBBQG-2FSDD-F2WRB-D7AT7 German sn: T7QUR-G6F42-JNCU4-547ZV-3QGC6 Russian sn: MZ32P-J39GF-HYGZK-PKFW7-KTZZ8 French sn: QVBW8-WN5K2-ABSUJ-VGEPQ-KUS6X Czech sn: 3HN9R-DFGW6-4L7UB-MSDR5-PV2AR By Alkavour (05/01/2006) http://www.acronis.com
Disk Director Suite 10.0 S/N: English sn: VDUWK-YDRPX-4KU5C-393ZA-EME7B German sn: PWN8K-KNG32-8D9VN-VMS2D-VGQQ8 Russian sn: ZSYLT-K8VE5-HSPFH-98AM3-XCAG5 French sn: 25QUK-XMR6B-KK9MW-QRKEM-AJDRD Czech sn: 65X7K-S4U3H-NKLGQ-LDY97-VQ3BK By Alkavour (05/01/2006) http://www.acronis.com
Disk Director Suite 10.0.2077 S/N: English sn: N4NEC-UQYMF-MX2DX-2U4SK-NCNAP German sn: 2RKXK-U525C-FATTM-ESNMR-2N7C2 Russian sn: PLC6N-Y7CMX-ELXHG-42HQ3-AFF9S French sn: 3ZTB4-KAPJY-5PT5R-NSJPH-8MGHT Czech sn: F8672-VPRBH-XVKXG-3UFDP-TDV2K By Alkavour (05/01/2006) http://www.acronis.com
DiskGrabber 1.2.2.194 s/n: 4679-3856-0577-9574 http://www.softpile.com
DiskView 3.3 Name: Simon Cranmer S/N: VB7ZQH-WCXA3Z-ZA63Y0-A492AP-KN6G5D-C4T8RA-72NVXU-0QPUJE http://www.diskview.com/
DivX Create Bundle 6.11 S/N: ZDIJTNUJ78RYAVVRZYFC sn:EDKUPVNV3GRKJ8BWTVHD sn:YVAUJN6Z3CMYFHC7YAYC By Alkavour (24/01/2006) http://www.divx.com/
DivxToDVD 2 1.99.24.66 S/N: M4MLW - SPE4Q - UPLTV - QP6AV - 8S8Y9 - L http://www.vso-software.fr/
Doc-O-Matic Pro 5.0 Name: MySTiC S/N: company:SSG sn:eqWPGWL38+MO1vfSstB1g5vxkm1YkxRwaWps9xcJ By Alkavour (24/01/2006) http://www.doc-o-matic.com/
Documents To Go 8 S/N: Registration # 9854862-9094 Activation # 8E5F-9600E94B612F http://www.dataviz.com
Documents To Go Symbian UIQ S/N: 350443-10-372640-0 http://www.dataviz.com
DoInventory Plus 3.7.6 Name: AGAiN tEAM s/n: 10693 http://www.wakefieldsoft.com
Driver Genius Professional 2005 6.0.1882 S/N: License Quantity : 1 Registration Name : TEAM TBE 2005 Registration Code : K8ENE-RY5EKQY68RKVDX5 (null)
DriveSitter 1.3.5.3 Name: Team EXPLOSiON s/n1: 23015 s/n2: DS-7X1ZNY5U-URNPZ1Y3-Y8S3Z4S7-0Q9X5OU0-L5U91TT1:Z0.V8U81YV2 http://www.otwesten.de
DSL Speed 3.1 Name: TEAM TBE s/n: PDDI33L6JTW6 http://www.dsl-speed.org
Dual Burner For MP3 Players 2005 6.2.0.418 Name: tam/CORE s/n1: 379340380 s/n2: 5F7F00AE2600 http://www.net-burner.com
Dual DVD Copy Gold 4.07 Name: iNDUCT S/N: 6H0>Q-AOEOM-3EVY3 By Alkavour (23/01/2006) http://dualdvdcopy.com/
DVD Audio Extractor 3.5.0 S/N: DA1X6ZY5EPOD snA1XHSUIWIKX snA1XYKHTOAWJ By Alkavour (14/01/2006) http://www.castudio.org/dvdaudioextractor/index.htm
DVD CD Data Burner 2005 6.1.0.536 Name: tam/CORE s/n1: 379340380 s/n2: 435F9AF83A00 http://www.net-burner.com
DVD PixPlay 2.23 Name: Team@EXPLOSiON.2005 s/n: dpp2cmr37ex5sb2vqe9hnii43 http://www.xequte.com
DVDComposer V.1.0.5 Name: Knugen S/N: 1089B0EA45B849F8 (null)
DVDInfoPro 4.36 S/N: 92604-07715-95927-44862-75228 sn:38653-66429-81012-15195-43738 By Alkavour (07/01/2006) http://www.dvdinfopro.com/
dw 22 S/N: 34354435 (null)
DynSite 1.11.780.5 Name: TEAM ACME s/n. 06717-251 noeld.com
E
Easy CD-DA Extractor 8.0.1.2 E-Mail: howard.young@rheintal.com s/n: 000015-KKZNJG-GFRDM3-4M8DEA-5A5QTM-WJQG1N-JZMXD0-URZAJZ-DW1NND-QPYYKG http://www.poikosoft.com
Easy Contacts Manager 1.35.DC10152005 Name: nGen 2oo5 s/n: Con6-53F62bsar-F623 http://www.lenosoft.com
Easy DVD Extractor 1.2.3 Name: TEAM ViRiLiTY s/n: Q@DH083108W10W3108Q http://www.witcobber.com
Easy File Sharing Web Server 3.0 Name: TeFmEXPLOSisNL S/N: Yrc8gUW4xBmwk07I9zEk0OwQfD3C By Alkavour (07/01/2006) http://www.sharing-file.com/
Easy Quittung 1.5.1.55 Name: Team ACME s/n1: EQ141411 s/n2: 91388103 http://www.zwahlen-informatik.ch
EaysTagger 2.2 build 193 Name: Fade S/N: C6C29346 http://www.istoksoft.com
eBible For Palm OS 1.0 S/N: 9G49-00XB-EPAL-A09V (null)
Edit4Win 2.02 s/n: 91-36C6322B-E700-980D-EQTETU-77710F-78329075AC http://www.edit4win.de
EditExt 5.5 DC10212005 s/n: EP-0285123456789 http://www.alcodasoftware.com
E-Ditor 3.0 build 1090 Name: EGOiST s/n: 0679859299A8BF http://www.e-ditor.com
Einkaufsplaner 6.0.1 s/n: SW5RI7F94M http://www.rolandwagnersoftware.de
Elegance Technologies C-Sharpener for VB 2.0 s/n: 9D115115591454315C1D4B http://www.elegancetech.com
Email Pump Engine 2.03 Name: NiTROUS s/n: 594i376f http://www.offsitelabs.com
Email Pump 2.02 Name: NiTROUS s/n: 594i376f http://www.offsitelabs.com
EngInSite PHP Editor 3.4.2.214 Name: ESH-B7195D S/N: 44F2B-5977A-D337A-6F4DE By Alkavour (14/01/2006) http://www.enginsite.com
Enteo Citrix Management Suite 5.8.91 s/n1: 34-8570-880472 s/n2: 3B91DF0BBC3G7F59B5DA http://www.enteo.com
Enteo NetInstall Enterprise 5.8.1236 s/n1: 12-4602-935557 s/n2: 52F19GBD55A658F4CBD1 http://www.enteo.com
eovia carrara v4 pro S/N: RF40CRD-001336-PWW works (null)
Equity Evaluator 6.0.3 Name: TeaM BRD s/n1: 989898 s/n2: eu6GRJfWdbEmewXGEgfMxbyIe http://www.net-equities.com
EWIDO ANTI MALWARE 3.5 S/N: 3925-6307-8316-9835 9623-8715-1629-9638
Ewido Security Suite 3.5 S/N: 3925-6307-8316-9835 9623-8715-1629-9638 2647-9115-6675-4837 7557-3204-8123-2239 (null)
Exact Word 5.2.5 s/n: EPS-8745475506497 http://www.alcodasoftware.com
Exe Warpper 2.1 s/n: RGNXHZGRJDHNJZCBBYDBDDCFY-533FV2 http://www.533soft.com
Executable File Icons Changer 3.85 s/n: IESY-QGQC-SIME-MCOY-3571 exeico.softboy.net
Executable File Icons Changer 4.0 s/n: 9#81119129YPPSSUANX0INF1GOSMITKGPNZPOWOF4 exeico.softboy.net
EximiousSoft GIF Creator 2.51 S/N: 9859664036 By Alkavour (15/01/2006) http://www.eximioussoft.com/
eXPert PDF 2 Professional 2 S/N: B117-70A9-8469-44AD-C29F-E4A7 (null)
eXPert PDF 2 Standard 2 S/N: 7B4F-4162-8569-42AD-C29F-EDA7 (null)
ExpressMirror 1.2 s/n: SUMD-ACEC-WXYL-LIWP-ENTF http://www.livepim.com
EZ File Transplanter 1.01.05 s/n: cAs41crE8r http://www.superwin.com
EZ Mozilla Backup 1.23 Name: TEAM ACME@none.de s/n: 0513813350 http://www.rinjanisoft.com
EZ ThunderBird Backup 1.23 Name: TEAM ACME@none.de s/n: 9402702249 http://www.rinjanisoft.com
EZDetach for Microsoft Outlook 5.0.1.178 Name: EMBRACE s/n: 1F1B2304D8 http://www.techhit.com
F
F.E.A.R. normal S/N: PUC9-MEW7-DAS3-BUJ9-2796 NEJ5-JYR6-ZER4-DAM3-5273 WEJ2-FAR2-GYZ4-REC4-5865 XAM2-PAS6-JUT5-SES8-5677 NER4-XAM5-WUR8-WAT8-7799 Funktionieren alle...Wink (null)
F1 Racing 3d Screensaver 1.0 S/N: User: (Your name) serial: fmJsDP5YvA8CFJEo http://www.digimindsoft.com
FantaMorph 1.0 S/N: Name:Ihr Name S/N:5701-6523-5660-4057-4113 Muss funktioniern da es eine orginale Seriennummer ist. mailto:chuclemens@yahoo.de
Fantasy Forest 3D Screensaver 1.0 S/N: Name : (Your name) Code : a72lidNp0yXjh3HK http://www.digimindsoft.com
FantasyDVD Player Professional 8.50 Name: alkavour S/N: email:alkavour@mycosmos.gr Order ID:EyyhJ7nMjU693 Product Key:RBdqBouThpcVd Activation Key:BCF32077269CC468510E1E7C0C064E6CAD059872 By Alkavour (10/01/2006) http://http://www.fantasysoft.net
FIFA 2004 1.0 Name: Renjith Varghese S/N: NPX7-X9EN-JD6B-RPF6-2WA4 J3CL-DMRE-FCQU-YX9X-9AU7 by renjith varghese (null)
FIFA 2005 1.0 Name: Renjith Varghese S/N: WDUU-AZV4-KS2Z-3DX3-KE2Q QTDD-ENH5-EXZ7-DMVT-D7UB by renjith varghese (null)
FIFA 2005 1.0 S/N: 2DWE-2DAS-ETLA-7KZ6-HRNZ (null)
fifa 2006 2006 S/N: vrtt - 7ex9 - kdev - xqng - qdev (null)
Fifa World Cup 2002 1.0 Name: Renjith Varghese S/N: 9767-3584462-4490770-2860 5382-3725294-3355164-1021 by renjith varghese (null)
File Compare & Folder Synchronization 6.0 S/N: 272097792 By Alkavour (29/01/2006) http://www.sobolsoft.com
File Mass Opener 1.10 Name: UnderPl s/n: 7fmo http://www.van-tilburg.info
Firma und Verein 2.1.256 s/n: 112093-9140260-201664 http://www.firma-und-verein.de
flash 8 professional 8.0 S/N: WPD800-59735-96732-30497 (null)
Flash SWF to AVI GIF Converter 1.4.10 s/n: swfavi20-1009 http://www.minihttpserver.net
Focus Photoeditor 4.1.1 Name: TEAM ACME s/n: 7556653746229649408 new-world-software.com
Focus Photoeditor 4.4.0.17 Name: TEAMHERETiC s/n: 2760939217542078464 new-world-software.com
Folder Guard pro 7.6 7.6 Name: Todor S/N: Number of Copies : 50 Serial Number : A6297590 (null)
Folder Indexer 1.61 s/n: FGD123SGgsdfgFD http://www.van-tilburg.info
Folder Security Personal 3.00.0135 Name: EGOiST S/N: ProdID:{73D9FBB7-9B65-5C17-9301-0E80E5CFA581} RegCode:{2DA508FD-E77C-B979-354A-0EEB944A403B} By Alkavour (07/01/2006) http://www.y0ys.com/
Folder Sizes 3.1.0.2 Name: freeserials.com S/N: 0000WQ-XDKVHP-GAYMN1-5UZ3MP-ZUYM3B-BNGMQX-V5DRY4 (null)
Font Fitting Room Deluxe 2.2.0 Name: Team ZWT S/N: wSMPLdV170zN8MCMN1XBj By Alkavour (10/01/2006) http://www.apolisoft.com/ffr/index.php
FontDoctor 2.0 s/n: GTY8qa439 http://www.morrisonsoftdesign.com
Forest World 3D Screensaver 1.0 S/N: Name : (Your Name) Code : U00xMHEjVdxnYp0K http://www.digimindsoft.com
FortiClient 2.0.148 S/N: 10cbc667a01cb01bd4d7b4355014cfcf6c (null)
FotoStation Pro 5.1 S/N: FSW5-E417-0000-0101-0000 (null)
Foxy 1.30 Name: LTB/CORE S/N: 01018339 By Alkavour (29/01/2006) http://www.2-power-n.com/features.html
Frame Explorer 1.0 S/N: AFHNBTEPHQKIXEEV snTRHAZXTRDUGMTHU sn:KQMFGPEPLSOBUTPK By Alkavour (15/01/2006) http://www.dl-c.com
FrameShape 1.06 s/n: FF0AAB4715961A80FF26 http://www.structureshape.com
FrameShots 2.2.4 S/N: 8B9E97AA9B9B79A557799EA5A437122 http://www.frame-shots.com/
FREND 4.8 Name: TEAM ACME s/n: FFwQl-5bAACA-Ev49-vCb55-Cv9 http://www.kernfranken.de
Fresh Diagnose 7.20 S/N: bg3d4c-5x3wh3n6-6f3e43 By Alkavour (07/01/2006) http://www.freshdevices.com/mainboards_test.html
Fresh UI 7.47 S/N: sc7s8s-6x2vw4s3-3v6f36 snq4i3a-3g6wp8b3-6f3f64 By Alkavour (10/01/2006) http://www.freshdevices.com/freshui.html
FreshDownload 7.22 S/N: User name: Ash Registration code: 2H9B4-Q849-UB48-8E7J (null)
Frontline Attack - War over Europe 1.0 S/N: WB8P-B5KR-CPY8-T7BQ (null)
Futuremark PCMark05 Advanced 1.1.0 Name: tam/CORE s/n: PCM05-134Q-8041-664D-6562-L2X6 http://www.futuremark.com
G
GameAccelerator ? S/N: 3266ba6e04fce2a42ad8de2892633bd612755e6915479ccc70 b56673e6eb6e73 Muss funktionieren da es eine orginale Seriennummer ist. mailto:chuclemens@yahoo.de
GameTuner 1.0.0.33 s/n: 105A5S-482YFS-KES7KS-Y0E5L5-R5ZXL0 http://www.s-a-d.de
GData AntiVirusKit 2006 Name: fischer2815 s/n: 7j1n1e http://www.gdata.de
Gene Troopers GAME S/N: KEY: JKWSM-YXPS8-N7A7M-QXUED-7RP2K (null)
GetIPDL 2.14.10 s/n: WDGFE-P5P6Q-9YXYH-S78B7-BCTPY http://www.ujihara.jp
GetRight 4.5 S/N: GETRIGHT-45TR (null)
GhostSurf 3 S/N: GH4X5X2K (null)
GIF Construction Set Professional 2.0.73a Name: TEAM LAXiTY s/n: 22261-09-21673-16 http://www.mindworkshop.com
GIF Construction Set Professional 2.0.74a Name: Alkavour S/N: 19835-24-19019-14 By Alkavour (05/01/2006) http://www.mindworkshop.com
Girokonto German 2005.10.73 Name: TEAM ViRiLiTY s/n: 57909297 http://www.oggisoft.de
google earth pro 3.0.6 S/N: JCPMF9VXRQQ6HBL (null)
GpsTools SDK 2.20l S/N: component:.NET for Windows snCn7lC1yoTmmWNJkw0GknusakBucci3ZoCl7 component:.NET for Pocket PC sn:5549EF79BB9AE784DA6A component:ActiveX for Pocket PC sn:B349EFA45DC60D415DC6 component:ActiveX for Windows sn:C549EFCA1E043132151E By Alkavour (24/01/2006) http://franson.com/
GraphicsGale 1.70 Name: GanJaMaN E-Mail: WEED@CORE.COM s/n: jXwZESwtO7gzXtFgWb9IO6tkuUHFRKHD6RGR0w== tempest-j.com
GraphicsGale 1.78 Name: alkavour S/N: email:WEED@CORE.COM sn:lnknJQ3A3JjhgcUAIIoQs8vNthTlh86YyatwAg== By Alkavour (07/01/2006) tempest-j.com
GraphicsGale 1.80 Name: GanJaMaN S/N: email:WEED@CORE.COM serial:jXwZESwtO7gzXtFgWb9IO6tkuUHFRKHD6RGR0w== By Alkavour (14/01/2006) tempest-j.com
Greeting Card Designer 3.0 S/N: TX37TPIP379FBF3P (null)
GS-Auswertungsdesigner 1.0.9.7 Name: Melborn [ACME] s/n: 11466207 http://www.sage.de
GUITAR STUDIO 4.8 Name: acme S/N: company: paranoici / olografix key: 2780556 (null)
Gutterball 2 2 Name: Lord Voldemord S/N: XT8CVHQAYCMSJNA http://www.gamehouse.com
H
habbohotel 1.6 S/N: 71r85gr2 (null)
HaneWin DHCP Server 2.1.5 Name: Team BLiZZARD s/n: BBLZ197031D91549 http://www.hanewin.de
Hangman Pro 1.05 Name: NiTROUS s/n: HPROP-39863 http://www.mv.com
Hangman Pro 1.08 Name: http://www.bg-warez.org S/N: HPROP-42258 By Alkavour (10/01/2006) http://www.mv.com
Harry Potter :Quidditch World Cup 1.0 S/N: TS9H-BA3T-2UCK-VEQC-PDTD 57YG-DGQZ-7JQZ-RZS6-H6XT by Renjith Varghese renjith varghese (null)
Harry Potter and Prisoner of Askaban 1.0 Name: Renjith Varghese S/N: 5CSJ-GRQG-7JQZ-PZS6-H6XT 7Z7X-XPDQ-YLUC-M5X9-5L9V 3LPX-TEDC-7FHB-WQJE-7FLM by renjith varghese (null)
Harry Potter And The Sorcerer's Stone 1.0 Name: Renjith Varghese S/N: 5380-3235151-5291052-6713 3603-5988003-2247511-4904 by renjith varghese (null)
health calc 4 S/N: 487234681 (null)
HealthFile Plus 3.2.6 Name: AGAiN tEAM s/n: 11953 http://www.wakefieldsoft.com
HeavyMath Cam 3D Webmaster Edition 2.7.0.51024 E-Mail: BRD@Cult s/n: SDD67ATE8STSDB6VE7W5FCWFCW http://www.heavymath.com
HelpBlocks 1.17 Name: Alkavour S/N: 5486B5E4-EB5D9E7D-A8E0C0FE By Alkavour (15/01/2006) http://www.helpblocks.com/
Hex Workshop 4.1.100.1332 S/N: 904A9-506213-A96E (null)
Hidden Camera 1.4 Name: 4kusN!ck S/N: FCGBCFEDFGAFGDGF Mail: 4kusNick@revenge-crew.com Quantity: 256 By Alkavour (29/01/2006) http://www.oleansoft.com/hiddencamera.htm
Hidden Recorder 1.7 Name: DIGERATI E-Mail: DIGERATI@DIGERATI.com s/n: HADMMCEHHBJMLJL http://www.oleansoft.com
Hide IP Platinum 2.3 S/N: A70B5854DA4D74A6 By Alkavour (07/01/2006) http://www.hide-ip-soft.com
Hide IP Platinum 2.31 S/N: C741757E29FC5DC1 sn:80055378BDCE3E9E sn:FA65D8092D961427 By Alkavour (14/01/2006) http://www.hide-ip-soft.com
HideAll 2.04 S/N: 1AH10S3BVT2901 By Alkavour (10/01/2006) http://www.sgssoft.com
HIPAA Training Program 2.0 s/n: HIPAATRNGREG2005 http://www.reg-software.com
HistoryWasher 1.04 S/N: 9S8H9I1V928911 By Alkavour (10/01/2006) http://www.sgssoft.com
HOAI- und VOB-Mustervertraege und -briefe ArchIng 09.2004 s/n: 728395ED http://www.weka.de
Home Data Deluxe 7.8 s/n: HOMEDATAREG2000 http://www.reg-software.com
Home Inventory Plus 5.2 s/n: HOMEINVREG2000 http://www.reg-software.com
HOMEWORLD 2 Ver.1 Name: HOMEWORLD 2 S/N: NAF5-XAC6-WYJ3-JEG2-8775 Name Any, Serial Unused, UnReg. http://www.sierra.com
Hope Mailer Standard Edition 1.16 Name: NiTROUS s/n: MLESTD-72BB6690BDDB356D41 http://www.nesox.com
Hot Corners 2.21 Name: DEVOTED s/n: 1152-10566-1497-301 http://www.southbaypc.com
HotMail+ 1.3 S/N: 155001237750184 By Alkavour (10/01/2006) http://www.hotmailplus.net/
HttpWatch 3.2.0.47 Name: TEAM EMBRACE s/n: 832719240 http://www.simtec.ltd.uk
HttpWatch 3.2.0.65 s/n: 843310024 http://www.simtec.ltd.uk
Hyena German 6.5 Name: TEAM ZWT s/n: 1ieh-4ja0-1gj3-2585 http://www.systemtools.com
Hyena 6.5 Name: TEAM ZWT s/n: 1ieh-4ja0-1gj3-2585 http://www.systemtools.com
I
IA eMailServer Corporate Edition 5.3.2 s/n: SAUN-09-B32BC4656BD969D7-05 http://www.tnsoft.com
IA eMailServer Corporate Edition 5.3.3 s/n: SAUN-09-4E99420364C7D1EB-05 http://www.tnsoft.com
Icon Extracter XP 3.2 Name: TEAM ViRiLiTY s/n: 11110101000101010100010001001010010110011010100100 10010101011001010100100101010110010001011001010101 11110101000100011111 http://www.casepro.de
idImager 2.6.1.6 s/n: O-064381795-ZM-27759 http://www.idfoxx.com
IDo Wedding Couple Edition 7.5.0.2254 s/n: NG6I3 MR8U9 JI3D2 IE5A5 http://www.elmsoftware.com
IDOS J??zdn?? R??dy 2005/2006 S/N: archiv: BAM1984BY S/N: CX4-YBA-6EF4 CXB-76N-DC5B CXH-4JA-DD28 http://www.chaps.cz
IE FTP Enhancer 1.4 s/n: AEAX2R4Y43-94BH6VK67W http://www.stevefoxover.com
IFufi2 2.0.0.0 Name: http://www.bg-warez.org S/N: Email: crackteam@bg-warez.org SN: V41T-4TQ4-O07P-5OM3-I83G-7KA4 By Alkavour (05/01/2006) http://www.giber.si/d/ifufi2_setup.exe
Import Wizard 8.2.0e S/N: license type:regular license number of licenses:99999 name:embrace it bitch sn:AYDJVCJLS3IW8A99999:embrace it bitch or license type:
ImToo DVD Audio Ripper v2.0.55.801 S/N: CBE2-A0E0-6D29-6ED9-66CD-A278-197D-3A55 (null)
ImTOO MP3 WAV Converter 2.1.41.1025 Name: TEAM LMi s/n: 2935-0381-9952-2329-F452-D276-F5FD-8CF6 http://www.imtoo.com
ImTOO PSP Video Converter 2.1.55.1025b Name: TEAM LMi s/n: 2935-0381-9952-2329-3D90-B8E1-DD95-CC8E http://www.imtoo.com
ImTOO WMA MP3 Converter 2.1.41.1025 Name: TEAM LMi s/n: 2935-0381-9952-2329-2D1E-3C31-8049-006D http://www.imtoo.com
InfoSafe Plus 3.4.6 Name: AGAiN tEAM s/n: 12898 http://www.wakefieldsoft.com
Installer Design Studio NET 3.0 S/N: DEV368307486321496275 SN: DEV368307448360388334 SN: DEV624563599641677742 By Alkavour (23/01/2006) http://www.scriptlogic.com
Installer2GO 4.1.3 Name: tam/CORE mania s/n: 11MFISH8Z1 dev4pc.com
IntelliTipster 1.5.1.1770 Name: TEAM BLZ E-Mail: BLiZZARD@0day.net s/n: 189A-978F-0753-B9CE http://www.intellitipster.com
inter key 1.1 1.1 S/N: papip41a-0000001648-09320-7122 (null)
Inter Video Win DvD 7 7 S/N: Mail: COREMANIA@CYBERJUNKIE.COM / Password: CORE / License N*: VYECEXAD-43J54RT2-WEFMLZK5-LZVZK5HJ / CB Number: 2222 its funct http://www.intervideo.com
internet download manager 5.01 Build 2 (DEC/21/2005)full S/N: Y30OZ-DNQH7-FRWPW-YYZMA (null)
Iolo Search and Recover 3.0 Name: HERETiC s/n: 50935-R3441-5727961011 http://www.iolo.com
Iolo System Mechanic Pro 6.0h Name: HERETiC s/n: 89881-P6091-0776466900 http://www.iolo.com
ipTicker 1.9h Name: TEAM BLZ E-Mail: BLiZZARD@0day.net s/n: 4BC0AL88D2F6ZC1D http://www.intellitipster.com
iRadio 1.5.0.512 S/N: hardware key:37C-E68-303-235-4A3-230-30 license key:FE1F51EAB280EDA371BE By Alkavour (14/01/2006) http://www.3alab.com/
iSilo 4.29 S/N: KJNX9TKNP4TG94U7 http://www.isilo.com/
ISMail EP 3.3.65 S/N: 372A645F6FAC34E3 sn:4D99FA0CC866FCC5 By Alkavour (10/01/2006) http://www.instantservers.net/
IsoBuster Pro 1.903 S/N: Email: crackteam@bg-warez.org ID: crackteam@bg-warez.org_JMBWTEXMDXKFUPCQYOI Key: 6C9933F7-EC964610-3C04110C-073E65F9-605E470C-341304C8 By Alkavour (05/01/2006) http://www.zen16305.zen.co.uk/isobus...r_all_lang.zip
ISOpen 3.2.5 Name: TEAM CAT 2005 s/n: ISP325-2100110415606933616737143050434885200489654246413 koyotstar.free.fr
ISS BlackICE PC Protection 3.6 cot Name: tam/CORE S/N: 1677790-RS-A4532 By Alkavour (07/01/2006) http://www.iss.net
ISS BlackICE PC Protection 3.6 cox Name: alkavour S/N: 3028290-RS-A4645 By Alkavour (14/01/2006) http://www.iss.net
ISS BlackICE PC Protection 3.6.coq Name: tam/CORE s/n: 1677790-RS-A4532 http://www.iss.net
ISS BlackICE Server Protection 3.6.coq Name: tam/CORE s/n: 16777A2-RS-E4F2E http://www.iss.net
izotope ozone 3 3.08.527 S/N: IZ-OZONE3-E1732ABB-CD97 (null)
_________________
Ilmu Akan Bermanfaat Bila Membiasakan Untuk Saling Berbagi.
James Bond 007 Nightfire 1.0 Name: Renjith Varghese S/N: 1814-2249945-2284603-2559 2358-7276712-2855463-0485 by renjith varghese (null)
JesCopy 3.46 Name: BLZ-TEAM s/n: 0006700C4BL0060Z09F0J32F03CF04AF01DF044F035F031F03 DF000F300F600F300F600F300F61DF010F044F035F031F03DF 010F032F03CF059F04AF04AF031F042F034F010F022F020F02 0F025F010F01DF05550CF06662CF076F075DF0BLZ software.thomasjacob.de
JinJon (all versions) All Name: Mher Harutyunyan S/N: A8BH-3DV2-3DLR-WABN P8BH-3BV2-4DSR-6ARE C8BH-MBV2-4DSR-KAVJ B7BH-3BV2-2DSR-6ARK S8BH-MBV2-2DSR-MAVF L6BH-3BV2-2DLR-YARW Q8BH-MBV2-2DSR-8AV2 Enter the kode and join! http://www.mher1991.narod.ru
JsAutoStart Pro 1.34.103 Names: TEAM; NiTROUS; 2004 s/n: 1139-008j6h2h-08f228nd-0501d2d0-4968 http://www.tiosoft.de
JSFactory PopUp 4.0 s/n: 7o2x3a8i yaldex.com
JSFactory Pro 2.0 s/n: CG6UI-XHDY5 yaldex.com
JSPMaker 1.0.1 Name: alkavour S/N: 0971ACDD20446183 By Alkavour (29/01/2006) http://www.hkvstore.com/jspmaker/
JSPMaker 1.0.1 Name: alkavour S/N: 62E5B220C97097B9 By Alkavour (15/01/2006) http://www.hkvstore.com/jspmaker/
July20th Dynamic Range Workshop for Adobe Photoshop 1.2 Name: TEAM SCOTCH s/n: 0c8d2b31a85bcd238975acf3cf476608 http://www.july20th.com
Jungle Heart 1.0.1 s/n: 99999999999999999999999999999999890978 http://www.elephant-games.com
Just Checking 3.02 Name: d/TMG s/n: 7774045-36890400-2839809 justapps.com
Just Money 1.13 Name: d/TMG s/n: 7775610-51163200-2840122 justapps.com
K
K Database Magic 1.0.5.0 Name: AGGRESSiON s/n: 197191B2-68E55DC0 kdbmagic.uw.hu
KaraWin Std 2.3.0.0 B221005 Name: HERETiC s/n: 93C8C711 http://www.karawin.fr
kaspersky S/N: 5.0.390 S/N: 0038-00006b-000de784 (null)
KC Softwares K-FTP 4.4.489 Name: NiTROUS s/n: 60085094092083086106091 http://www.kcsoftwares.com
KC Softwares K-MAIL 4.2.289 Name: NiTROUS s/n: 60085094092083086106091 http://www.kcsoftwares.com
KC Softwares PhotoToFilm 1.4.2.45 Name: BRD s/n: 90073103076 http://www.kcsoftwares.com
KeyPass Enterprise Edition 4.0.2 S/N: KEYC-N33E-9ST2-NPFN-TUKK-59G3-USTR-FRFS-EUG3 SN: KEYC-SEFM-3HUK-HM5T-NKHM-U3EF-MFFH-25GT-RTE5 SN: KEYC-FRUN-GTFF-URMS-GRSM-3RR5-9FNR-U2FM-HHS5 By Alkavour (24/01/2006) http://www.dobysoft.com/products/key...pass-setup.zip
Keystroke Converter 4.7 Name: CRUDE s/n: C-40254988YJOOTNLHICRUDERGPRHPWRCQYZJSZZ softboy.net
K-FTP 4.5.493 Name: seeker02 [pHrOzEn-HeLL] s/n. 21090090077076076103048050032112088073089100098070 085045080070083097101 http://www.kcsoftwares.com
Kingdia CD Extractor 1.0.6 Name: BLZ-TEAM s/n: B405A9436BF7B3AAB45A556F1F2B0C4 http://www.kingdia.com
Kingdia DVD Audio Ripper 1.3.8 E-Mail: TEAM ViRiLiTY s/n: 2738A968F185F000EB8BFCE090D7A20E http://www.kingdia.com
Kingdia DVD Ripper Professional 2.1.8 E-Mail: TEAM ViRiLiTY s/n: 2EF5B14DA46C46C1D19D19EECEF499A9 http://www.kingdia.com
KIYUT Sketsa SVG Graphics Editor 3.2.2 Name: GeFcReW s/n: 2133-466788-86143-71137-16529 http://www.kiyut.com
KLS Backup 2006 Professional 1.8.0.1 Name: TEAM SSG S/N: KLSBLICENSEPRO2D7C466FAFDC59CE64A4973E24040BA71 By Alkavour (15/01/2006) http://www.kls-soft.com/klsbackup/index.php
K-ML 3.19.326 Name: seeker02 [pHrOzEn-HeLL] s/n: 21090090077076076103048050032112088073089100098070 085045080070083097101 http://www.kcsoftwares.com
K-MP3 5.11.3.98 Name: TEAM ViRiLiTY s/n: 91091090073078032107081083080097081085096 http://www.kcsoftwares.com
K-MP3 5.12.0.100 Name: TEAM ACME s/n1: 20091090073078032086075078076 s/n2: 21091090073078032086075078076 http://www.kcsoftwares.com
Kofax Capio 1.52 S/N: E794-7030-A3AA-DF34 By Alkavour (24/01/2006) http://www.kofax.com/
Kreuzwortraetsel-Werkstatt 3.6 Name: Kent s/n: ZUUCCCVVC4 http://www.scotandrews.com
Kristanix Password Generator Pro 1.4.8 s/n: KRX-262-X4802528684585590188932786462092 http://www.kristanix.com
Kristanix Right Click Image Converter 1.6.2 s/n: KRX-642726923947011105446583971405113038 http://www.kristanix.com
Kristanix Yatzy 2.3.5 s/n: KRX-832960535702477780278134436177350075 http://www.kristanix.com
KsL Software Reigstry First Aid 3.4.0.541 Name: TEAM High Society s/n: 2FB622-18A45DE5-BB5232FA-58EAE58F-139E54 http://www.rosecitysoftware.com
Kyodai Mahjongg 2006 v1.0 final S/N: Name: Agent Smith Serial: 98416852410 (null)
Kyodai Mahjongg 2006 1.0 Name: THOR_MESTER S/N: 66117262731 by_THOR_MESTER REG: SPACE+F9 http://kyodai.com/kyodown.en.html
Kyodai Mahjongg 2006 v.1.0 Name: THOR_MESTER S/N: 66117262731 http://kyodai.com/kyodown.en.html
L
La Chouine 1.51 Name: TEAM NGEN s/n: 19418 stcras.free.fr
Label Designer Plus DELUXE 8 S/N: 0665-E60B http://www.camdevelopment.com/designer/label_deluxe/
LAN-eMail Pro 2005.2.1135 "Name: TEAM ViRiLiTY s/n: 72136404 Note: use 9 digits for ""Rechnungsnummer""" http://www.oggisoft.de
Langenscheidt T1 5.0 S/N: 016999787 Englisch - Deutsch Deutsch - Englisch ??bersetzungsprogramm http://www.langenscheidt.de/deutsch/...ien/t1_v6.html
Lasha 2006 Name: Lasha S/N: 765146 (null)
Lavavo CD Ripper 3.15 Name: Team Cafe s/n: 1A3E7E71FF62EF6A http://www.lavavo.com
Lavavo DVD Duplicator 1.02 Name: Team Cafe s/n: 34CDD3F1FC176638 http://www.lavavo.com
Lavavo DVD Ripper 1.0 Name: Team Cafe s/n: 36005FD07EBAF8FC http://www.lavavo.com
Lavavo DVD Ripper 1.1 Name: BLZ-TEAM s/n: 25734A096D12726E http://www.lavavo.com
LB Workshop 4.3.0 s/n: 68720717 alycesrestaurant.com
LehrerOffice 2004.12.9.0 Name: TEAM ViRiLiTY s/n: VBXG-LXNM-CCCC http://www.rothsoft.ch
LehrerOffice 2005.1.0.0 Name: ACME s/n: UZRVLGBOGYEQ http://www.rothsoft.ch
LehrerOffice 2005.13.6.0 Name: TEAM ViRiLiTY s/n: ANXGLEAYLNLF http://www.rothsoft.ch
Leonardo Express 1.6.0.36 S/N: wra39cttkqcykx36 LeonardoExpress von Hermstedt um mit normalen ISDN-Karten vom PC aus zu Hermstedt Karten im Macintosh Verbindung auf zunehmen. (null)
LesefixPRO 5.12 Name: TEAM ViRiLiTY s/n: 1212-D612-1315-1618 http://www.kinderleichtsoftware.de
LigaChampion 7.0.9 Name: PV27285 s/n: 64723 http://www.wido-software.de
Lite Photos 1.2 Name: 4kusN!ck S/N: LGDHDDIQCEMGHIQN Mail: 4kusNick@revenge-crew.com By Alkavour (29/01/2006) http://www.oleansoft.com/litephotos.htm
LMSOFT FTP Site Manager 1.0.0.1 s/n1: 0120-0018-79 s/n2: 0455-0219-11 http://www.lmsoft.com
Loan Advisor 1.07 Name: DiGERATi s/n: 31317461 http://www.othersoft.co.za
LoanExpert Plus 3.2.6 Name: AGAiN tEAM s/n: 09973 http://www.wakefieldsoft.com
LoHice 2.3 S/N: 25646652256635854347864135764365165 (null)
Lotto007 XP 2005 6.0 Name: 1537-3003 s/n: 3038-1887-3088 http://www.lotto-007.com
M
M2-edit Pro 5.0 s/n: 24zk ThFU qTEf 2BXz PpAY 8QZF http://www.mediawaresolutions.com
Macro Mania 10 Name: TEAM@LUCiD.COM s/n: 88547151011 http://www.nstarsolutions.com
Macro Mania 10.2.1 Name: BRD Cult s/n: 88JG7161005 http://www.nstarsolutions.com
Macromedia Coldfusion MX Enterprise Edition 7 s/n: CED700-41965-74353-12291 http://www.macromedia.com
Macromedia Dreamweaver 8 8 S/N: WPD800-54232-80332-94711 http://www.marcomedia.com
Macromedia Flash 8 Professional 8.0 S/N: WPD800-59735-96732-30497 WPD800-51937-56932-99728 WPD800-50836-44132-84176 PFD800-51945-73748-42725 PFD800-50844-09148-22946 PFD800-57949-38748-01307 PFD800-50844-06948-81405 PFD800-52644-69448-72841 PFD800-57543-37248-22825 PFD800-59745-98848-61 http://macromedia.com
Macromedia Flash 8 8.0 S/N: WPD800-57137-56432-75244 WPD800-51135-12332-86296 WPD800-57531-71132-10762 http://macromedia.com
macromedia freehand mx11 espa??ola S/N: S/N: fhm110-02280-87210-08484 (null)
Magic DVD Ripper 3.5d Name: TEAM ViRiLiTY s/n: 3JA2403O1A8N90INBM20WJ9DGGLRVV http://www.magicdvdripper.com
Magic DVD Ripper v3.6 Name: THOR_MESTER S/N: C6Cg30OYsnK7CT3cf26ZjI by___THOR_MESTER http://www.magicdvdripper.com
Magic Recovery Professional English 3.2 s/n. 2201-3719-1214-5827 http://www.ondata.es
Magic Recovery Professional Spanish 3.2 s/n. 2608-6218-1955-2652 http://www.ondata.es
MahJong Suite 2005 2.10 Name: TEAM BLZ s/n: E4339B36-B4E8D7EA-C5B9AE2C http://www.mahjongsuite.com
Mail Melder 1.1.5 Name: TEAM iNFECTED2oo5 s/n: iNFECTED-6F548850-4BA63416-303C http://www.tssev.de
MainConcept H.264 Encoder 2.0.15 S/N: AVC-TEAM-ZWT-CRACKING By Alkavour (24/01/2006) http://www.mainconcept.com/h264_encoder.shtml
MarkAble 1.4.0 Name: End-User s/n: 0543-2145-6951-1000 http://www.ipodsoft.com
Marksman 1.0.2 Name: End-User s/n: 0532-2134-6940-9893 http://www.ipodsoft.com
McFunSoft Video Solution 3.66 S/N: MVS5945630 By Alkavour (12/01/2006) http://www.enfull.com/download3.asp?id=1332&soft=down
MDE Info Handler 8.16.1 Name: TEAM FALLEN Company: TEAM FALLEN s/n: NF-84059-48354.33180 http://www.mdesoft.com
Medal Of Honor :Spearhead 1.0 S/N: 1119-2468399-8009944-2524 5141-8894801-7071263-0429 2500-9611912-8344207-1325 5654-3599075-7956405-0822 Enjoy (null)
Medal of honor Allisd Assault 1.1 Name: Renjith Varghese S/N: 5654-3599075-7956405-1867 8957-3996266-2473168-0751 Enjoy 100% working key (null)
Medal of Honor Pacific Assault 1.1 Name: Renjith Varghese S/N: 3AEN-8CDT-7FHB-FQJE-7FLM 96C4-ZMVX-KW58-28S6-J9QH PBLL-FPWS-ZP93-TWNL-ZMC9 by renjith varghese (null)
Medal Of Honour Pacific Assault 1.0 S/N: FGPX-Y3NH-4FUN-RGTI-G0IH DXNE-N2PL-ZJXC-9ZZ4-PQGJ BMXP-JXZE-2NX4-5EQX-89UT 100% working (null)
Media Monke 2.4.2.874 Name: http://www.serialnews.com S/N: V24FA55VAUUL8M6Z9SJ89PE (null)
Mediafour MacDrive 6.05 Name: Team ArCADE 2005 Company: ArCADE s/n: MD6-EWR4G-QHRHD-WEG http://www.mediafour.com
Mes Bases de donnees Multimedia French 1.0 s/n: MBD01-U4LN1-98NJ7-XK4WS http://www.oriasoft.fr
MessageSave for Microsoft Outlook 3.0.0.133 Name: EMBRACE s/n: 3175702A48 http://www.techhit.com
MetaProducts TrayIcon Pro 1.5.190 Name: snatch s/n: dqmaAejqQ3R5AjN9HGT0Hi2RSxgM+rVz6pXCBM8c3uaIRbvIYd JDcjAYGvsxgDeNYIc=amqd http://www.metaproducts.com
Microsoft Windows 2000 Pro sp4 Fran??ais sp4 S/N: PQHKR-G4JFW-VTY3P-G4WQ2-88CTW (null)
Microsoft Windows Vista Beta 2 Build 5270 S/N: R4HB8-QGQK4-79X38-QH3HK-Q3PJ6 (null)
Microsoft Windows XP Professional Edition Corporate SP2 S/N: YQ7XW-QPT6C-233QF-RRXC7-VF7TY S/N: X4PTJ-6WP7J-BFVCY-WYDJT-DMDDK S/N: 3PX6Y-7HTR8-4PVPQ-PYFGK-GRK3P S/N: 3FC6J-PVRYV-38KFF-B2K46-CDHH6 S/N: PM732-VDYT8-27YHH-QMMB7-CXPYW http://www.microsoft.com
Microsoft Word 2000 S/N: HPHW2-9JMXY-BG4CB-Q2FTX-R66TG http://www.microsoft.com
MIDI Tracker 1.1.0 Name: Nitrogen / TSRh s/n: VupL-edzZ rf1.net
MIDletPascal 2.0 Name: TEAM TBE s/n: 15RvhB9sHXkA6BE http://www.midletpascal.com
MightyFax 3.15 Name: VRL ROCKZ s/n: RKS-4422683 http://www.rkssoftware.com
MightyFax 3.3 Name: d/tmg S/N: RKS-2427607 By Alkavour (14/01/2006) http://www.rkssoftware.com/mightyfax/
Migrate Easy 7.0 S/N: English sn: 3BGAG-7BHM9-3JAN2-V2EUM-QHHJM German sn: JCM44-SJSZQ-XQHT2-MAEK2-JAD85 Russian sn: BSADB-J7X6B-HTEZM-QPLEX-9NSPM French sn: WUJ63-WZNHA-P6Y5E-ZUM3R-SJLP5 Czech sn: PY45D-BN7LW-J67CU-EJNJK-BPT3A By Alkavour (05/01/2006) http://www.acronis.com
MindManager Pro 6 6 S/N: MP61-935-MP79-2A85-1CA3 http://www.mindjet.com
Mindscope Software FingerPaint 1.1 s/n: 74134447941 http://www.mindscopesoftware.com
minimarket Express Private Edition S/N: ke32zt75nx188 (null)
MiShell Budget 1.0c s7n: LeX1O9u1R5S3 http://www.mishell.ca
mks antivirus 2005 S/N: 89VP-GT2W-X6JS-1LWT-R5R1-1KL1 (null)
MMS Soft 6.0 s/n: 001-MMS-400186-TJBF7L http://www.hasenbein.de
Monster Fair 1.2 S/N: E-mail: www@crackzplanet.com Serial: 772275131552730131952802 (null)
Mootools 3D Photo Browser for 3D Users 8.31 s/n: 6351-DCA4-048D-66B7C http://www.mootools.com
Mootools 3D Photo Browser for Digital Camera 8.31 s/n: 971A-B9CE-A81E-72A38 http://www.mootools.com
Mootools 3D Photo Browser Light 8.31 s/n: 859C-4C13-337D-8707A http://www.mootools.com
MooTools Polygon Cruncher for 3D Photo 6.7 s/n: 7F51-31D6-FCF1-8E300 http://www.mootools.com
MooTools Polygon Cruncher for 3DSMAX 6.7 s/n: 50E1-FC93-1763-A7CDF http://www.mootools.com
MooTools Polygon Cruncher for Lightwave 6.7 s/n: 4F5A-623B-062C-48A40 http://www.mootools.com
MooTools Polygon Cruncher 7.0 S/N: For 3d Photoshop:7B15-7431-B9B1-C9184 For 3DSMAX:5E0F-1227-F889-C9051 For Lightwave:4287-BF80-DAF5-0C02D By Alkavour (14/01/2006) http://www.mootools.com/
MooTools PolygonCruncher for 3DS Max and Lightwave 6.6 s/n: 5baa606b5334a6025 http://www.mootools.com
MooTools RC Localize for Windows 3.0 s/n: a18ad65d440599814 http://www.mootools.com
Move My Printers 3.1.0 s/n: SKU3321-23187DWS-ed32d5 http://www.movemyprinters.com
Movie Jack 3.5 VCD 3.50.510 s/n: 32V07-96PZC-52A75-WQZ11-A72ZD http://www.s-a-d.de
Movie Jack DVD 2 2.04.001 s/n: A25UT9-5376K4-3V30PQ-Q2Q74W-Q47VSD http://www.s-a-d.de
Movie Writer Pro 2.79 s/n: GAVJ-MBAF-JUDU-FCAA http://www.reynes.org
MP3 Alarm Clock 1.6 Name: TEAM LAXiTY s/n: H6T4081587D3 http://www.wisecoder.com
MP3 Burner The Simple Way 2005 6.1.0.415 Name: tam/CORE s/n1: 379340380 s/n2: 924B1E8B2100 http://www.net-burner.com
MP3 CD Convertor 4.20 Name: EMBRACE s/n: 3vlFSDGCASViFCHS http://www.mp3-cd-converter.com
MP3 DJ 7.9.0 E-Mail: team@tbe.org s/n1: 4020 s/n2: 54941051 http://www.mp3-dj.net
MP3 PowerEncoder windows xp S/N: ME1M343882k98287 (null)
MP3 To Ringtone Gold 3.16 Name: LTB/CORE S/N: 298368-1aa5 By Alkavour (10/01/2006) http://www.ddz1977.com/
mp3player 3.50 S/N: 353387001798788 http://www.viking.tm
MP3Producer 2.40 E-Mail: TEAM@ViRiLiTY.com s/n: 04-112433-80907554 http://www.mp3developments.com
MP3TagEditor 2.04 Name: Mad^Agent S/N: 02-66017-80907554 http://www.mp3developments.com/
mp640 1 S/N: 1000002806 (null)
MPEG Video Wizard 2005 S/N: MVW-MPEG2-23EVQTSRGKLHYK (null)
MPEG-2 Decoder and Streaming Pack 3.0 S/N: F9E6BD862A76A8C8BF9D5FF91FE41D1F SN: 3FDF865196D74D375FFED956565F5603 SN: C652A9E6530022FFFF27A38BEDA320D0 By Alkavour (23/01/2006) http://www.elecard.com
MSN Checker Sniffer 1.0 S/N: 4BB66E176A5B7798 sn:C0225A4410359CD2 By Alkavour (07/01/2006) http://www.msn-tools.net/
mst Defrag Home Edition Win2KXP 1.5.26.49 Name: tE Company: tE s/n: DFRHDEF2E6E615D8F3053489FFF81EE87B71C2039D3B http://www.mstsoftware.com
mst Defrag Server Edition WinSkSRV 1.5.26.49 Name: tE Company: tE s/n: DFRS70AE740015D8F305F5DADBDC1EE87B718B36D7D0 http://www.mstsoftware.com
mst Defrag Workstation Edition Win2KXP 1.5.26.49 Name: tE Company: tE s/n: DFRW1F79806015D8F305BC2514A11EE87B716B28EB0C http://www.mstsoftware.com
mst Defrag Workstation Edition 1.8.30.58 Name: alkavour S/N: DFRW9379BDDCBBE1C541795AAEBED50157521E39F43A company:home By Alkavour (16/01/2006) http://www.mstsoftware.com
Multi Edit 9.10.03 ~ 9.10.04 S/N: Serial Number: ME91-MS315132430 Release Code: 21B4-E0E8-C895-378C http://www.multiedit.com/downloads/u...SetupMe910.exe ftp://ftp.multiedit.com/upgrade/SetupMe910.exe http://www.multieditsoftware.com
Multisim 8.0.4.5 S/N: Education : F4CG-2257-2488-9001-99640 Professional : F4CG-2148-8610-9001-19620 Power Pro : F4CG-2028-4010-9001-69646 Special : F4CG-2551-3040-9001-89768 all should work (null)
Music-DVD on CD and DVD 1.0.1.4 s/n: DCLFKY-VSS7RB-673LNU-9MLBLL-2SPNSM http://www.s-a-d.de
My Digital Photos 1.02 s/n: HiDetHoUme http://www.superwin.com
My Notes Keeper 1.41.461 Name: alkavour S/N: 495AD214AF1D41E5 By Alkavour (24/01/2006) http://www.mynoteskeeper.com/
My WinPopup Express 2005.01 Name: Team@DigERATi s/n: 123A000000020000 http://www.namtuk.com
MySQL Front 3.2.10.6 Name: http://www.bg-warez.org S/N: oKHGgdJ5OUiwJty4l+EEJj1seNWjYhNbW nWZ8sFWpSXNOMfnH6QNa+HG6WBhAesX4b FtF+27aLVUpW2Rs6evOoC7Xui64+40Tkd r+2e7QROkCUEAJIIrjSeVBbX7bZOFLy0P IRj6WdStaU4Ix5c6epTcEV2QyP3aky980 UnM5FrTKJdKzo0e5d4aZh7vAnJ4qxD/oh 6hBS5i4+Hsf5aBN94UAnULlTjGl3pPkuu qK3oP6MW3i By Alkavour (05/01/2006) http://downloads.chesnetweb.com/MySQL-Front_Setup.exe
N
Napis Adresu 1.0.1 Name: rG s/n: 18PRH53100-999 ussoft.hyperlink.cz
Natso Backup Pro Workstation 4.21.3.20 S/N: 9AJ6EG-2866TJ-A82ZCG-KAJZP2-C9AGAX SN: 9AJ6QX-QGXQZK-J8K22X-6QTQPZ-9C868Q SN: 9AJ62A-GZKAZC-CQXGA8-ZP6JQJ-T9SGSX By Alkavour (24/01/2006) http://www.natso-backup.com/download...orkstation.exe
NBA Live 2004 1.0 Name: Renjith Varghese S/N: QMVM-8HNX-F4BN-Y5GB-E3JP 3GAS-SWQD-48YW-MN3B-F2U8 by renjith varghese (null)
NBA Live 2005 1.0 Name: Renjith Varghese S/N: ES5V-RQDG-YSB5-S6HT-RZ7T W4MM-PWY8-Z5E5-DYPV-3T7B QQCL-PTNU-F4BN-X5GB-E3JP by renjith varghese (null)
Need for Speed "Most Wanted" 1.0 S/N: EXYQ-74C3-FESA-VU75-Z2DF (null)
Need For Speed Hot Pursuit 2 1.0 Name: Renjith Varghese S/N: 3Z4Q-HQUP-678C-PQQX XX6D-MPKB-G8QG-YAYG (null)
Need For Speed Underground 1.1 Name: Renjith Varghese S/N: XEFX-3855-YZ4L-GNR5-ACAU YQQQ-NCH2-QX9V-FMUC-HNTT by renjith varghese (null)
NemaTalker 1.9.2 Name: TEAMCORE s/n1: 11fI1249 s/n2: 87A7509F http://www.sailsoft.nl
NeoN Reminder 1.1 Name: Name: Bauer Lindemann s/n: WYUjcmAOUg3g5Ys http://www.neon-labs.com
nero 7 essential 7.0.1.4b S/N: 5C82001080100000000073825114 (null)
Nero 7 Premium 7.0.1 Name: Renjith Varghese S/N: 1C80-4091-8086-0000-8185-9145-9396 5C03001080100000000282593471 5C01001080100000000121316811 5C80001080000000008666563859 by renjith varghese 5C01001080100000000121316811 (null)
Nero 7 7.0.1.4b S/N: 1C80-00EE-19E5-MA2X-4003-414M-A71C Should work on all versions of Nero Burning Rom 7 Even new ones that come out. If the version number starts with a 7 (7.x.x.x) this serial should work for it. This is the premium version serial. http://www.nero.com
Nero Advanced Audio Plug-In 7.0.1.2 S/N: 5C03001080100000000089576725 http://www.nero.com
Nero Burning ROM v6.6.0.18 S/N: 1A21-0809-9030-2431-4539-4160 (null)
Nero DVD-Video Plug-In 7.0.1.2 S/N: 5C02001080100000000044871419 http://www.nero.com
Nero Essentials Edition 7.0.1.2 S/N: 5C87001080100000000180670433 http://www.nero.com
Nero Express 6.6.0.8 S/N: 1A20-030E-0010-2274-5129-6507 http://www.nero.com
Nero MP3 Pro Plug-In 7.0.1.2 S/N: 5C00001080100000000141238901 http://www.nero.com
Nero Multichannel Plug-In 7.0.1.2 S/N: 5C01001080100000000118952272 http://www.nero.com
Nero OEM SUITE 6 S/N: 1A20-0100-0000-1589-6412-2958 (null)
Nero Premium 7.0.1.2 S/N: 5C80001080100000000848434804 http://www.nero.com
Nero Premium 7.0.1.4 S/N: 5C80001080100000000474189603 http://www.nero.com
Nero Ultra Edition 7.0.1.2 S/N: 5C82001080100000000155698621 (null)
Nero Ultra Editon 7.0.1.4b S/N: 5C82001080100000000500115434 mal nen key der wirklich funktioniert! (null)
Nero Vision 3.1.0.11 S/N: 1A21-0609-4030-2329-6358-9615 (null)
nero 7.0.1.4b Name: chaser S/N: 5C82001080100000000073825114 it works (null)
Net Control 2 4.15.4.1.5.65 Name: Team EXPLOSiON s/n: KEibeIxBGiCXmYEKIFGEVEEgEpZaVHQXLszbcfweyklwCwfzXT UyHgOvokIEdQ http://www.netcontrol2.com
Net Monitor For Employees 2.4.3 Name: DIGERATI s/n: 9727817D2A2EA2B751999993A4 http://www.eduiq.com
Net Profile Switch 4.11 Name: TEAM ViRiLiTY s/n: KYHKGX886N2PJW3S http://www.jitbit.com
NetObject Fusion 9 S/N: NFW-900-R-666-14503-23526 (null)
NetObjects Fusion 7.5 S/N: Passwort f??r die Installation des Programms: F75CM Zur Aktivierung des Programms ben??tigen Sie eine Seriennummer, diese erhalten Sie nach der Registrierung beim Hersteller, ??ber den folgenden Link: http://www.netobjects.com/computerbild (null)
NetSarang Xmanager CLS Class A 2.0.0505 s/n: 060101-410241-000600 http://www.netsarang.com
NetSarang Xmanager CLS Class B 2.0.0505 s/n: 060101-520401-000840 http://www.netsarang.com
NetSarang Xmanager CLS Class C 2.0.0505 s/n: 060101-530481-000080 http://www.netsarang.com
NetSarang Xmanager DLS 2.0.0505 s/n: 060101-450331-000480 http://www.netsarang.com
NetSarang Xmanager Educational 2.0.0505 s/n: 060101-160161-000184 http://www.netsarang.com
NetSarang Xmanager Enterprise 2.0.0505 s/n: 060101-156821-000678 http://www.netsarang.com
NetSarang Xmanager SLS 2.0.0505 s/n: 060101-440211-000600 http://www.netsarang.com
NetSarang Xmanager Standard 2.0.0505 s/n: 060101-150801-000592 http://www.netsarang.com
NetSarang Xmanager Student 2-Year 2.0.0505 s/n: 060101-320481-000640 http://www.netsarang.com
NetSarang Xmanager Student 4-Year 2.0.0505 s/n: 060101-340301-000080 http://www.netsarang.com
NetSarang Xmanager Xftp Standard 2.0.0505 s/n: 060101-114011-000044 http://www.netsarang.com
NetSarang Xmanager Xlpd Standard 2.0.0505 s/n: 060101-112471-000482 http://www.netsarang.com
NetSarang Xmanager Xshell Educational 2.0.0505 s/n: 060101-121221-000953 http://www.netsarang.com
NetSarang Xmanager Xshell Standard 2.0.0505 s/n: 060101-151701-000609 http://www.netsarang.com
NetSarang Xmanager Xshell Student 2-Year 2.0.0505 s/n: 060101-321061-000073 http://www.netsarang.com
NetSarang Xmanager Xshell Student 4-Year 2.0.0505 s/n: 060101-341051-000641 http://www.netsarang.com
NetSuportmanager v9.10 S/N: Lisence: BIZIMKLUB.COM Serial:NSM980778 Max clients :25 Auth code : 8AF6BAB4 (null)
Network LookOut Administrator 1.83 Name: alkavour S/N: 7193F09790;EC;EF51214743A4 By Alkavour (15/01/2006) http://www.networklookout.com/
Network Monitor Professional v 3.7.11.20 S/N: 30075-25E-9BB92I2-848A6 SN: 30093-55I-9DB96F0-148F4 SN: 30057-58A-9BA98D9-278D1 By Alkavour (24/01/2006) http://downloads.numarasoftware.com/...7_Pro_Eval.exe
NewLive All Media Fixer Pro 5.2 S/N: 94935343-16637954-07732346-37221645-34234284-35950948-14C628362F9036174F672D12 By Alkavour (15/01/2006) http://www.realconvert.com/
NewsAloud 1.00 s/n: 921745+331181+9121234519156364 http://www.nextup.com
Nexus Mainframe Terminal 5.46 S/N: 3D3E-7393-F334-3036 sn:3B33-83B3-9339-353D sn:3E31-3353-3330-3F31 By Alkavour (24/01/2006) http://www.nexit.com/
NiftyNotes 1.0 Name: UnderPl Team s/n: 18578478 http://www.simplyawesomesoftware.com
Nizana dbWrench 1.0.6 s/n: 24868538 http://www.dbwrench.com
No1 Video Converter 4.0.1 Name: Team ZWT S/N: D0E78229-610D4894-393D7D61-B936B0AA-DC5544D4-22718CA3-38204A70-0D2F754C By Alkavour (24/01/2006) http://www.videotox.com/
NoClone 3.2.49 Name: FudoWarez S/N: C273FNL6 Name & Serial are CaSe sEnSiTiVe http://NoClone.net
Noiseware Professional for Adobe Photoshop 3.4.0.3 E-Mail: SSG@Team s/n: 34C64077D64B62A69BEB8674445EF0F9 http://www.imagenomic.com
nokia pc sait 017 S/N: 1244-4234-5467 (null)
Norman Virus Control German 5.80 R2 s/n: WY5ACATADRJQZ5WEJUSUSNT6E http://www.norman.com
Norman Virus Control Swedish 5.80 R2 s/n: CBBSCJZWB9RPX3MMQSYXIZVIE http://www.norman.com
Norman Virus Control 5.80 R2 s/n: 7QFSCQS5ERXPJ95YZTCN9YRFE http://www.norman.com
Norton 2006 2006 Name: Renjith Varghese S/N: VKFJ9-JVBJJ-VV426-RP29M-Y2YRK VKFJ9-JVBJJ-VV426-RP29M-Y2YRK by renjith varghese (null)
Norton 2006 7 S/N: VB96F FGRFP G8DFG YCJDX JMBBH (null)
Norton Anti virus 2006 1.0 Name: Renjith Varghese S/N: VKFJ9-JVBJJ-VV426-RP29M-Y2YRK by renjith varghese 100% working (null)
Norton Internet Security 2006 2006 S/N: JGG84-8YM4W-KPPCRQXRJJ-XBFFK (null)
Norton Personal Firewall 2005 8.0.2.5 S/N: 842442812 (null)
Norton System Works 2006 2006 Name: Norton System Works 2006 S/N: S/N: WK2WK-4T7B8-TPFGX-27JJC-6D8MX http://www.symantec.com/home_homeoff...nce/nswpr2006/
Norton Systemworks 2006 2006 S/N: WJF9K-WFRGJ-BJKJG-7VHYJ-HYMBQ (null)
Norton Systemworks Premier 2006 2006 S/N: WJF9K-WFRGJ-BJKJG-7VHYJ-HYMBQ (null)
Noteable 5.10 E-Mail: team@lz0.net s/n: 82310-29208-41613-75348 http://www.noteablesoftware.us
NotesXP 1.54 Name: Team Cafe s/n: 6921-0141 http://www.webhotel.lv/wiesturs/
Novation V-Station VSTi 1.4 s/n: 1014399654 http://www.novationmusic.com
Nurium BreakQuest 1.1.0 "s/n: UZ5FAN - 7BVUV1 - LPCTZK - EQ8H22 - TVE4Y3 - XPCBDS Note ! If you get access violation error , delete ""HKEY_CLASSES_ROOT\.mokt"" and reregister" http://www.nurium.com
_________________
Ilmu Akan Bermanfaat Bila Membiasakan Untuk Saling Berbagi.
O
O&O Defrag Personal 8.0.1398 S/N: Name: nn Firma: nn DPM9-0156-85A7-89AV-0DED (null)
O&O defrag pro v8 Name: freeserials.com S/N: DPM9-01HT-W5U7-89AL-0DET works (null)
Oceantiger jDeveloper 2.8 Name: BLZ-TEAM s/n: 82764-17458-27533-35508 oceantiger-software.com
OfficeIntercom 4.01 E-Mail: gibt@vonuns.net Name: TEAM ViRiLiTY s/n: 147852-hkvorz http://www.nch.com.au
Oggisoft EXE Blocker 2005.1.31 Name: TEAM TBE s/n: 11020464 http://www.oggisoft.de
Oggisoft Registerkarten Editor German 2005.10.88 Name: TEAM TBE s/n: 16447704 http://www.oggisoft.de
One Cat File Manager 2.02 Name: GeFcReW s/n: 1122334GSARPLN http://www.onecatweb.com
One Click CD DVD Writer 1.21 S/N: CDBURN-16BXUT-BBOGUT-193BFD-LL19Y2-UNLOCK SN: CDBURN-1D1FYW-5UD0K4-1AAOL7-QUFUYU-UNLOCK SN: CDBURN-1DEYBF-846UAW-15J15G-OP06BA-UNLOCK By Alkavour (05/01/2006) http://www.oneclicktools.com/cdwriter12.exe
One Click Ringtone Converter 1.1 S/N: 2LB2J2A4-N47F8D-1B8LIA-KOBLH4-MHA5CE-409A5A By Alkavour (24/01/2006) http://www.streamware-dev.com/
OO-Structure Maker for Delphi 5 1.0 Name: REVENGE CREW S/N: SEDHWSWIWHKJSEDJ By Alkavour (29/01/2006) http://www.dlldesigner.com
OO-Structure Maker for Delphi 6 1.0 Name: REVENGE CREW S/N: SEDHWSWIWHKJSEDJ By Alkavour (29/01/2006) http://www.dlldesigner.com
OO-Structure Maker for Delphi 7 1.0 Name: REVENGE CREW S/N: SEDHWSWIWHKJSEDJ By Alkavour (29/01/2006) http://www.dlldesigner.com
Opera 11000 Name: Nikola S/N: 1352005 ja sam ja http://www.opera.com
Opera 7.54u2 s/n: w-WMiJe-jYFuz-EW8Am-cD5SM-ipx6c http://www.opera.com
Opera 8.00 or later Name: SFdT WST S/N: s/n : w-sNRTB-kkYmU-J6Bta-45tJR-Sj4xz s/n : w-mMsDc-Unp3v-Wi4KE-ny4RF-D8Rha s/n : w-wtXFr-i5vXj-3TFvQ-ap58i-4rbkT Thank you fo choosing SFdT WST! go to official SFdT WST page at:search in google http://www.opera.com
Oplayo Media Designer 3.6.4 s/n: 3YR8424XSW20P3G0 http://www.oplayo.com
ORCA 13.0 S/N: Kundennr. 13899 Lizenztext: Fachhochschule Lippe und H??xter, 37671 H??xter Lizenznr.: OOM76 - Ue3qw - agO62 - o6Eu3 - agqL?? (null)
Ordix Mpack Professional 4.72 Name: BLZ-TEAM s/n: MPK472-C0087-8AD70-2060A http://www.ordix.com
Orphalese Tarot .NET 5.5.1 Name: king_KINK s/n1: 894438 s/n2: 2127224369780496 http://www.orphalese.net
OSL2000 Boot Manager 8.80 s/n: BHB-HAI-PFOA http://www.osloader.com
OTo Protect Photos 1.5 s/n: AWOEUTM9OR45EI http://www.quotevision.net
Outlook Express Backup V6.5 V6.5 Name: Aline S/N: OEB-363F86A0A2008AE7 http://www.genie-soft.com/
Outlook Picture Extractor 1.33 Name: TEAM ACME s/n: 78409818 http://www.ope2000.com
P
Panorado 3.3.1.53 Name: email:heretic@hush.ai S/N: 569f495e-02050000-0213a3b4-d1cd4f97 By Alkavour (14/01/2006) http://www.panorado.com
Partition Magic Pro 8.0 S/N: PM80PM800ENSP1-11141269 0ENSP1-11111131 (null)
Password Door 7.0.2 s/n: 83524-86774-82745-92071 http://www.toplang.com
PC 2 Answering Machine Pro Edition 2.0.8.4 s/n: oeiu-564-oqei-97 http://www.teley.com
PC Booster 3 S/N: 34623-igcd-97387 (null)
PC SYNC PRO 5.0 S/N: Z6SN-9NXE-EG7A-XQ86 (null)
PC Weather Machine 1.2.3 Name: TEAM TBE s/n: IJDP-7121-2195-7000 http://www.pcwm.com.ar
PC-Adresszz Enterprise 6.06 Name: TEAM ViRiLiTY s/n: V6E-6R74TR-2X5QW9-4T63V7-WM7G5U http://www.hastasoft.de
PC-Adresszz Professional 6.06 Name: TEAM ViRiLiTY s/n: V66-D36QBS-77P7RT-7757RT-77P73T http://www.hastasoft.de
PC-Network Remote Server 2.1 Name: d/tmg s/n: Vd-195728 http://www.hastasoft.de
PDACookbook Plus 3.7.6 Name: AGAiN tEAM s/n: 13123 http://www.wakefieldsoft.com
PDF Generator 1.48 Name: Team F4CG s/n: D3810ACC26A0EEA0B766EE0750FFADA0 http://www.alientools.com
PDF U Append Desktop Edition 1.08 s/n: IJ110H93016M4435J75KH4JM2IJ8JJ29KIJ82HJKJL2MH1I5 http://www.traction-software.co.uk
PDF2HTML 1.6 E-Mail: anything s/n: 15$/Q892X2OD6N59 http://www.verypdf.com
pdfFactory Pro Enterprise 2.35 Name: EMBRACE s/n: EYE8-85VL-EKR2 http://www.pdffactory.com
Pdffactory Pro Server Edition 2.50 S/N: CD62M-QY77D-DLDZ6 sn:VZ7C4-W6DM2-JZQE6 sn:P7AR7-TPDM2-X72D6 By Alkavour (12/01/2006) http://www.fineprint.com/
Pentom AgentOrganizer 2.7.82 s/n: 1697-2994-7022-1844 http://www.pentomtechnologies.com
Pentom AgentOrganizer 2.8.29 s/n: 1697-2950-2022-1364 http://www.pentomtechnologies.com
PercussionStudio 3.1b Name: The Millenium Group s/n: JTJXMEHGCG http://www.henrykellner.com
Perfect Keylogger 1.62.DC10262005 Name: CRUDE s/n: DHRU-JGKA-FGNJ-HNFE http://www.blazingtools.com
Persits AspJpeg 1.5.0.0 Name: Hambo s/n: 63294-52920-94153 http://www.persits.com
Personal Inspector 4.14 Name: Team EXPLOSiON E-Mail: Team EXPLOSiON s/n: R1XU7DXG21I182HMXIMIX5DFK http://www.personal-inspector.com
Personal Inspector 4.22 Name: Team EXPLOSiON E-Mail: Team EXPLOSiON s/n: 9DBBVXXJJAXB12XBZ1FI7DDYR http://www.personal-inspector.com
Personal Mailing List 1.2k Name: TEAM ACME s/n: RKS-3612184 http://www.rkssoftware.com
Personalzeit Manager 1.30.3.15 Name: Team ACME s/n1: PM128528 s/n2: 70387245 http://www.zwahlen-informatik.ch
PestPatrol 4 S/N: 08116751427 (null)
Phoenix Backup Professional 2.0.0.4610 s/n: J3F0B-87K9G-A97HF-A9XH4-BF696 http://www.s-a-d.de
Phone Calls Filter 1.0.2.0 s/n: asoip_35_sldk_413 http://www.teley.com
Phone Recorder Plus 1.0.3.0 s/n: exr-987-mvp-im10 http://www.teley.com
Photo Screensaver Maker 5.0 Name: BRD Cult S/N: 0ED63A40-7E7CCE93-95F6F221-1A7D1286-077FCEFC-306B992A-73E4D82D-3FC7898E By Alkavour (14/01/2006) http://www.aone-soft.com
Photo Vector 1.98.5 Name: TEAM HERETiC S/N: email:heretic@inter.net serial:011MU2MASPR7GM4TC0B By Alkavour (14/01/2006) http://www.algolab.com/Photovector.htm
PhotoFiltre Studio 7.3 Name: KaiZer SoZe S/N: 74B57-D5274-884E7-DB17B By Alkavour (24/01/2006) http://www.photofiltre.com/
PhotoFiltre 6.1 Name: KaiZer SoZe s/n: 1EFAC-F0B8D-B47C8-EB47C http://www.photofiltre.com
PhotoImpact 11 S/N: 111A3-0B000-01054510 Great Software for Photo and Graphic Editing and Creation http://www.ulead.com
PhotoToFilm 1.4.1.44 E-Mail: TEAM ViRiLiTY s/n: 91091090073078032107081083080097081085096 http://www.kcsoftwares.com
PhotoToFilm 1.4.2.45 Name: CRUDE s/n: 21074103093069076 http://www.kcsoftwares.com
PhotoToFilm 2.2.0.48 Name: TEAM ViRiLiTY s/n: 91105080082086032101088086074090085089088 http://www.kcsoftwares.com
Picture Ace 2.5.9 s/n: 3BF9-C8E2-8F57-F291-242F-BCFD-0965-AF98-82E1-2D75 http://www.pictureace.com
Picture Suite 1.9.1.45 Name: ReD0c/GEAR S/N: 3009-2963-6114-GEAR http://www.ck-software.de
Pigna 1.60 s/n: ZWVDFRPYLCX http://www.trillium-production.de
Pigna 1.70 s/n: ZWVDFRPYLCX http://www.trillium-production.de
Pindersoft ASL Logbook 1.0.1788 s/n: 063063A2-2E05-4250-9639-57BCE8192C2B http://www.pindersoft.com
Pindersoft CardFile PS.Net 2.1.1800.19594 s/n: E090DA94-B9C5-42e5-B09E-2792580C207F http://www.pindersoft.com
Pindersoft Source Code Organizer PS 2.0 s/n: 6FAA4915-8231-4575-8EE0-CBD429C1171F http://www.pindersoft.com
Pindersoft Web Resources 2.5 s/n: 8F4F111F-1F05-4555-9D25-902837785140 http://www.pindersoft.com
Pink Calendar and Day Planner 5.0 s/n: 14921776 http://www.orangesoftware.net
Pintexx pinTab Net 2.0 s/n: 5B4E4D3D3A3C3D3F http://www.pintexx.com
Planet Earth 3D Screensaver 1.0 S/N: Name : (Your name) Code : IoN8hhTlp3hwvnPW http://www.digimindsoft.com
Planet Jupiter 3D Screensaver 1.0 S/N: Name : (Your name) Code : 1fFrWIdHktCeHuIy (null)
Planet Mars 3D Screensaver 1.0 S/N: Name : (Your name) Code : C931ruEUzelsNCnk http://www.space-screensavers.com
Planet Mercury 3D Screensaver 1.0 S/N: Name : (Your name) Code : ojiumuTV0TqoLZTk http://www.space-screensavers.com
Planet Neptune 3D Screensaver 1.0 S/N: Name : (Your name) Code : eP5A44B8fBzNctqa http://www.space-screensavers.com
Planet Pluto 3D Screensaver 1.0 S/N: Name : (Your name) Code : Ksn9vgGZo1j92CTY or 50CUCCH8N2FRNccJ http://www.space-screensavers.com
Planet Saturn 3D Screensaver 1.0 S/N: Name : (Your name) Code : FIuMphJmE2wodEI5 http://www.space-screensavers.com
Planet Uranus 3D Screensaver 1.0 S/N: Name: (Your name) Serial: G8mnGVNqKNR86B86 http://www.space-screensavers.com
Planet Venus 3D Screensaver 1.0 S/N: Name : (Your name) Code : kZ2voxY5QCt3sSAy http://www.space-screensavers.com
PlanMaker 2004 R279b Name: AGAiN tEAM E-Mail: AGAiN tEAM s/n1: 74760329107-PH-FT s/n2: 2CT3D-L7HXJ-BY5U4-9DFF0-Z9031 http://www.softmaker.de
Plato DVD Ripper 1.16 s/n: 5350-21800-9094-7356 http://www.dvdtomp3.net
Plato DVD Ripper 1.17 s/n: 5716S17153V572085717 http://www.dvdtomp3.net
Plato DVD Ripper 2.33 S/N: 751387C0F6B0DED2492CE9C1148E9C351BA99E7C4C50695038 5A8CA4E836FA283CC26EEB7EA7796A6D75B1BC55B11F8B sn:60136E2661CE5DBC6B5AB48FE8FD31B10DC88DA68773E78 6B77DA877CD11BF185D5372D0E9EC70C0C5E9D4AB1515A07F sn:6BC9C85E38957131098C984ED0AF59690BA011443EB4EE6 09124E By Alkavour (23/01/2006) http://www.dvdtomp3.net
What’s New in the Auto Run Design 3.0.0.16 serial key or number?
Screen Shot
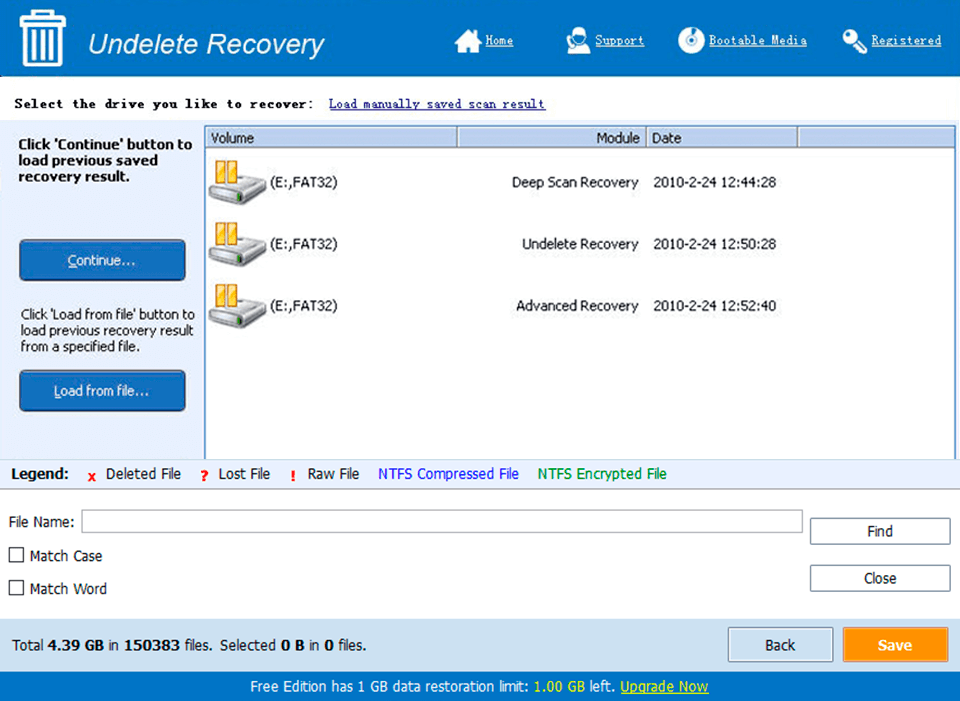
System Requirements for Auto Run Design 3.0.0.16 serial key or number
- First, download the Auto Run Design 3.0.0.16 serial key or number
-
You can download its setup from given links: