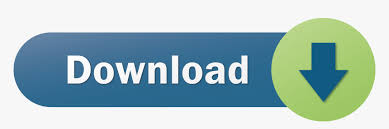
Tw keygen
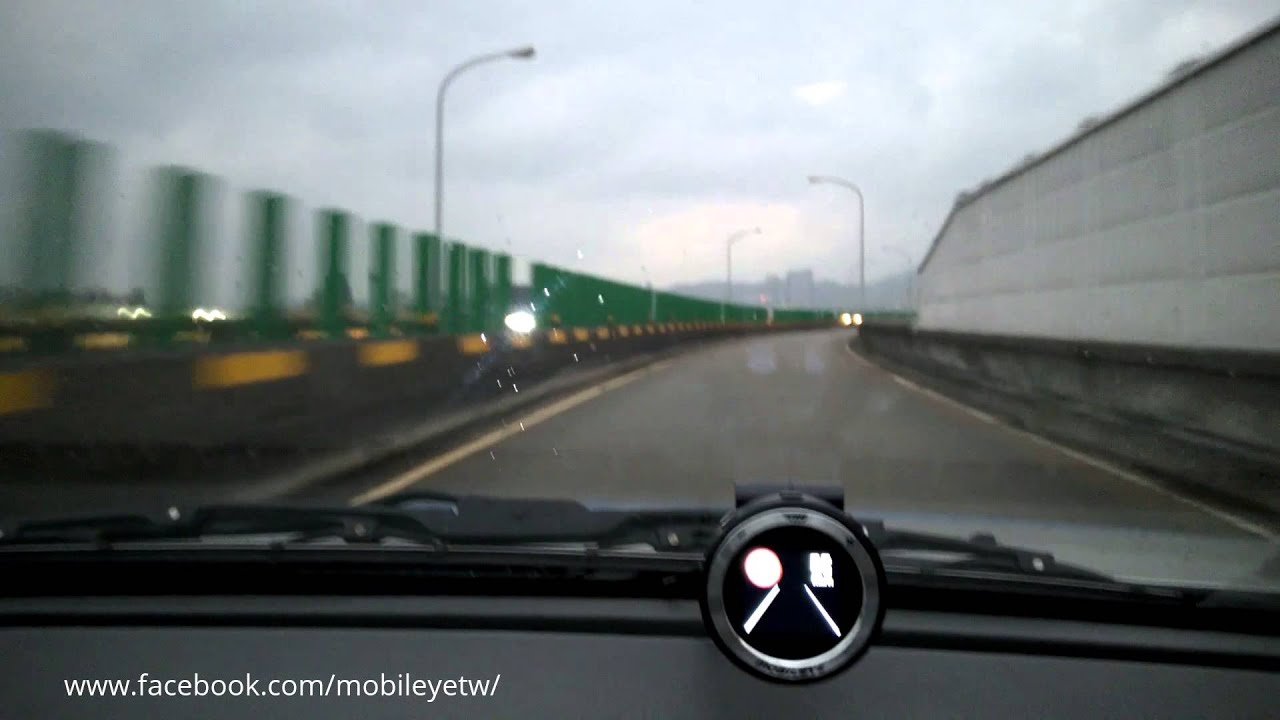
Tw keygen
Falcon source files (reference implementation)
keygen.c
1 /* 2 * Falcon key pair generation. 3 * 4 * ==========================(LICENSE BEGIN)============================ 5 * 6 * Copyright (c) 2017-2019 Falcon Project 7 * 8 * Permission is hereby granted, free of charge, to any person obtaining 9 * a copy of this software and associated documentation files (the 10 * "Software"), to deal in the Software without restriction, including 11 * without limitation the rights to use, copy, modify, merge, publish, 12 * distribute, sublicense, and/or sell copies of the Software, and to 13 * permit persons to whom the Software is furnished to do so, subject to 14 * the following conditions: 15 * 16 * The above copyright notice and this permission notice shall be 17 * included in all copies or substantial portions of the Software. 18 * 19 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 20 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 21 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 22 * IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY 23 * CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, 24 * TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE 25 * SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 26 * 27 * ===========================(LICENSE END)============================= 28 * 29 * @author Thomas Pornin <thomas.pornin@nccgroup.com> 30 */ 31 32 #include"inner.h" 33 34 #define MKN(logn) ((size_t)1 << (logn)) 35 36 /* ==================================================================== */ 37 /* 38 * Modular arithmetics. 39 * 40 * We implement a few functions for computing modulo a small integer p. 41 * 42 * All functions require that 2^30 < p < 2^31. Moreover, operands must 43 * be in the 0..p-1 range. 44 * 45 * Modular addition and subtraction work for all such p. 46 * 47 * Montgomery multiplication requires that p is odd, and must be provided 48 * with an additional value p0i = -1/p mod 2^31. See below for some basics 49 * on Montgomery multiplication. 50 * 51 * Division computes an inverse modulo p by an exponentiation (with 52 * exponent p-2): this works only if p is prime. Multiplication 53 * requirements also apply, i.e. p must be odd and p0i must be provided. 54 * 55 * The NTT and inverse NTT need all of the above, and also that 56 * p = 1 mod 2048. 57 * 58 * ----------------------------------------------------------------------- 59 * 60 * We use Montgomery representation with 31-bit values: 61 * 62 * Let R = 2^31 mod p. When 2^30 < p < 2^31, R = 2^31 - p. 63 * Montgomery representation of an integer x modulo p is x*R mod p. 64 * 65 * Montgomery multiplication computes (x*y)/R mod p for 66 * operands x and y. Therefore: 67 * 68 * - if operands are x*R and y*R (Montgomery representations of x and 69 * y), then Montgomery multiplication computes (x*R*y*R)/R = (x*y)*R 70 * mod p, which is the Montgomery representation of the product x*y; 71 * 72 * - if operands are x*R and y (or x and y*R), then Montgomery 73 * multiplication returns x*y mod p: mixed-representation 74 * multiplications yield results in normal representation. 75 * 76 * To convert to Montgomery representation, we multiply by R, which is done 77 * by Montgomery-multiplying by R^2. Stand-alone conversion back from 78 * Montgomery representation is Montgomery-multiplication by 1. 79 */ 80 81 /* 82 * Precomputed small primes. Each element contains the following: 83 * 84 * p The prime itself. 85 * 86 * g A primitive root of phi = X^N+1 (in field Z_p). 87 * 88 * s The inverse of the product of all previous primes in the array, 89 * computed modulo p and in Montgomery representation. 90 * 91 * All primes are such that p = 1 mod 2048, and are lower than 2^31. They 92 * are listed in decreasing order. 93 */ 94 95 typedefstruct{ 96 uint32_t p; 97 uint32_t g; 98 uint32_t s; 99 } small_prime; 100 101 static const small_prime PRIMES[] = { 102 {2147473409,383167813,10239}, 103 {2147389441,211808905,471403745}, 104 {2147387393,37672282,1329335065}, 105 {2147377153,1977035326,968223422}, 106 {2147358721,1067163706,132460015}, 107 {2147352577,1606082042,598693809}, 108 {2147346433,2033915641,1056257184}, 109 {2147338241,1653770625,421286710}, 110 {2147309569,631200819,1111201074}, 111 {2147297281,2038364663,1042003613}, 112 {2147295233,1962540515,19440033}, 113 {2147239937,2100082663,353296760}, 114 {2147235841,1991153006,1703918027}, 115 {2147217409,516405114,1258919613}, 116 {2147205121,409347988,1089726929}, 117 {2147196929,927788991,1946238668}, 118 {2147178497,1136922411,1347028164}, 119 {2147100673,868626236,701164723}, 120 {2147082241,1897279176,617820870}, 121 {2147074049,1888819123,158382189}, 122 {2147051521,25006327,522758543}, 123 {2147043329,327546255,37227845}, 124 {2147039233,766324424,1133356428}, 125 {2146988033,1862817362,73861329}, 126 {2146963457,404622040,653019435}, 127 {2146959361,1936581214,995143093}, 128 {2146938881,1559770096,634921513}, 129 {2146908161,422623708,1985060172}, 130 {2146885633,1751189170,298238186}, 131 {2146871297,578919515,291810829}, 132 {2146846721,1114060353,915902322}, 133 {2146834433,2069565474,47859524}, 134 {2146818049,1552824584,646281055}, 135 {2146775041,1906267847,1597832891}, 136 {2146756609,1847414714,1228090888}, 137 {2146744321,1818792070,1176377637}, 138 {2146738177,1118066398,1054971214}, 139 {2146736129,52057278,933422153}, 140 {2146713601,592259376,1406621510}, 141 {2146695169,263161877,1514178701}, 142 {2146656257,685363115,384505091}, 143 {2146650113,927727032,537575289}, 144 {2146646017,52575506,1799464037}, 145 {2146643969,1276803876,1348954416}, 146 {2146603009,814028633,1521547704}, 147 {2146572289,1846678872,1310832121}, 148 {2146547713,919368090,1019041349}, 149 {2146508801,671847612,38582496}, 150 {2146492417,283911680,532424562}, 151 {2146490369,1780044827,896447978}, 152 {2146459649,327980850,1327906900}, 153 {2146447361,1310561493,958645253}, 154 {2146441217,412148926,287271128}, 155 {2146437121,293186449,2009822534}, 156 {2146430977,179034356,1359155584}, 157 {2146418689,1517345488,1790248672}, 158 {2146406401,1615820390,1584833571}, 159 {2146404353,826651445,607120498}, 160 {2146379777,3816988,1897049071}, 161 {2146363393,1221409784,1986921567}, 162 {2146355201,1388081168,849968120}, 163 {2146336769,1803473237,1655544036}, 164 {2146312193,1023484977,273671831}, 165 {2146293761,1074591448,467406983}, 166 {2146283521,831604668,1523950494}, 167 {2146203649,712865423,1170834574}, 168 {2146154497,1764991362,1064856763}, 169 {2146142209,627386213,1406840151}, 170 {2146127873,1638674429,2088393537}, 171 {2146099201,1516001018,690673370}, 172 {2146093057,1294931393,315136610}, 173 {2146091009,1942399533,973539425}, 174 {2146078721,1843461814,2132275436}, 175 {2146060289,1098740778,360423481}, 176 {2146048001,1617213232,1951981294}, 177 {2146041857,1805783169,2075683489}, 178 {2146019329,272027909,1753219918}, 179 {2145986561,1206530344,2034028118}, 180 {2145976321,1243769360,1173377644}, 181 {2145964033,887200839,1281344586}, 182 {2145906689,1651026455,906178216}, 183 {2145875969,1673238256,1043521212}, 184 {2145871873,1226591210,1399796492}, 185 {2145841153,1465353397,1324527802}, 186 {2145832961,1150638905,554084759}, 187 {2145816577,221601706,427340863}, 188 {2145785857,608896761,316590738}, 189 {2145755137,1712054942,1684294304}, 190 {2145742849,1302302867,724873116}, 191 {2145728513,516717693,431671476}, 192 {2145699841,524575579,1619722537}, 193 {2145691649,1925625239,982974435}, 194 {2145687553,463795662,1293154300}, 195 {2145673217,771716636,881778029}, 196 {2145630209,1509556977,837364988}, 197 {2145595393,229091856,851648427}, 198 {2145587201,1796903241,635342424}, 199 {2145525761,715310882,1677228081}, 200 {2145495041,1040930522,200685896}, 201 {2145466369,949804237,1809146322}, 202 {2145445889,1673903706,95316881}, 203 {2145390593,806941852,1428671135}, 204 {2145372161,1402525292,159350694}, 205 {2145361921,2124760298,1589134749}, 206 {2145359873,1217503067,1561543010}, 207 {2145355777,338341402,83865711}, 208 {2145343489,1381532164,641430002}, 209 {2145325057,1883895478,1528469895}, 210 {2145318913,1335370424,65809740}, 211 {2145312769,2000008042,1919775760}, 212 {2145300481,961450962,1229540578}, 213 {2145282049,910466767,1964062701}, 214 {2145232897,816527501,450152063}, 215 {2145218561,1435128058,1794509700}, 216 {2145187841,33505311,1272467582}, 217 {2145181697,269767433,1380363849}, 218 {2145175553,56386299,1316870546}, 219 {2145079297,2106880293,1391797340}, 220 {2145021953,1347906152,720510798}, 221 {2145015809,206769262,1651459955}, 222 {2145003521,1885513236,1393381284}, 223 {2144960513,1810381315,31937275}, 224 {2144944129,1306487838,2019419520}, 225 {2144935937,37304730,1841489054}, 226 {2144894977,1601434616,157985831}, 227 {2144888833,98749330,2128592228}, 228 {2144880641,1772327002,2076128344}, 229 {2144864257,1404514762,2029969964}, 230 {2144827393,801236594,406627220}, 231 {2144806913,349217443,1501080290}, 232 {2144796673,1542656776,2084736519}, 233 {2144778241,1210734884,1746416203}, 234 {2144759809,1146598851,716464489}, 235 {2144757761,286328400,1823728177}, 236 {2144729089,1347555695,1836644881}, 237 {2144727041,1795703790,520296412}, 238 {2144696321,1302475157,852964281}, 239 {2144667649,1075877614,504992927}, 240 {2144573441,198765808,1617144982}, 241 {2144555009,321528767,155821259}, 242 {2144550913,814139516,1819937644}, 243 {2144536577,571143206,962942255}, 244 {2144524289,1746733766,2471321}, 245 {2144512001,1821415077,124190939}, 246 {2144468993,917871546,1260072806}, 247 {2144458753,378417981,1569240563}, 248 {2144421889,175229668,1825620763}, 249 {2144409601,1699216963,351648117}, 250 {2144370689,1071885991,958186029}, 251 {2144348161,1763151227,540353574}, 252 {2144335873,1060214804,919598847}, 253 {2144329729,663515846,1448552668}, 254 {2144327681,1057776305,590222840}, 255 {2144309249,1705149168,1459294624}, 256 {2144296961,325823721,1649016934}, 257 {2144290817,738775789,447427206}, 258 {2144243713,962347618,893050215}, 259 {2144237569,1655257077,900860862}, 260 {2144161793,242206694,1567868672}, 261 {2144155649,769415308,1247993134}, 262 {2144137217,320492023,515841070}, 263 {2144120833,1639388522,770877302}, 264 {2144071681,1761785233,964296120}, 265 {2144065537,419817825,204564472}, 266 {2144028673,666050597,2091019760}, 267 {2144010241,1413657615,1518702610}, 268 {2143952897,1238327946,475672271}, 269 {2143940609,307063413,1176750846}, 270 {2143918081,2062905559,786785803}, 271 {2143899649,1338112849,1562292083}, 272 {2143891457,68149545,87166451}, 273 {2143885313,921750778,394460854}, 274 {2143854593,719766593,133877196}, 275 {2143836161,1149399850,1861591875}, 276 {2143762433,1848739366,1335934145}, 277 {2143756289,1326674710,102999236}, 278 {2143713281,808061791,1156900308}, 279 {2143690753,388399459,1926468019}, 280 {2143670273,1427891374,1756689401}, 281 {2143666177,1912173949,986629565}, 282 {2143645697,2041160111,371842865}, 283 {2143641601,1279906897,2023974350}, 284 {2143635457,720473174,1389027526}, 285 {2143621121,1298309455,1732632006}, 286 {2143598593,1548762216,1825417506}, 287 {2143567873,620475784,1073787233}, 288 {2143561729,1932954575,949167309}, 289 {2143553537,354315656,1652037534}, 290 {2143541249,577424288,1097027618}, 291 {2143531009,357862822,478640055}, 292 {2143522817,2017706025,1550531668}, 293 {2143506433,2078127419,1824320165}, 294 {2143488001,613475285,1604011510}, 295 {2143469569,1466594987,502095196}, 296 {2143426561,1115430331,1044637111}, 297 {2143383553,9778045,1902463734}, 298 {2143377409,1557401276,2056861771}, 299 {2143363073,652036455,1965915971}, 300 {2143260673,1464581171,1523257541}, 301 {2143246337,1876119649,764541916}, 302 {2143209473,1614992673,1920672844}, 303 {2143203329,981052047,2049774209}, 304 {2143160321,1847355533,728535665}, 305 {2143129601,965558457,603052992}, 306 {2143123457,2140817191,8348679}, 307 {2143100929,1547263683,694209023}, 308 {2143092737,643459066,1979934533}, 309 {2143082497,188603778,2026175670}, 310 {2143062017,1657329695,377451099}, 311 {2143051777,114967950,979255473}, 312 {2143025153,1698431342,1449196896}, 313 {2143006721,1862741675,1739650365}, 314 {2142996481,756660457,996160050}, 315 {2142976001,927864010,1166847574}, 316 {2142965761,905070557,661974566}, 317 {2142916609,40932754,1787161127}, 318 {2142892033,1987985648,675335382}, 319 {2142885889,797497211,1323096997}, 320 {2142871553,2068025830,1411877159}, 321 {2142861313,1217177090,1438410687}, 322 {2142830593,409906375,1767860634}, 323 {2142803969,1197788993,359782919}, 324 {2142785537,643817365,513932862}, 325 {2142779393,1717046338,218943121}, 326 {2142724097,89336830,416687049}, 327 {2142707713,5944581,1356813523}, 328 {2142658561,887942135,2074011722}, 329 {2142638081,151851972,1647339939}, 330 {2142564353,1691505537,1483107336}, 331 {2142533633,1989920200,1135938817}, 332 {2142529537,959263126,1531961857}, 333 {2142527489,453251129,1725566162}, 334 {2142502913,1536028102,182053257}, 335 {2142498817,570138730,701443447}, 336 {2142416897,326965800,411931819}, 337 {2142363649,1675665410,1517191733}, 338 {2142351361,968529566,1575712703}, 339 {2142330881,1384953238,1769087884}, 340 {2142314497,1977173242,1833745524}, 341 {2142289921,95082313,1714775493}, 342 {2142283777,109377615,1070584533}, 343 {2142277633,16960510,702157145}, 344 {2142263297,553850819,431364395}, 345 {2142208001,241466367,2053967982}, 346 {2142164993,1795661326,1031836848}, 347 {2142097409,1212530046,712772031}, 348 {2142087169,1763869720,822276067}, 349 {2142078977,644065713,1765268066}, 350 {2142074881,112671944,643204925}, 351 {2142044161,1387785471,1297890174}, 352 {2142025729,783885537,1000425730}, 353 {2142011393,905662232,1679401033}, 354 {2141974529,799788433,468119557}, 355 {2141943809,1932544124,449305555}, 356 {2141933569,1527403256,841867925}, 357 {2141931521,1247076451,743823916}, 358 {2141902849,1199660531,401687910}, 359 {2141890561,150132350,1720336972}, 360 {2141857793,1287438162,663880489}, 361 {2141833217,618017731,1819208266}, 362 {2141820929,999578638,1403090096}, 363 {2141786113,81834325,1523542501}, 364 {2141771777,120001928,463556492}, 365 {2141759489,122455485,2124928282}, 366 {2141749249,141986041,940339153}, 367 {2141685761,889088734,477141499}, 368 {2141673473,324212681,1122558298}, 369 {2141669377,1175806187,1373818177}, 370 {2141655041,1113654822,296887082}, 371 {2141587457,991103258,1585913875}, 372 {2141583361,1401451409,1802457360}, 373 {2141575169,1571977166,712760980}, 374 {2141546497,1107849376,1250270109}, 375 {2141515777,196544219,356001130}, 376 {2141495297,1733571506,1060744866}, 377 {2141483009,321552363,1168297026}, 378 {2141458433,505818251,733225819}, 379 {2141360129,1026840098,948342276}, 380 {2141325313,945133744,2129965998}, 381 {2141317121,1871100260,1843844634}, 382 {2141286401,1790639498,1750465696}, 383 {2141267969,1376858592,186160720}, 384 {2141255681,2129698296,1876677959}, 385 {2141243393,2138900688,1340009628}, 386 {2141214721,1933049835,1087819477}, 387 {2141212673,1898664939,1786328049}, 388 {2141202433,990234828,940682169}, 389 {2141175809,1406392421,993089586}, 390 {2141165569,1263518371,289019479}, 391 {2141073409,1485624211,507864514}, 392 {2141052929,1885134788,311252465}, 393 {2141040641,1285021247,280941862}, 394 {2141028353,1527610374,375035110}, 395 {2141011969,1400626168,164696620}, 396 {2140999681,632959608,966175067}, 397 {2140997633,2045628978,1290889438}, 398 {2140993537,1412755491,375366253}, 399 {2140942337,719477232,785367828}, 400 {2140925953,45224252,836552317}, 401 {2140917761,1157376588,1001839569}, 402 {2140887041,278480752,2098732796}, 403 {2140837889,1663139953,924094810}, 404 {2140788737,802501511,2045368990}, 405 {2140766209,1820083885,1800295504}, 406 {2140764161,1169561905,2106792035}, 407 {2140696577,127781498,1885987531}, 408 {2140684289,16014477,1098116827}, 409 {2140653569,665960598,1796728247}, 410 {2140594177,1043085491,377310938}, 411 {2140579841,1732838211,1504505945}, 412 {2140569601,302071939,358291016}, 413 {2140567553,192393733,1909137143},HTML DOM Keygen Object
Keygen Object
The Keygen object represents an HTML <keygen> element.
Note: The <keygen> element is not supported in Internet Explorer / Edge.
Access a Keygen Object
You can access a <keygen> element by using getElementById():
Create a Keygen Object
You can create a <keygen> element by using the document.createElement() method:
Keygen Object Properties
Property | Description |
---|---|
autofocus | Sets or returns whether a keygen field automatically gets focus when the page loads, or not |
challenge | Sets or returns the value of the challenge attribute of a keygen field |
disabled | Sets or returns whether a keygen field is disabled, or not |
form | Returns a reference to the form that contains the keygen field |
keytype | Sets or returns the value of the keytype attribute of a keygen field |
name | Sets or returns the value of the name attribute of a keygen field |
type | Returns which type of form element the keygen field is |
Standard Properties and Events
The Keygen object also supports the standard properties and events.
Related Pages
HTML tutorial: HTML Forms
HTML reference: HTML <keygen> tag
MS Office 2007 Language Pack - Traditional Chinese (zh-tw) Keygen
MS Office 2007 Language Pack - Traditional Chinese (zh-tw) Keygen
Language packs add additional display, help, and proofing tools to Office. You can install additional language accessory packs after installing Microsoft Office.. Listen to MS Office 2007 Language Pack - Traditional Chinese (zh-tw) Keygen and forty-eight more episodes by War Child: A Child Soldier's.... Method 4: Microsoft Office 2000 MultiLanguage Pack ... Install the Traditional Chinese Language Pack is the best and easiest way to view.... MS Office 2007 Language Pack - Traditional Chinese (Zh-Tw) ... Service Pack 3 Microsoft Office 2007 .2007 ... R2R [deepstatus],,,Windows XP Pro - Working KeyGen and Serial Number List,,,.... DVD Chinese Taiwan6. SP1 twwindows7enterprisewithsp. OCAT.jpg' alt='Ms Office 2007 Language Pack - Traditional Chinese (Zh-Tw)'.... Microsoft Office IME 2010 is offered to Office 2013 users on Windows 7 or Windows 2008 Server R2. This is also offered for free to the users who have the Office license. ... Selecting a language below will dynamically change the complete ... Chinese (Simplified), Chinese (Traditional), English, Japanese.... Where to get Office language packs and proofing tools for Chinese language, ... Simplified Chinese by number of strokes or Pinyin, and Traditional Chinese ... "Proofing Tools", in the full 36-language Office Multi-Language Pack 2007 new site.... Find out how to download and install additional language packs for your version of ... To use Microsoft Office in your preferred language, download an Office language pack. ... Chinese Traditional, (), Any language.. MS Office 2007 Language Pack - Traditional Chinese (zh-tw) Keygen >>> http://bytlly.com/182xuq 4c1e08f8e7.... goto the Taiwan section and download all three zh-tw files ... I got the same problem with my Microsoft Office 2007 English / Traditional ... Chinese language packs, only Excel got a tab on Chinese translation in the. Download Traditional Chinese (zh-TW) Language Pack for Firefox. Traditional Chinese (zh-TW) Language Pack.. Ms Office 2007 Language Pack - Traditional Chinese (Zh-Tw) Get help, support, and tutorials for Windows productsWindows 10, Windows 8.. ... Pro 6.05 latest1 updated crack MS Office 2007 Language Pack - Traditional Chinese (zh-tw) Serial Key keygen MICROSOFT OFFICE WORD...
c7eb224936
descargar cantinflas el portero dvdrip 16
MS Office 2016 Pro Plus VL X64 MULTi-22 JUNE 2018 {Gen2} download pc
Powershell stuff.rar .rar
Laawaris movie download in hindi 720p download
addictive drums 2 keygen torrent
Serial for nvidia 3dtv play unlock 38
css slider 2.1 registration key crackgolkes
Malwarebytes Premium 3.0 FINAL Crack [TechTools] keygen
Bbc literary companion class 9 pdf
ArcSoft Portrait Plus 3.0.0.400 [Patch MPT] [Photoshop Plugin] full version
What’s New in the Tw keygen?
Screen Shot
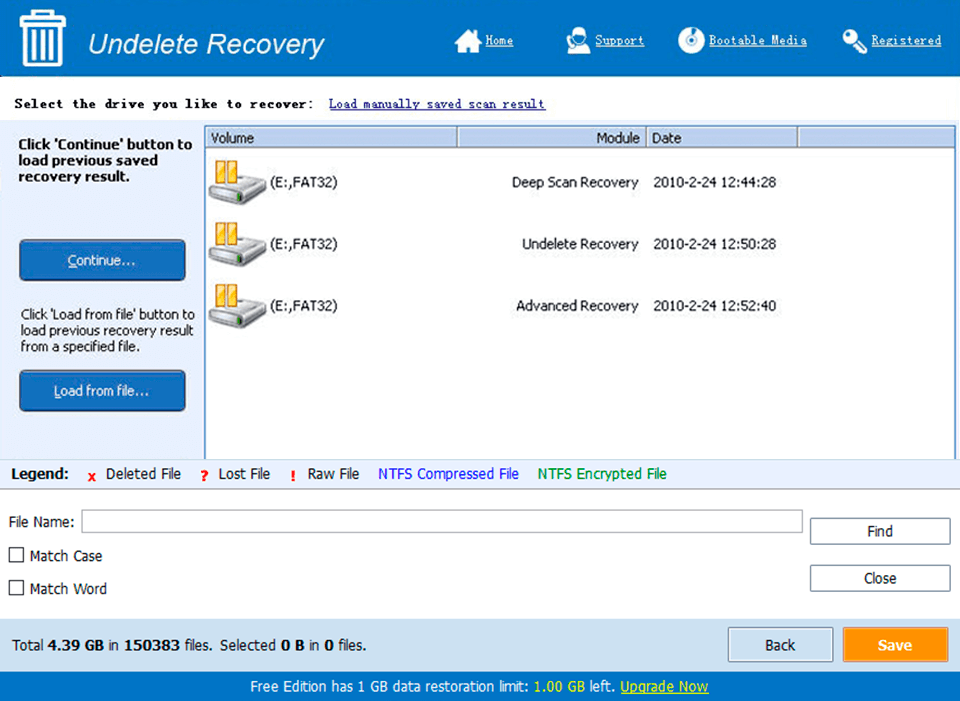
System Requirements for Tw keygen
- First, download the Tw keygen
-
You can download its setup from given links: